filmov
tv
How To Iterate On Lists Using For Loop | Python 4 You | Lecture 229
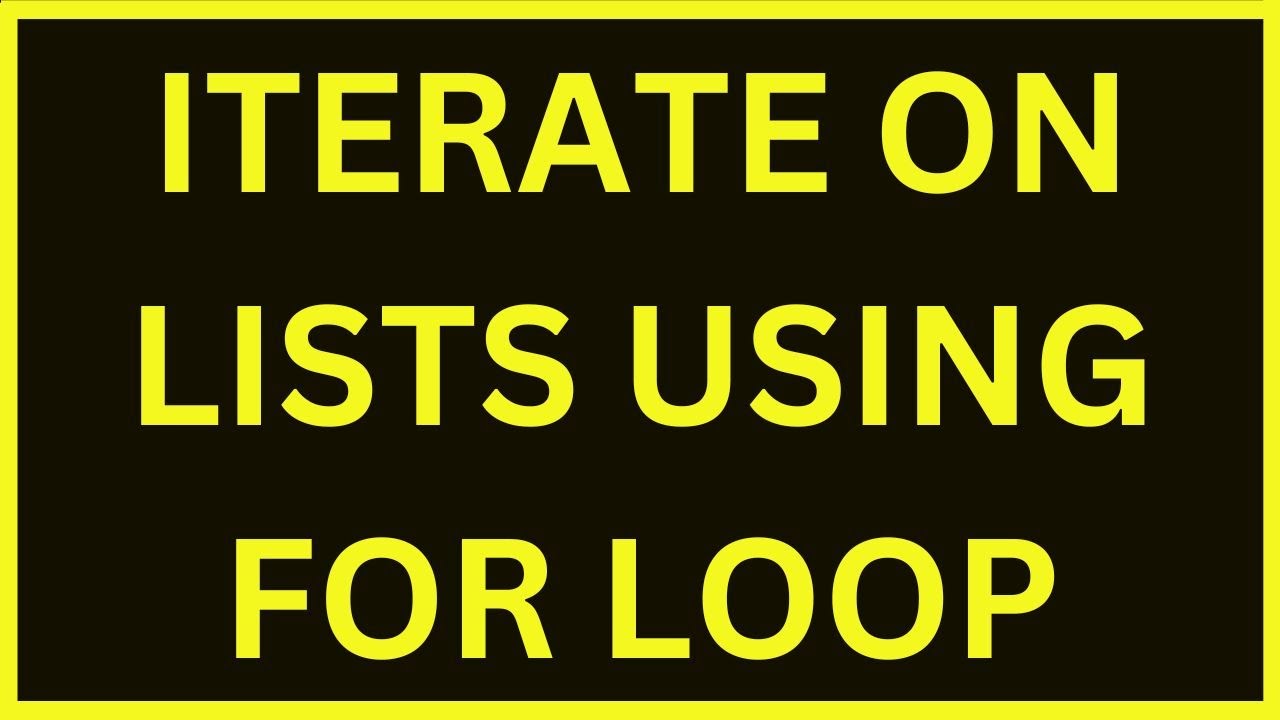
Показать описание
Exploring List Iteration with For Loops in Python: A Comprehensive Guide
Iteration is a fundamental concept in programming, and when it comes to working with lists in Python, the for loop stands out as a powerful and versatile tool. In this comprehensive guide, we will delve into the intricacies of iterating over lists using for loops, exploring various aspects such as basic iteration, customization, and efficiency considerations.
Understanding Lists in Python:
Before diving into list iteration, let's revisit the basic characteristics of lists in Python. A list is a mutable, ordered collection of elements. It can contain items of different data types, and its elements are accessed by index. Lists are widely used for storing and manipulating data, making them an essential part of Python programming.
Basic List Iteration:
The most common use of a for loop with lists is for basic iteration, where each element of the list is accessed in sequence. This straightforward approach is useful for tasks that involve processing each item in the list.
python
my_list = [1, 2, 3, 4, 5]
for item in my_list:
print(item)
In this example, the for loop iterates over each element in `my_list`, and the `print()` statement displays each item. This fundamental form of list iteration is the building block for more complex operations.
Accessing Index and Value Together:
While iterating over a list, it is often useful to access both the index and the corresponding value. The `enumerate()` function facilitates this by returning both the index and the value in each iteration.
my_list = ['apple', 'banana', 'orange']
for index, value in enumerate(my_list):
print(f"Index: {index}, Value: {value}")
Here, `enumerate()` provides a convenient way to obtain both the index and the value, enhancing the versatility of list iteration.
Customizing List Iteration:
List iteration can be customized based on specific requirements. For instance, using conditional statements within the loop allows selective processing of elements.
numbers = [1, 2, 3, 4, 5]
for number in numbers:
if number % 2 == 0:
print(f"Even number: {number}")
else:
print(f"Odd number: {number}")
In this example, the for loop is customized to print whether each number in the list is even or odd. This demonstrates how list iteration can be tailored to perform specific actions based on element properties.
Efficiency Considerations:
While for loops provide a flexible means of iterating over lists, it's essential to consider efficiency, especially when dealing with large datasets. For instance, if the goal is to perform an operation on each element without modifying the list, list comprehension can offer a more concise and potentially faster solution.
squares = [x**2 for x in range(1, 6)]
List comprehension allows the creation of a new list by specifying the operation to be performed on each element in a concise manner. It is a powerful construct that aligns with the principles of Pythonic code.
