filmov
tv
Simplifying Conditional Logic: How to Use if Statement in C+ +
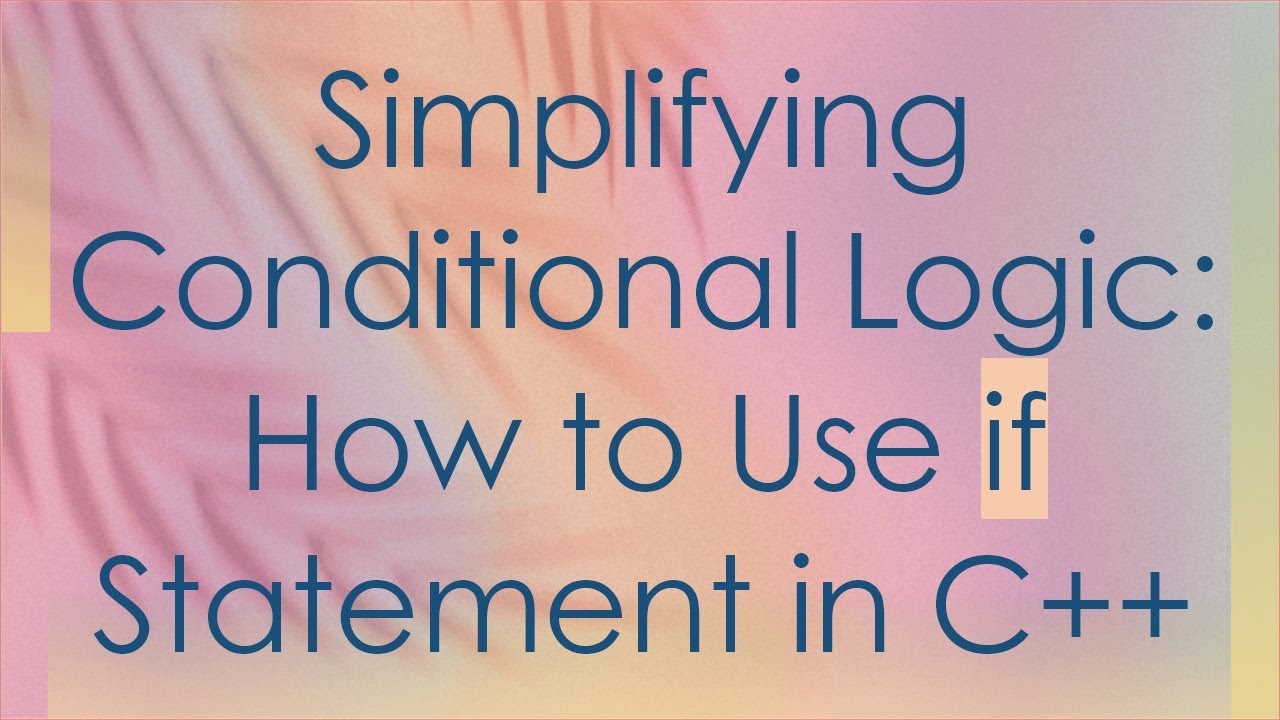
Показать описание
Learn how to replace multiple conditional checks in C+ + with a single `if` statement or by using ternary operators for improved clarity and efficiency.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How can I replace this code with one if statement?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Simplifying Conditional Logic: How to Use if Statement in C+ +
In programming, especially in C+ + , creating efficient and readable code is essential. One common challenge developers face is how to simplify conditional logic. Specifically, many question how to replace multiple if statements with a single one. In this post, we'll dive into a typical C+ + example that demonstrates this challenge and explore different methods to achieve a solution.
The Challenge
Consider the following C+ + code snippet where a variable y is assigned a value based on the value of x:
[[See Video to Reveal this Text or Code Snippet]]
The goal is to replace this code with a single if statement. Why would we want to do this? Simplifying code can enhance readability and maintainability, although care should be taken to preserve the logic.
