filmov
tv
How to Group a JavaScript Object? A Simple Guide to Data Processing
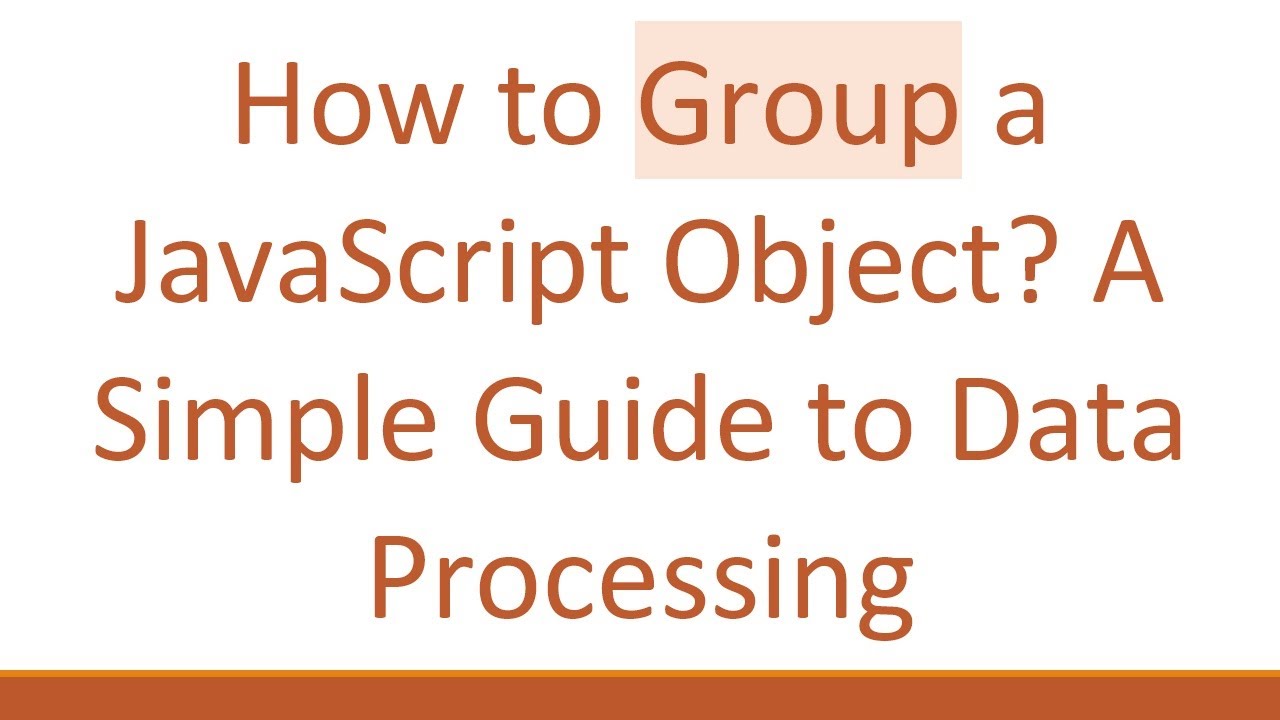
Показать описание
Learn how to `group` an array of JavaScript objects to effectively organize and manipulate your data. This guide walks you through the process step-by-step with code examples.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to group an javascript object?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Group a JavaScript Object? A Simple Guide to Data Processing
Working with arrays of objects in JavaScript is a common practice, especially when dealing with data from databases. One common challenge developers face is how to group these objects based on certain properties. In this guide, we will explore how to effectively group objects in JavaScript using the reduce function and a Map.
Introduction to the Problem
Suppose you have an array of objects representing some data from a database, like this:
[[See Video to Reveal this Text or Code Snippet]]
Your goal is to transform this array into a new structure that groups the data by date, combining the values of each type. The expected output should look like this:
[[See Video to Reveal this Text or Code Snippet]]
Attempting a Solution
Initially, you might try to use the reduce function like this:
[[See Video to Reveal this Text or Code Snippet]]
However, while this attempts to group the data, it can leave the values unmodified or incorrectly structured.
Optimal Solution Using Map and ForEach
To achieve the required transformation effectively, we can utilize a Map object for storing our values. This approach not only helps in better organizing our data but also allows for easy accumulation of values where multiple entries for the same type exist.
Step-by-Step Explanation
Initialize a Map: Create a new instance of a Map to hold your grouped data.
Iterate Through the Array: Use forEach to loop through each object in the original array.
Check for Existing Dates: For each object, check if the date already exists in the Map.
Store or Update Values:
If it doesn't exist, create a new entry.
If it does exist, update the corresponding type value by adding the new value to the existing one.
Convert the Map to an Array: Once you’ve processed all the objects, convert the values of the Map back into an array.
Here’s the working code:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
Map Initialization: const map = new Map();
ForEach Loop: This will loop through arr and destructure each object into date, type, and value.
Check if Map has Date: If a date is already stored in map, we update the corresponding type with the cumulative value. If not, we create a new entry.
Output
The final result will be:
[[See Video to Reveal this Text or Code Snippet]]
This approach is efficient, clean and takes care of potential duplicate types for each date.
Conclusion
Grouping JavaScript objects by specific properties such as date and type requires a careful approach to ensure we correctly organize and sum values where necessary. By using a combination of forEach and the powerful Map object, we can succinctly achieve our objective with clarity and efficiency. Improve your JavaScript skills by mastering this grouping technique!
Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to group an javascript object?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Group a JavaScript Object? A Simple Guide to Data Processing
Working with arrays of objects in JavaScript is a common practice, especially when dealing with data from databases. One common challenge developers face is how to group these objects based on certain properties. In this guide, we will explore how to effectively group objects in JavaScript using the reduce function and a Map.
Introduction to the Problem
Suppose you have an array of objects representing some data from a database, like this:
[[See Video to Reveal this Text or Code Snippet]]
Your goal is to transform this array into a new structure that groups the data by date, combining the values of each type. The expected output should look like this:
[[See Video to Reveal this Text or Code Snippet]]
Attempting a Solution
Initially, you might try to use the reduce function like this:
[[See Video to Reveal this Text or Code Snippet]]
However, while this attempts to group the data, it can leave the values unmodified or incorrectly structured.
Optimal Solution Using Map and ForEach
To achieve the required transformation effectively, we can utilize a Map object for storing our values. This approach not only helps in better organizing our data but also allows for easy accumulation of values where multiple entries for the same type exist.
Step-by-Step Explanation
Initialize a Map: Create a new instance of a Map to hold your grouped data.
Iterate Through the Array: Use forEach to loop through each object in the original array.
Check for Existing Dates: For each object, check if the date already exists in the Map.
Store or Update Values:
If it doesn't exist, create a new entry.
If it does exist, update the corresponding type value by adding the new value to the existing one.
Convert the Map to an Array: Once you’ve processed all the objects, convert the values of the Map back into an array.
Here’s the working code:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
Map Initialization: const map = new Map();
ForEach Loop: This will loop through arr and destructure each object into date, type, and value.
Check if Map has Date: If a date is already stored in map, we update the corresponding type with the cumulative value. If not, we create a new entry.
Output
The final result will be:
[[See Video to Reveal this Text or Code Snippet]]
This approach is efficient, clean and takes care of potential duplicate types for each date.
Conclusion
Grouping JavaScript objects by specific properties such as date and type requires a careful approach to ensure we correctly organize and sum values where necessary. By using a combination of forEach and the powerful Map object, we can succinctly achieve our objective with clarity and efficiency. Improve your JavaScript skills by mastering this grouping technique!
Happy coding!