filmov
tv
Converting SQL Queries to LINQ in C# Web API
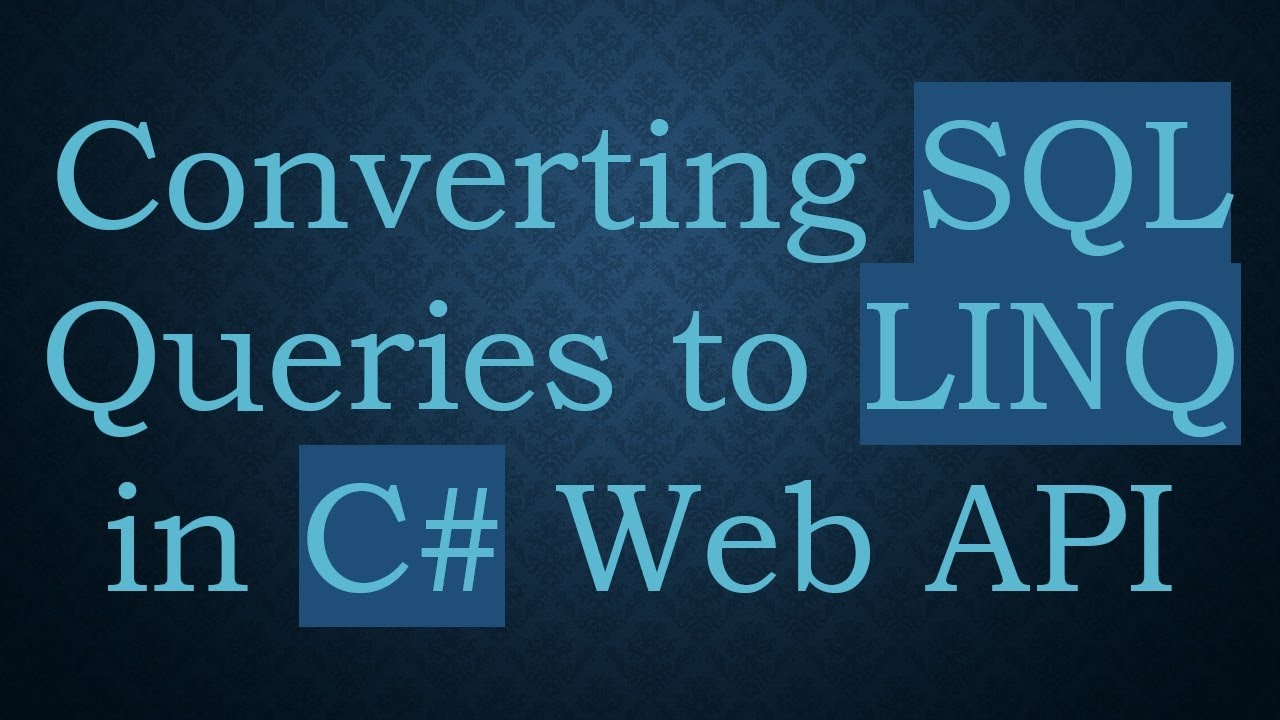
Показать описание
Learn how to effectively convert SQL queries into LINQ expressions in C-. This guide provides a detailed breakdown of the process to ensure success in your projects!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: SQL TO linq in c-
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Converting SQL Queries to LINQ in C- Web API: A Step-by-Step Guide
In modern software development, especially when working with .NET applications, it's crucial to understand how to interact with databases effectively. One common challenge developers face is converting SQL queries into LINQ (Language-Integrated Query). This conversion is not only essential for maintaining readability and functionality in C- applications but also for leveraging the advantages of strong typing and compiler checks that LINQ provides.
In this post, we'll explore how to convert a specific SQL query into an equivalent LINQ expression within a C- Web API context.
The SQL Query
Let’s start by examining the SQL query in question. Here’s the SQL code:
[[See Video to Reveal this Text or Code Snippet]]
This query selects branch names from the Branch table where there are no corresponding entries in the vBranching view for a specific username ('xCuttiepies').
The Initial LINQ Attempt
The developer initially attempted the following LINQ translation:
[[See Video to Reveal this Text or Code Snippet]]
However, this code contains several issues that prevent it from functioning correctly:
Improper Use of ToList(): The ToList() method blocks the execution of the query at that point, which is not desirable when we want to filter records.
Wrong Use of Lambda Syntax: The lambda expressions do not correctly represent the desired conditions.
Incorrect Return Value: The returned variable vBranching does not match the SQL query's intent.
The Correct Approach
To correctly implement the desired functionality in LINQ, we need to keep the database query intact until the last possible moment. Here’s the correct version of the LINQ query:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Correct Query
Using Any Instead of Exists: In LINQ, the equivalent of the SQL EXISTS is the Any method. This method checks if any records match the specified condition.
Deferring Execution: By not calling ToList() until the end, we allow LINQ to build a complete query that can be executed once and retrieves only the necessary data.
Returning the Correct Result: The final list of branch names is returned as intended, matching the SQL query's outcome.
Conclusion
Converting SQL to LINQ might seem daunting at first, but with careful attention to detail, it can be done easily. Always remember to ensure that your syntax aligns with LINQ conventions, use methods like Any for existence checks, and defer execution until the final result is needed.
By following the outlined steps, you can effectively transform your SQL queries into LINQ expressions in your C- Web APIs or any other .NET applications. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: SQL TO linq in c-
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Converting SQL Queries to LINQ in C- Web API: A Step-by-Step Guide
In modern software development, especially when working with .NET applications, it's crucial to understand how to interact with databases effectively. One common challenge developers face is converting SQL queries into LINQ (Language-Integrated Query). This conversion is not only essential for maintaining readability and functionality in C- applications but also for leveraging the advantages of strong typing and compiler checks that LINQ provides.
In this post, we'll explore how to convert a specific SQL query into an equivalent LINQ expression within a C- Web API context.
The SQL Query
Let’s start by examining the SQL query in question. Here’s the SQL code:
[[See Video to Reveal this Text or Code Snippet]]
This query selects branch names from the Branch table where there are no corresponding entries in the vBranching view for a specific username ('xCuttiepies').
The Initial LINQ Attempt
The developer initially attempted the following LINQ translation:
[[See Video to Reveal this Text or Code Snippet]]
However, this code contains several issues that prevent it from functioning correctly:
Improper Use of ToList(): The ToList() method blocks the execution of the query at that point, which is not desirable when we want to filter records.
Wrong Use of Lambda Syntax: The lambda expressions do not correctly represent the desired conditions.
Incorrect Return Value: The returned variable vBranching does not match the SQL query's intent.
The Correct Approach
To correctly implement the desired functionality in LINQ, we need to keep the database query intact until the last possible moment. Here’s the correct version of the LINQ query:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Correct Query
Using Any Instead of Exists: In LINQ, the equivalent of the SQL EXISTS is the Any method. This method checks if any records match the specified condition.
Deferring Execution: By not calling ToList() until the end, we allow LINQ to build a complete query that can be executed once and retrieves only the necessary data.
Returning the Correct Result: The final list of branch names is returned as intended, matching the SQL query's outcome.
Conclusion
Converting SQL to LINQ might seem daunting at first, but with careful attention to detail, it can be done easily. Always remember to ensure that your syntax aligns with LINQ conventions, use methods like Any for existence checks, and defer execution until the final result is needed.
By following the outlined steps, you can effectively transform your SQL queries into LINQ expressions in your C- Web APIs or any other .NET applications. Happy coding!