filmov
tv
MAXIMUM SWAP | LEETCODE 670 | PYTHON SOLUTION
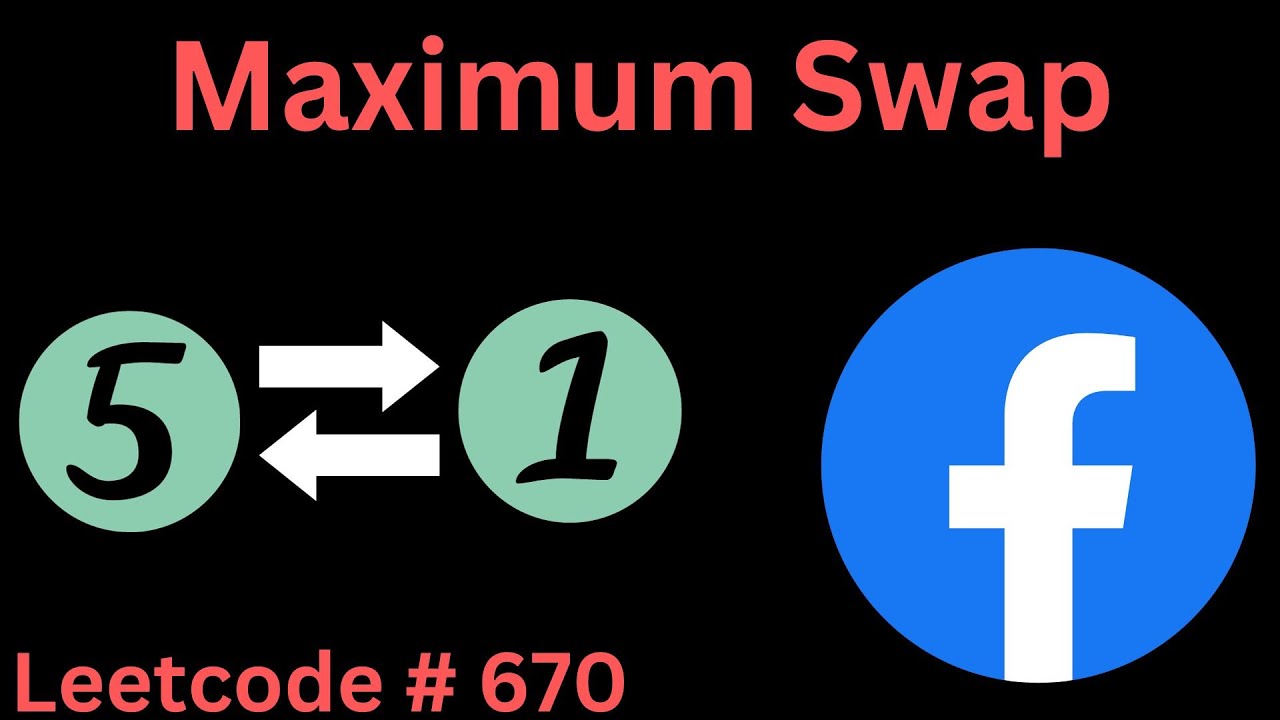
Показать описание
Today we are solving a god awful problem: Maximum Swap. It's a question that is pretty easy to grasp in terms of the intuition of the problem but it's such a chore to actually code up. There's so many annoying nuances in this problem that just add up and give you a headache.
TIMESTAMPS
00:00 Intro
00:20 Question Prompt
00:28 Basic Example
01:38 Solution Intuition
02:57 Coding
12:30 Time/Space Complexity
13:55 Outro
Maximum Swaps - Leetcode 670 - Python
Maximum Swap | 4 techniques | Leetcode 670
MAXIMUM SWAP | LEETCODE 670 | PYTHON SOLUTION
Maximum Swap - Leetcode 670 - Java
Maximum Swap | 2 Simple Approaches | Leetcode 670 | codestorywithMIK
LeetCode 670. Maximum Swap Explanation and Solution
670. Maximum Swap | Leetcode Daily (POTD) 17 Oct 2024 | Medium | Java | Hindi
LeetCode 670. Maximum Swap
Leetcode #670 - Maximum Swap (Solution)
#LeetCode 670 | Maximum Swap #Java
670. Maximum Swap | Leetcode Daily Challenge | DSA | Java | FAANG
Maximum Swap | Leetcode #670 | Interview Help
Leetcode: 670 Maximum Swap
Maximum Swap | Leetcode 670
Leetcode 670. Maximum Swap
Maximum Swap | Leetcode 670 Solution in Hindi
Maximum Swap | Leetcode 670 | Java Code | Developer Coder
670. Maximum Swap | Editorial | Leetcode | #leetcode #dailychallenge #coding
Leetcode 670 Maximum Swap
Leetcode 670: Maximum Swap
Leetcode 670 - Maximum Swap - Python Solution
Maximum Swap | LeetCode 670 | Python
670. Maximum Swap | C++ | Simple and easy explanation | Leetcode daily question | DSA
Leetcode 670 Maximum Swap
Комментарии