filmov
tv
Resolving the Cannot delete python object instance Error: Understanding Global Variables in Python
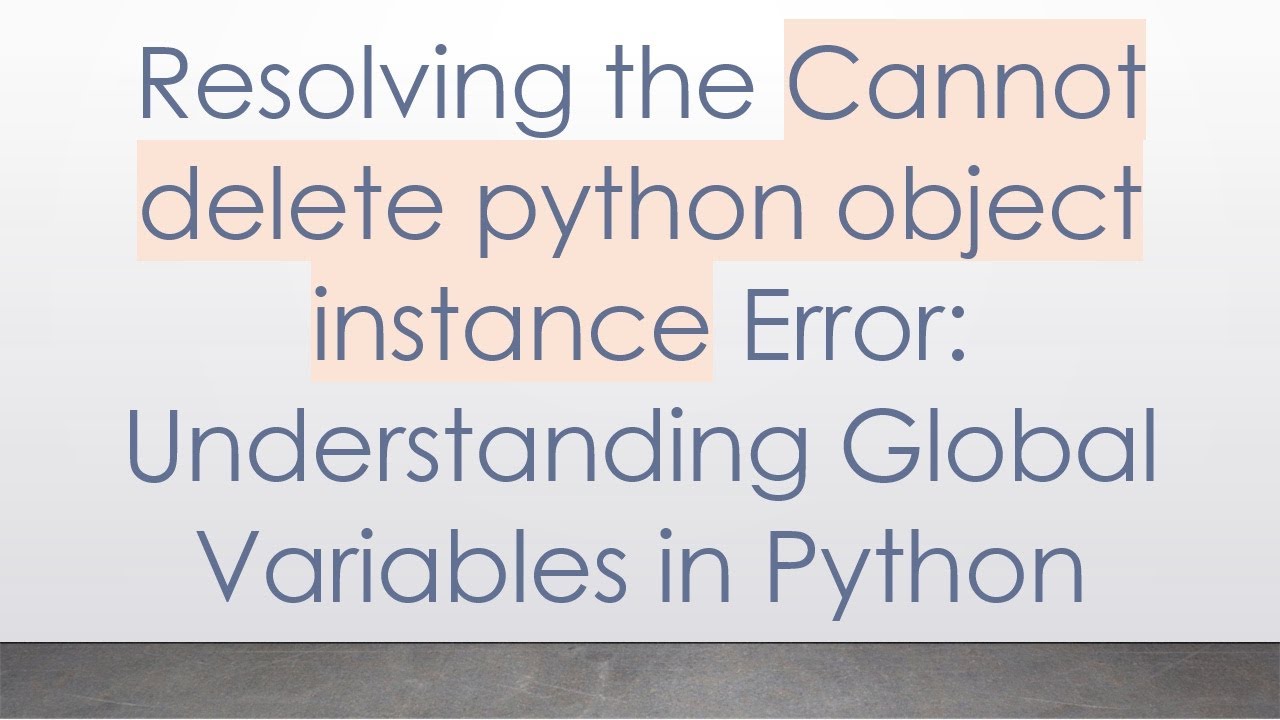
Показать описание
Learn how to effectively manage object instances in Python by understanding global variable scoping and best practices in this informative guide.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Cannot delete python object instance
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Resolving the Cannot delete python object instance Error: Understanding Global Variables in Python
Python is a powerful programming language, but like any language, it has its quirks that can cause confusion for developers, especially beginners. One common issue is the inability to delete an object instance, which can lead to errors like local variable 'object' referenced before assignment. This guide will help you understand this problem and offer a clear solution to manage object instances effectively using global variables.
The Problem: Understanding the Error
You may encounter the following situation in your Python code:
[[See Video to Reveal this Text or Code Snippet]]
This snippet is meant to create an instance of a class A and later delete that instance. However, upon executing the stop() function, you encounter the error local variable 'object' referenced before assignment. This indicates that Python is attempting to treat object as a local variable rather than a global one.
What Causes This Error?
The issue arises because of two main factors:
Naming Conflict: The use of the name object is problematic, as it is a built-in keyword in Python. Using it can lead to unpredictable behavior and errors.
Variable Scope: You must declare a variable global within a function before you can modify it. If you try to use it without a prior declaration, Python assumes it's a new, local variable.
The Solution: Proper Handling of Global Variables
To effectively manage global variables in Python, follow these steps:
1. Avoid Using Keywords
Firstly, never use keywords like object as variable names. This practice can lead to confusion and errors in your code. Instead, choose a more descriptive name for your object, like obj.
2. Declare Global Variables Correctly
You should redeclare your global variable in every function where you intend to modify it. Here’s how to properly write your start and stop functions:
[[See Video to Reveal this Text or Code Snippet]]
3. Example Implementation
To illustrate further, consider this complete example:
[[See Video to Reveal this Text or Code Snippet]]
4. Considerations for Best Practices
While using global variables can work, relying on them is generally considered bad practice in Python. When possible, consider refactoring your code to avoid globals, such as using class instances, returning values, or encapsulating function behaviors within classes.
Conclusion
Deleting an object instance in Python when leveraging global variables isn't as straightforward as one might hope due to variable scopes and naming conventions. By avoiding keywords for variable names, properly declaring global variables within functions, and understanding best practices, you can effectively manage your object instances without running into common traps and errors.
By following the guidance provided in this post, you should be able to resolve the error and write cleaner, more efficient Python code. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Cannot delete python object instance
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Resolving the Cannot delete python object instance Error: Understanding Global Variables in Python
Python is a powerful programming language, but like any language, it has its quirks that can cause confusion for developers, especially beginners. One common issue is the inability to delete an object instance, which can lead to errors like local variable 'object' referenced before assignment. This guide will help you understand this problem and offer a clear solution to manage object instances effectively using global variables.
The Problem: Understanding the Error
You may encounter the following situation in your Python code:
[[See Video to Reveal this Text or Code Snippet]]
This snippet is meant to create an instance of a class A and later delete that instance. However, upon executing the stop() function, you encounter the error local variable 'object' referenced before assignment. This indicates that Python is attempting to treat object as a local variable rather than a global one.
What Causes This Error?
The issue arises because of two main factors:
Naming Conflict: The use of the name object is problematic, as it is a built-in keyword in Python. Using it can lead to unpredictable behavior and errors.
Variable Scope: You must declare a variable global within a function before you can modify it. If you try to use it without a prior declaration, Python assumes it's a new, local variable.
The Solution: Proper Handling of Global Variables
To effectively manage global variables in Python, follow these steps:
1. Avoid Using Keywords
Firstly, never use keywords like object as variable names. This practice can lead to confusion and errors in your code. Instead, choose a more descriptive name for your object, like obj.
2. Declare Global Variables Correctly
You should redeclare your global variable in every function where you intend to modify it. Here’s how to properly write your start and stop functions:
[[See Video to Reveal this Text or Code Snippet]]
3. Example Implementation
To illustrate further, consider this complete example:
[[See Video to Reveal this Text or Code Snippet]]
4. Considerations for Best Practices
While using global variables can work, relying on them is generally considered bad practice in Python. When possible, consider refactoring your code to avoid globals, such as using class instances, returning values, or encapsulating function behaviors within classes.
Conclusion
Deleting an object instance in Python when leveraging global variables isn't as straightforward as one might hope due to variable scopes and naming conventions. By avoiding keywords for variable names, properly declaring global variables within functions, and understanding best practices, you can effectively manage your object instances without running into common traps and errors.
By following the guidance provided in this post, you should be able to resolve the error and write cleaner, more efficient Python code. Happy coding!