Understanding the Solution
There are a few ways to tackle this problem, and we will discuss several approaches, highlighting their pros and cons:
1. Using Ternary Operators
One straightforward way to achieve the desired simplification is through the use of ternary operators. Here's how you can replace the multiple if statements with a single expression:
[[See Video to Reveal this Text or Code Snippet]]
Pros
The code is concise and easy to write.
It's a common pattern in C+ + for handling multiple outcomes based on conditions.
Cons
Readability can suffer if the number of conditions is high or if conditions are complex.
2. Using a Single if Statement with Combined Logic
If you specifically need to use only one if statement while retaining logical clarity, consider the following approach:
[[See Video to Reveal this Text or Code Snippet]]
Explanation
This method evaluates conditions while combining the outcomes into a single expression.
It contains only one if, making it fit the challenge but is less readable than the previous method.
3. An Improved One-If Variant
A more elegant approach, maintaining the requirement for just one if, looks like this:
[[See Video to Reveal this Text or Code Snippet]]
Benefits
This variant is simple and retains clarity.
It allows for easy adjustments in the calculation logic without increasing complexity.
4. Constant Cutoff Approach
If readability is essential, consider using constants to define thresholds, prompting encapsulation and maintainability:
[[See Video to Reveal this Text or Code Snippet]]
Advantages
It makes the cutoff easy to adjust and understand.
The logic remains straightforward and maintains clarity.
Conclusion
While simplifying conditional statements in C+ + can be approached in various ways, each method presents unique advantages. Depending on the context—whether it's a programming challenge or production code—choose the approach that balances readability and efficiency. Always prioritize writing clear and maintainable code, especially in collaborative environments.
If you're faced with similar challenges, try applying these principles to make your code not only functional but also elegant and comprehensible.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How can I replace this code with one if statement?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Simplifying Conditional Logic: How to Use if Statement in C+ +
In programming, especially in C+ + , creating efficient and readable code is essential. One common challenge developers face is how to simplify conditional logic. Specifically, many question how to replace multiple if statements with a single one. In this post, we'll dive into a typical C+ + example that demonstrates this challenge and explore different methods to achieve a solution.
The Challenge
Consider the following C+ + code snippet where a variable y is assigned a value based on the value of x:
[[See Video to Reveal this Text or Code Snippet]]
The goal is to replace this code with a single if statement. Why would we want to do this? Simplifying code can enhance readability and maintainability, although care should be taken to preserve the logic.
Understanding the Solution
There are a few ways to tackle this problem, and we will discuss several approaches, highlighting their pros and cons:
1. Using Ternary Operators
One straightforward way to achieve the desired simplification is through the use of ternary operators. Here's how you can replace the multiple if statements with a single expression:
[[See Video to Reveal this Text or Code Snippet]]
Pros
The code is concise and easy to write.
It's a common pattern in C+ + for handling multiple outcomes based on conditions.
Cons
Readability can suffer if the number of conditions is high or if conditions are complex.
2. Using a Single if Statement with Combined Logic
If you specifically need to use only one if statement while retaining logical clarity, consider the following approach:
[[See Video to Reveal this Text or Code Snippet]]
Explanation
This method evaluates conditions while combining the outcomes into a single expression.
It contains only one if, making it fit the challenge but is less readable than the previous method.
3. An Improved One-If Variant
A more elegant approach, maintaining the requirement for just one if, looks like this:
[[See Video to Reveal this Text or Code Snippet]]
Benefits
This variant is simple and retains clarity.
It allows for easy adjustments in the calculation logic without increasing complexity.
4. Constant Cutoff Approach
If readability is essential, consider using constants to define thresholds, prompting encapsulation and maintainability:
[[See Video to Reveal this Text or Code Snippet]]
Advantages
It makes the cutoff easy to adjust and understand.
The logic remains straightforward and maintains clarity.
Conclusion
While simplifying conditional statements in C+ + can be approached in various ways, each method presents unique advantages. Depending on the context—whether it's a programming challenge or production code—choose the approach that balances readability and efficiency. Always prioritize writing clear and maintainable code, especially in collaborative environments.
If you're faced with similar challenges, try applying these principles to make your code not only functional but also elegant and comprehensible.