filmov
tv
what is a singleton? (and python patterns) (intermediate - advanced) anthony explains #188
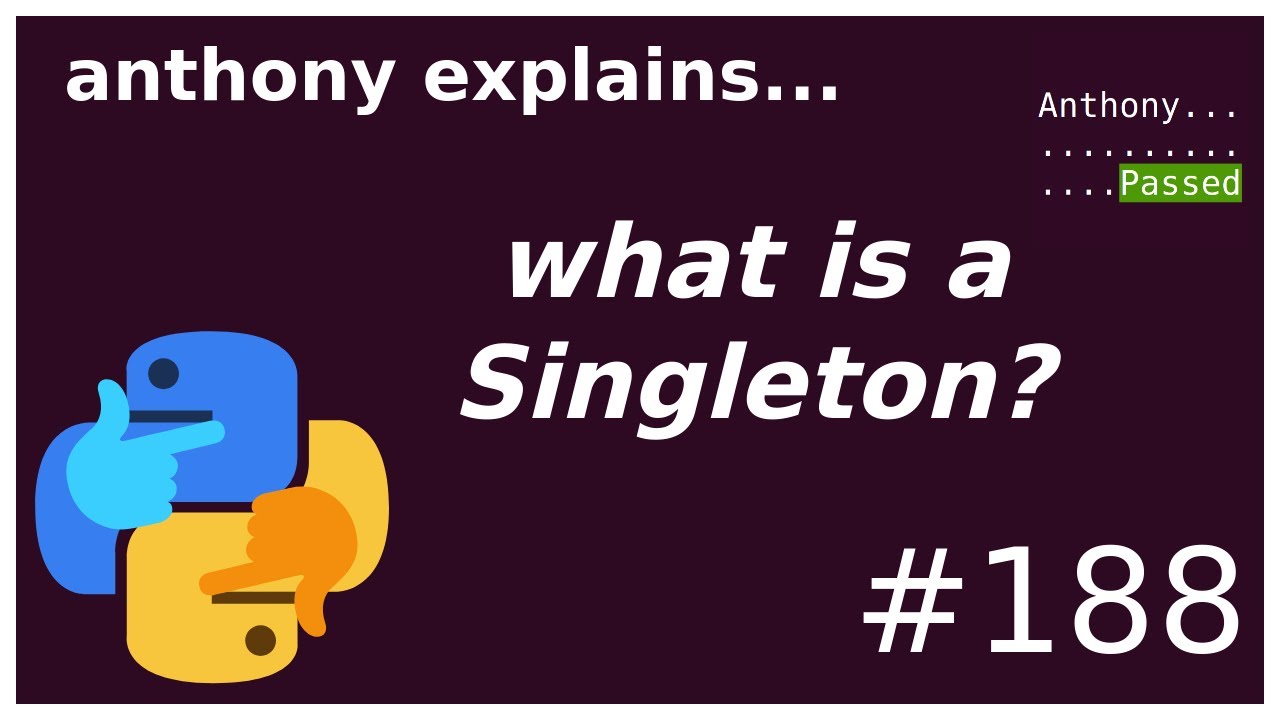
Показать описание
today I talk about the singleton pattern and a bunch of different ways to implement it in python!
==========
I won't ask for subscriptions / likes / comments in videos but it really helps the channel. If you have any suggestions or things you'd like to see please comment below!
==========
I won't ask for subscriptions / likes / comments in videos but it really helps the channel. If you have any suggestions or things you'd like to see please comment below!
what is a singleton? (and python patterns) (intermediate - advanced) anthony explains #188
What is Singleton Class in Java | Singleton Design Pattern Part 1
Singleton Pattern - Design Patterns
You HAVE To Learn Singleton in Flutter...
What is a singleton class in Java | Singleton design pattern | automateNow
What is the Singleton Pattern? (Software Design Patterns)
The Singleton Pattern Explained and Implemented in Java | Creational Design Patterns | Geekific
What Is A Singleton and How To Create One In Swift
How to crack Low level design interviews in 2025? | LLD Roadmap
The Singleton Design Pattern - Part of the Gang of Four
GO | Singleton Pattern with easy example in Code
How to create a Singleton? - Cracking the Java Coding Interview
Singleton Design Pattern - Advanced Python Tutorial #9
What is Singleton Pattern in Java - How to achieve this?
Singleton Design Pattern - What is it? And a Simple Example Implementing a Singleton Class in C#
What is Singleton?
Singleton Design Pattern Interview Questions
Singleton Classes In Java #java #javaframework #coding #programming
How To Implement A Lazy Singleton Class #shorts
Singleton Class explained #mysirg
Singleton Design Pattern Use Cases & Examples! #shorts
#3: Singletons vs @Singleton - Android Interviews #Shorts
Singleton and Double Checked Locking
Singleton Pattern – Design Patterns (ep 6)
Комментарии