filmov
tv
Solving 100 Python NumPy Problems! (From easy to difficult)
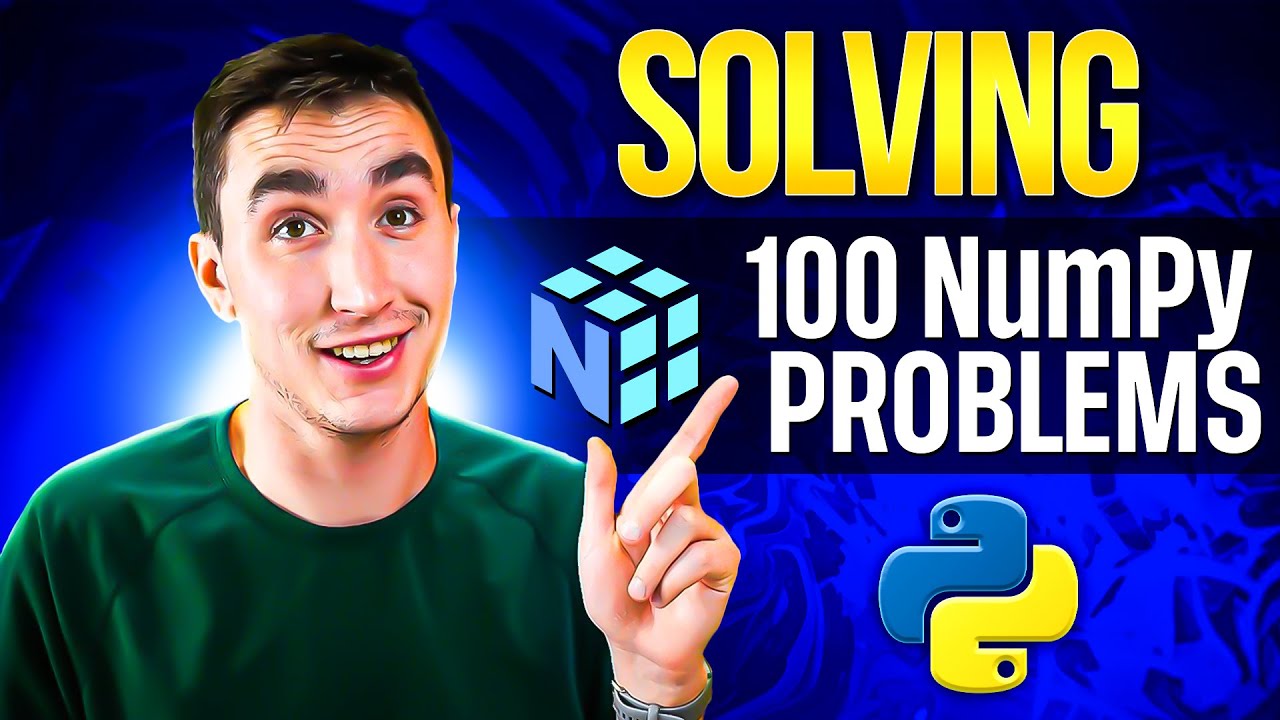
Показать описание
NumPy is a foundational library for computation in Python. In this video we walk through exercises to learn the library in a hands-on manner. We learn skills such as array creation and manipulation, working with random numbers, performing mathematical operations, handling dates, dealing with various data types, and more. Should be a lot of fun!
If you enjoy this video, make sure to throw it a like & subscribe if you haven't already 🫡
Here is a link to the similar video I did with the Python Pandas library!
Video timeline!
0:00 - Video Overview & Code Setup
4:18 - 1.) Import the numpy package under the name np
5:15 - 2.) Print the numpy version and the configuration
6:21 - 3.) Create a null vector of size 10
9:29 - 4.) How to get the memory size of any array
15:19 - 5.) How to get documentation of the numpy add function from the command line
18:51 - 6.) Create a null vector of size 10 but the fifth value which is 1
20:03 - 7.) Create a vector with values ranging from 10 to 49
21:48 - 8.) Reverse a vector (first number becomes last)
23:20 - 9.) Create a 3x3 Matrix with values ranging from 0 to 8
24:41 - 10.) Find indices of non-zero elements from array
26:24 - 11.) Create a 3x3 identity matrix
29:35 - 12.) Create a 3x3x3 array with random values.
30:48 - 13.) Create a 10x10 array with random values and find min/max values
33:17 - 14.) Create a random vector of size 30 and find the mean value
34:57 - 15.) Create a 2d array with 1 on the border and 0 inside
48:32 - 18.) Create a 5x5 matrix with values 1,2,3,4 just below the diagonal
56:01 - 19.) Create an 8x8 matrix and fill it with a checkerboard pattern
1:02:35 - 20.) Get the 100th element from a (6,7,8) shape array
1:16:22 - 22.) Normalize a random 5x5 matrix
1:24:20 - 23.) Create a custom dtype that describes a color as four unsigned bytes (RGBA)
1:29:27 - 24.) Multiply a 5x3 matrix by a 3x2 matrix (real matrix product)
1:32:54 - 25.) Given a 1D array, negate all elements which are between 3 and 8, in place
1:37:16 - 26.) Default “range” function vs numpy “range” function
1:40:25 - 27.) Evaluate whether expressions are legal or not
1:57:48 - 29.) How to round away from zero a float array?
1:59:22 - 30.) How to find common values between two arrays?
2:00:19 - 31.) How to ignore all numpy warnings?
2:05:22 - 33.) Get the dates of yesterday, today, and tomorrow with numpy
2:19:39 - 34.) How to get all the dates corresponding to the month of July 2016?
