filmov
tv
Managing Multiple User Inputs in React Native with One onChange Function
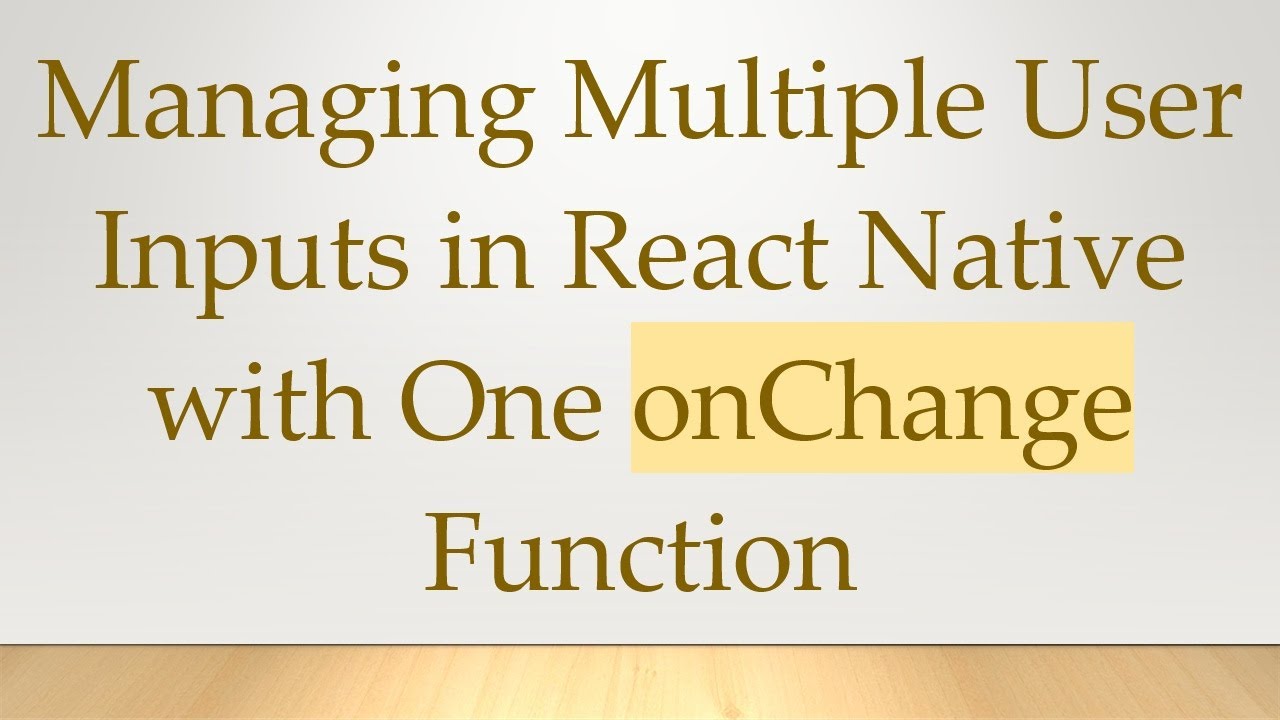
Показать описание
Learn how to effectively manage multiple text inputs in React Native using a single `onChange` handler. Discover step-by-step solutions to save your inputs with just one button click.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How do I manage multiple user inputs with only one onChange in React Native?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Managing Multiple User Inputs in React Native with One onChange Function
Working with forms can be a bit challenging, especially when you have multiple inputs that require handling in a React Native application. If you ever found yourself tangled in managing various text fields and wanted a more efficient way to handle user inputs, this guide is for you. We’ll break down how to manage multiple user inputs with just one onChange function and save those inputs efficiently.
The Problem
Consider a scenario where you want users to register details about movies they wish to watch. This involves several fields like:
Name of the movie
Synopsis
Comments
Source of where to watch
As a beginner, managing separate state variables for each input can be overwhelming. The goal is to have all these inputs covered by a singular onChange handler while also providing a button to save the data.
The Solution
To simplify the process of managing inputs in your React Native app, we will leverage an object to hold all user input values, and a single function to update this object. Below are the detailed steps to achieve this.
1. Set Up State Management
Instead of creating separate state variables for each input, we'll maintain a single object in the state:
[[See Video to Reveal this Text or Code Snippet]]
2. Create a Unified Input Handler
We will create a function, handleChange, that will dynamically update the state based on the input field that is being modified:
[[See Video to Reveal this Text or Code Snippet]]
3. Integrate the Change Handler into Your Inputs
You can now utilize this single handler in your TextInput components. Here’s how you can do it:
[[See Video to Reveal this Text or Code Snippet]]
4. Saving the Input Data
To save all the data at once, you will only need to call a function when the button is pressed. Here’s how it can look:
[[See Video to Reveal this Text or Code Snippet]]
And your button can call this submit handler:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By following the above steps, you've effectively managed multiple user inputs using a single onChange function in your React Native application. This simplifies your code, makes it more maintainable, and enhances performance by reducing the number of state updates.
Feel free to expand this initial structure with more complex validations or additional features, such as data storage using AsyncStorage. With practice, you'll gain more confidence in managing forms within React Native!
Now, go ahead and implement this in your project, and remember: when working with forms, keeping things simple and organized is key to great user experiences.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How do I manage multiple user inputs with only one onChange in React Native?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Managing Multiple User Inputs in React Native with One onChange Function
Working with forms can be a bit challenging, especially when you have multiple inputs that require handling in a React Native application. If you ever found yourself tangled in managing various text fields and wanted a more efficient way to handle user inputs, this guide is for you. We’ll break down how to manage multiple user inputs with just one onChange function and save those inputs efficiently.
The Problem
Consider a scenario where you want users to register details about movies they wish to watch. This involves several fields like:
Name of the movie
Synopsis
Comments
Source of where to watch
As a beginner, managing separate state variables for each input can be overwhelming. The goal is to have all these inputs covered by a singular onChange handler while also providing a button to save the data.
The Solution
To simplify the process of managing inputs in your React Native app, we will leverage an object to hold all user input values, and a single function to update this object. Below are the detailed steps to achieve this.
1. Set Up State Management
Instead of creating separate state variables for each input, we'll maintain a single object in the state:
[[See Video to Reveal this Text or Code Snippet]]
2. Create a Unified Input Handler
We will create a function, handleChange, that will dynamically update the state based on the input field that is being modified:
[[See Video to Reveal this Text or Code Snippet]]
3. Integrate the Change Handler into Your Inputs
You can now utilize this single handler in your TextInput components. Here’s how you can do it:
[[See Video to Reveal this Text or Code Snippet]]
4. Saving the Input Data
To save all the data at once, you will only need to call a function when the button is pressed. Here’s how it can look:
[[See Video to Reveal this Text or Code Snippet]]
And your button can call this submit handler:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By following the above steps, you've effectively managed multiple user inputs using a single onChange function in your React Native application. This simplifies your code, makes it more maintainable, and enhances performance by reducing the number of state updates.
Feel free to expand this initial structure with more complex validations or additional features, such as data storage using AsyncStorage. With practice, you'll gain more confidence in managing forms within React Native!
Now, go ahead and implement this in your project, and remember: when working with forms, keeping things simple and organized is key to great user experiences.