filmov
tv
Arrays in Java (Exercise 4)
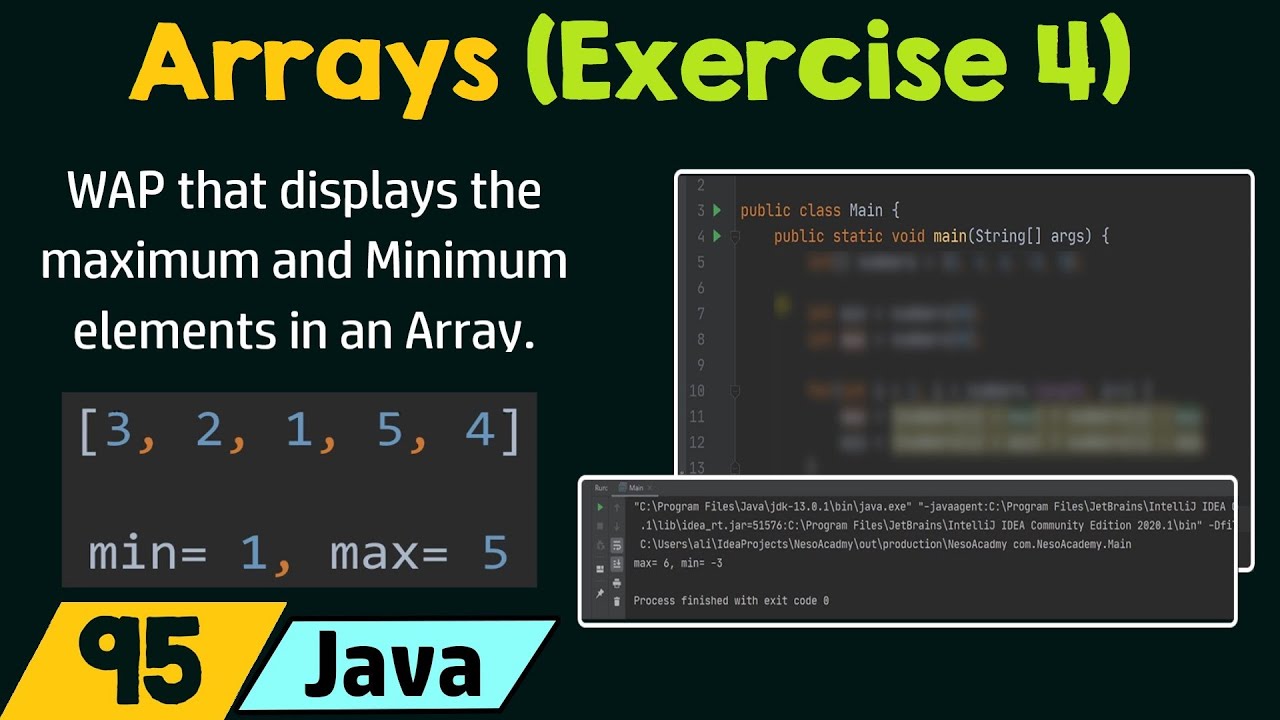
Показать описание
Java Programming: Programming Question on Arrays in Java Programming
Topics Discussed:
1. Writing a program that displays the maximum and minimum elements of an array.
Music:
Axol x Alex Skrindo - You [NCS Release]
#JavaByNeso #JavaProgramming #ArraysInJava
Topics Discussed:
1. Writing a program that displays the maximum and minimum elements of an array.
Music:
Axol x Alex Skrindo - You [NCS Release]
#JavaByNeso #JavaProgramming #ArraysInJava
Arrays in Java (Exercise 4)
Programming 1 Java - 5.6 Array Exercise 4
Learn Java - Exercise 04x - 2D Arrays in Java
w3resource.com: Java Array Exercise-4
Java coding exercises for beginners - Arrays
How To Get Array Input From A User In Java Using Scanner
Loops in Java (Exercise 4)
Java Tutorial For Beginners - Practice questions on Java Array
Array vs. ArrayList in Java Tutorial - What's The Difference?
Java Tutorial for Beginners #8 - Arrays
Learn Java - Exercise 14x - Using Arrays of Strings in Java
Java 2D arrays 🚚
Java Tutorial - 02 - Using a Loop to Access an Array
Java #4 - 2D Arrays and Exercises
❤❤❤ Make a full heart java code ❤❤💻💻💻📒📒💻😘🤗❤❤
Learn Java - Exercise 05x - Array Length Instance Variable
Java Tutorial - 06 - Using Enhanced For Loop with Arrays
Array of Objects Java Tutorial #73
❌ DON'T use a for loop like this for multiple Lists in Python!!!
Learn Java - Exercise 02x - Java Arrays and Loops
Learn Java for Beginners - 29 - Array Exercise
#Java introduction to arrays - Easy-to-follow Java programming for beginners
Learn Java - Exercise 13x - Search Strings for a Substring in Java
Learn Java - Exercise 06x - Enhanced For Loops & Arrays
Комментарии