Conclusion:
In conclusion, iterating over lists using for loops is a fundamental skill for Python developers. Whether it's basic iteration, accessing index-value pairs, or customizing the loop for specific tasks, the for loop provides a versatile mechanism for traversing lists. As with any programming task, the choice between different methods of iteration depends on the specific requirements of the task at hand. Being mindful of efficiency considerations and Pythonic constructs such as list comprehension contributes to writing clean, readable, and performant code. Mastering list iteration is an essential step in becoming proficient in Python programming and leveraging its capabilities for data manipulation and analysis.
Useful Queries that you may like to search:
How to iterate on list using for loop in python
How to iterate on list using for loop in java
for loop in list python
python iterate list with index
python loop through list of strings
python iterate over list of objects
for loop in list java
for loop in python
People may also search for:
for loop, python for loop list, for loops, python for loop, python for beginners, for loop in python, for loop python, for loop list, for loop iterating by sequence index, python - for loop iterating by sequence index, loops in python, for loop for lists, loop, python tutorial on for loop, iterating over lists in python, python loops, iterating over a string, iterating using pointer, traversing two lists in single loop, iterating over a python string, iterating lists
#how #howto #youtube #youtubers #viralvideo #viral #python4 #pythontutorial #pythonprogramming #python3 #pythonforbeginners #pythonlectures #pythonprograms #pythonlatest #rehanblogger #python4you #pythonlatestversion #pythonlatestversion Learn python3.12.0 and latest version of python3.13. If you are searching for python3.13.0 lessons, you are at the right place as this course will be very helpful for python learners or python beginners.
Iteration is a fundamental concept in programming, and when it comes to working with lists in Python, the for loop stands out as a powerful and versatile tool. In this comprehensive guide, we will delve into the intricacies of iterating over lists using for loops, exploring various aspects such as basic iteration, customization, and efficiency considerations.
Understanding Lists in Python:
Before diving into list iteration, let's revisit the basic characteristics of lists in Python. A list is a mutable, ordered collection of elements. It can contain items of different data types, and its elements are accessed by index. Lists are widely used for storing and manipulating data, making them an essential part of Python programming.
Basic List Iteration:
The most common use of a for loop with lists is for basic iteration, where each element of the list is accessed in sequence. This straightforward approach is useful for tasks that involve processing each item in the list.
python
my_list = [1, 2, 3, 4, 5]
for item in my_list:
print(item)
In this example, the for loop iterates over each element in `my_list`, and the `print()` statement displays each item. This fundamental form of list iteration is the building block for more complex operations.
Accessing Index and Value Together:
While iterating over a list, it is often useful to access both the index and the corresponding value. The `enumerate()` function facilitates this by returning both the index and the value in each iteration.
my_list = ['apple', 'banana', 'orange']
for index, value in enumerate(my_list):
print(f"Index: {index}, Value: {value}")
Here, `enumerate()` provides a convenient way to obtain both the index and the value, enhancing the versatility of list iteration.
Customizing List Iteration:
List iteration can be customized based on specific requirements. For instance, using conditional statements within the loop allows selective processing of elements.
numbers = [1, 2, 3, 4, 5]
for number in numbers:
if number % 2 == 0:
print(f"Even number: {number}")
else:
print(f"Odd number: {number}")
In this example, the for loop is customized to print whether each number in the list is even or odd. This demonstrates how list iteration can be tailored to perform specific actions based on element properties.
Efficiency Considerations:
While for loops provide a flexible means of iterating over lists, it's essential to consider efficiency, especially when dealing with large datasets. For instance, if the goal is to perform an operation on each element without modifying the list, list comprehension can offer a more concise and potentially faster solution.
squares = [x**2 for x in range(1, 6)]
List comprehension allows the creation of a new list by specifying the operation to be performed on each element in a concise manner. It is a powerful construct that aligns with the principles of Pythonic code.
Conclusion:
In conclusion, iterating over lists using for loops is a fundamental skill for Python developers. Whether it's basic iteration, accessing index-value pairs, or customizing the loop for specific tasks, the for loop provides a versatile mechanism for traversing lists. As with any programming task, the choice between different methods of iteration depends on the specific requirements of the task at hand. Being mindful of efficiency considerations and Pythonic constructs such as list comprehension contributes to writing clean, readable, and performant code. Mastering list iteration is an essential step in becoming proficient in Python programming and leveraging its capabilities for data manipulation and analysis.
Useful Queries that you may like to search:
How to iterate on list using for loop in python
How to iterate on list using for loop in java
for loop in list python
python iterate list with index
python loop through list of strings
python iterate over list of objects
for loop in list java
for loop in python
People may also search for:
for loop, python for loop list, for loops, python for loop, python for beginners, for loop in python, for loop python, for loop list, for loop iterating by sequence index, python - for loop iterating by sequence index, loops in python, for loop for lists, loop, python tutorial on for loop, iterating over lists in python, python loops, iterating over a string, iterating using pointer, traversing two lists in single loop, iterating over a python string, iterating lists
#how #howto #youtube #youtubers #viralvideo #viral #python4 #pythontutorial #pythonprogramming #python3 #pythonforbeginners #pythonlectures #pythonprograms #pythonlatest #rehanblogger #python4you #pythonlatestversion #pythonlatestversion Learn python3.12.0 and latest version of python3.13. If you are searching for python3.13.0 lessons, you are at the right place as this course will be very helpful for python learners or python beginners.