2:27:27 - 35.) How to compute ((A+B)*(-A/2)) in place (without copy)?
2:35:00 - 36.) Extract the integer part of a random array of positive numbers using 4 different methods
2:40:47 - 37.) Create a 5x5 matrix with row values ranging from 0 to 4
2:43:07 - 38.) Use generator function that generates 10 integers and use it to build an array
2:43:58 - 39.) Create a vector of size 10 with values ranging from 0 to 1, both excluded.
2:48:49 - 40.) Create a random vector of size 10 and sort it.
2:54:37 - 42.) Check if two random arrays A & B are equal
2:58:48 - 43.) Make an array immutable (read-only)
3:02:14 - Puppies are great
3:03:06 - 44.) Convert cartesian coordinates to polar coordinates
3:20:37 - 45.) Create a random vector of size 10 and replace the maximum value by 0
3:23:58 - 46.) Create a structured array with x and y coordinates covering the [0,1]x[0,1] area
3:26:25 - 47.) Given two arrays, X and Y, construct the Cauchy matrix C (Cij = 1/(xi-yj))
3:34:31 - 48.) Print the min/max values for each numpy scalar type
3:36:50 - 49.) How to print all the values of an array?
3:39:23 - 50.) How to find the closest value (to a given scalar) in a vector?
I got a little tired at the end, so not doing all 100 problems in this video. Will release the next 50 problems soon!
#python #numpy
--------------------
Follow me on social media!
--------------------
Learn data skills with hands-on exercises & tutorials at Datacamp!
Practice your Python Pandas data science skills with problems on StrataScratch!
*I use affiliate links on the products that I recommend. I may earn a purchase commission or a referral bonus from the usage of these links.
If you enjoy this video, make sure to throw it a like & subscribe if you haven't already 🫡
Here is a link to the similar video I did with the Python Pandas library!
Video timeline!
0:00 - Video Overview & Code Setup
4:18 - 1.) Import the numpy package under the name np
5:15 - 2.) Print the numpy version and the configuration
6:21 - 3.) Create a null vector of size 10
9:29 - 4.) How to get the memory size of any array
15:19 - 5.) How to get documentation of the numpy add function from the command line
18:51 - 6.) Create a null vector of size 10 but the fifth value which is 1
20:03 - 7.) Create a vector with values ranging from 10 to 49
21:48 - 8.) Reverse a vector (first number becomes last)
23:20 - 9.) Create a 3x3 Matrix with values ranging from 0 to 8
24:41 - 10.) Find indices of non-zero elements from array
26:24 - 11.) Create a 3x3 identity matrix
29:35 - 12.) Create a 3x3x3 array with random values.
30:48 - 13.) Create a 10x10 array with random values and find min/max values
33:17 - 14.) Create a random vector of size 30 and find the mean value
34:57 - 15.) Create a 2d array with 1 on the border and 0 inside
48:32 - 18.) Create a 5x5 matrix with values 1,2,3,4 just below the diagonal
56:01 - 19.) Create an 8x8 matrix and fill it with a checkerboard pattern
1:02:35 - 20.) Get the 100th element from a (6,7,8) shape array
1:16:22 - 22.) Normalize a random 5x5 matrix
1:24:20 - 23.) Create a custom dtype that describes a color as four unsigned bytes (RGBA)
1:29:27 - 24.) Multiply a 5x3 matrix by a 3x2 matrix (real matrix product)
1:32:54 - 25.) Given a 1D array, negate all elements which are between 3 and 8, in place
1:37:16 - 26.) Default “range” function vs numpy “range” function
1:40:25 - 27.) Evaluate whether expressions are legal or not
1:57:48 - 29.) How to round away from zero a float array?
1:59:22 - 30.) How to find common values between two arrays?
2:00:19 - 31.) How to ignore all numpy warnings?
2:05:22 - 33.) Get the dates of yesterday, today, and tomorrow with numpy
2:19:39 - 34.) How to get all the dates corresponding to the month of July 2016?
2:27:27 - 35.) How to compute ((A+B)*(-A/2)) in place (without copy)?
2:35:00 - 36.) Extract the integer part of a random array of positive numbers using 4 different methods
2:40:47 - 37.) Create a 5x5 matrix with row values ranging from 0 to 4
2:43:07 - 38.) Use generator function that generates 10 integers and use it to build an array
2:43:58 - 39.) Create a vector of size 10 with values ranging from 0 to 1, both excluded.
2:48:49 - 40.) Create a random vector of size 10 and sort it.
2:54:37 - 42.) Check if two random arrays A & B are equal
2:58:48 - 43.) Make an array immutable (read-only)
3:02:14 - Puppies are great
3:03:06 - 44.) Convert cartesian coordinates to polar coordinates
3:20:37 - 45.) Create a random vector of size 10 and replace the maximum value by 0
3:23:58 - 46.) Create a structured array with x and y coordinates covering the [0,1]x[0,1] area
3:26:25 - 47.) Given two arrays, X and Y, construct the Cauchy matrix C (Cij = 1/(xi-yj))
3:34:31 - 48.) Print the min/max values for each numpy scalar type
3:36:50 - 49.) How to print all the values of an array?
3:39:23 - 50.) How to find the closest value (to a given scalar) in a vector?
I got a little tired at the end, so not doing all 100 problems in this video. Will release the next 50 problems soon!
#python #numpy
--------------------
Follow me on social media!
--------------------
Learn data skills with hands-on exercises & tutorials at Datacamp!
Practice your Python Pandas data science skills with problems on StrataScratch!
*I use affiliate links on the products that I recommend. I may earn a purchase commission or a referral bonus from the usage of these links.
Комментарии