filmov
tv
How to `Parse` and `Split` a `String` in JavaScript
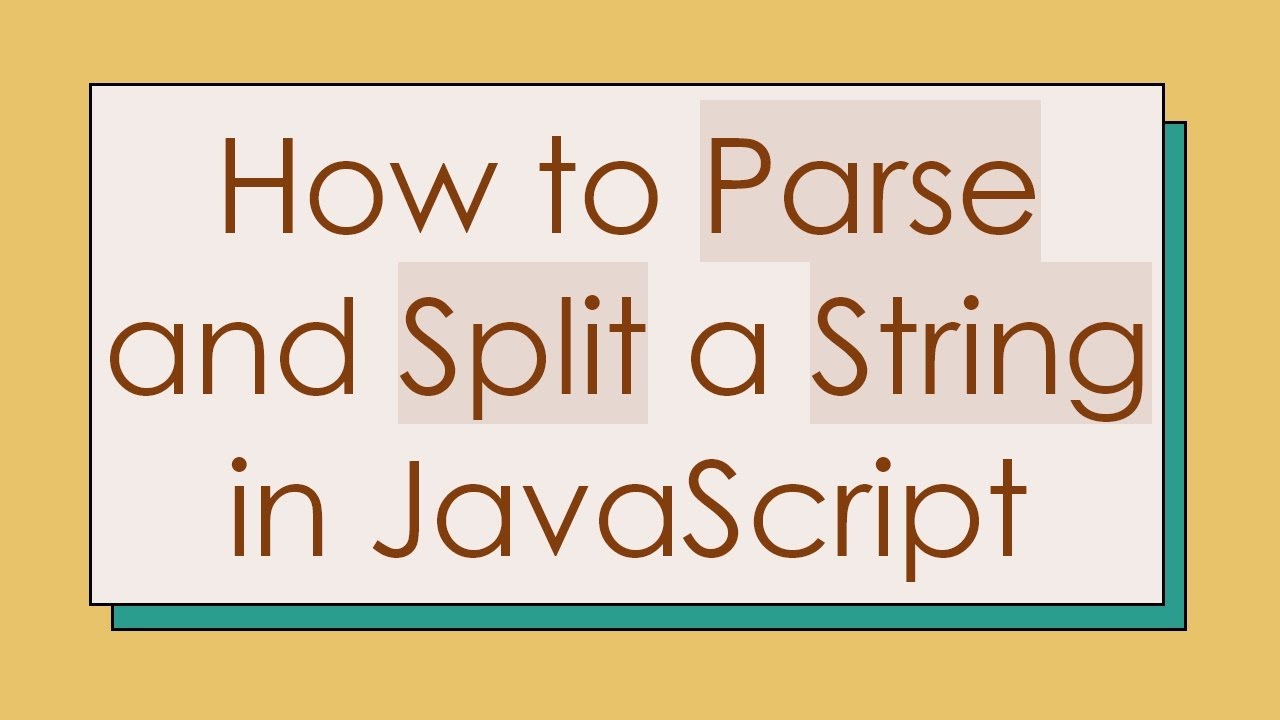
Показать описание
Summary: Learn the techniques to parse and split strings in JavaScript effectively. Expert tips on parsing JSON strings and query strings in JavaScript.
---
How to Parse and Split a String in JavaScript
String manipulation is a crucial part of programming in JavaScript. Whether you need to parse JSON data, handle query strings, or simply split strings into arrays, understanding these techniques can be immensely beneficial. This post will guide you through the essentials of parsing and splitting strings in JavaScript efficiently.
How to Parse a String in JavaScript
JavaScript provides several methods for parsing strings depending on the context and requirements.
JSON.parse()
When working with data APIs or storing configuration, JSON strings are common. JavaScript allows you to convert JSON strings into objects using the JSON.parse() method. Here's an example:
[[See Video to Reveal this Text or Code Snippet]]
JSON.parse() takes a JSON string and transforms it into a JavaScript object. This is particularly useful for dynamically handling structured data.
Query String Parsing
When working with URLs, you often deal with query strings. These are the portions of a URL that contain key=value pairs. Parsing these requires breaking them down into an object. Here’s how to do it:
[[See Video to Reveal this Text or Code Snippet]]
The URLSearchParams object helps simplify the extraction of query string parameters.
How to Split a String in JavaScript
String splitting is another essential operation which can be performed using the split() method.
split()
The split() method allows you to split a string into an array of strings based on a specified delimiter. Here's an example:
[[See Video to Reveal this Text or Code Snippet]]
The split() method takes a delimiter as an argument and returns an array of substrings. This method is useful when you need to convert CSV data or handle delimited text.
[[See Video to Reveal this Text or Code Snippet]]
In this example, the string is split at each space into an array of words.
Conclusion
Knowing how to parse and split strings in JavaScript is fundamental for effective data manipulation. Whether dealing with JSON, query strings, or simple text, these methods provide a robust toolkit for various string operations. Mastering these will certainly enhance your ability to work with data in JavaScript.
---
How to Parse and Split a String in JavaScript
String manipulation is a crucial part of programming in JavaScript. Whether you need to parse JSON data, handle query strings, or simply split strings into arrays, understanding these techniques can be immensely beneficial. This post will guide you through the essentials of parsing and splitting strings in JavaScript efficiently.
How to Parse a String in JavaScript
JavaScript provides several methods for parsing strings depending on the context and requirements.
JSON.parse()
When working with data APIs or storing configuration, JSON strings are common. JavaScript allows you to convert JSON strings into objects using the JSON.parse() method. Here's an example:
[[See Video to Reveal this Text or Code Snippet]]
JSON.parse() takes a JSON string and transforms it into a JavaScript object. This is particularly useful for dynamically handling structured data.
Query String Parsing
When working with URLs, you often deal with query strings. These are the portions of a URL that contain key=value pairs. Parsing these requires breaking them down into an object. Here’s how to do it:
[[See Video to Reveal this Text or Code Snippet]]
The URLSearchParams object helps simplify the extraction of query string parameters.
How to Split a String in JavaScript
String splitting is another essential operation which can be performed using the split() method.
split()
The split() method allows you to split a string into an array of strings based on a specified delimiter. Here's an example:
[[See Video to Reveal this Text or Code Snippet]]
The split() method takes a delimiter as an argument and returns an array of substrings. This method is useful when you need to convert CSV data or handle delimited text.
[[See Video to Reveal this Text or Code Snippet]]
In this example, the string is split at each space into an array of words.
Conclusion
Knowing how to parse and split strings in JavaScript is fundamental for effective data manipulation. Whether dealing with JSON, query strings, or simple text, these methods provide a robust toolkit for various string operations. Mastering these will certainly enhance your ability to work with data in JavaScript.