filmov
tv
Implementing a 5-Second Lock on Row Updates in SQLite3 with Python
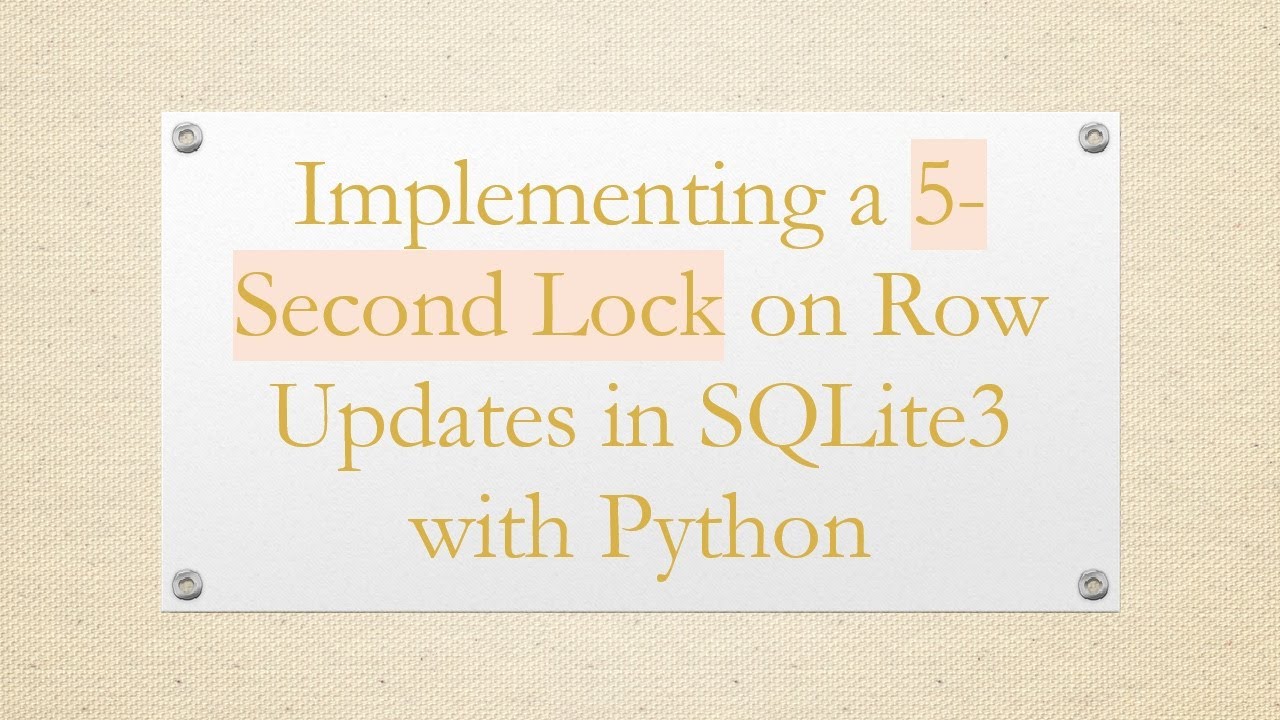
Показать описание
Learn how to prevent immediate updates on specific rows in SQLite3 using Python by implementing a `5-second lock`. Enhance data integrity and avoid conflicting changes with this simple method.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Is it possible in SQlite3 python that if a row has been previously updated, it cannot be updated for like 5 seconds
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Implementing a 5-Second Lock on Row Updates in SQLite3 with Python
In the realm of database management, ensuring data integrity is crucial. A common challenge arises when multiple operations want to update the same record simultaneously. Have you ever faced a scenario where a row in your SQLite3 database needs a brief pause before it can be updated again? For example, a user might attempt to update an order record multiple times in quick succession, leading to potential inconsistencies. This guide addresses how you can implement a mechanism in Python that enforces a 5-second lock on updates to a row in SQLite3.
The Problem
You want to ensure that once a row in your SQLite3 database is updated, it cannot be modified again for a short period—let's say, 5 seconds. This requirement protects the data from being overwritten accidentally or maliciously. But how do you implement this effectively?
The Solution
Yes, it’s entirely possible to achieve this behavior in SQLite3. The essential steps involve:
Adding a last_modified Column: This column will track the last time a row was updated.
Creating Functions to Check and Update Rows: Functions will ensure that rows can only be updated if a specified time interval has passed since the last modification.
Step 1: Modify Your Database Structure
First, you need to create or modify your SQLite table to include the last_modified column, which stores the timestamp of the last update.
[[See Video to Reveal this Text or Code Snippet]]
If your table is already created, you can add the column using:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Implement the Logic in Python
Now it’s time to write the Python code that will check whether a row can be updated. Below is a conceptual implementation using SQLite3 within Python:
[[See Video to Reveal this Text or Code Snippet]]
Code Explanation
Database Connection: Establishes a connection to your SQLite database and creates a cursor to interact with it.
Checking Function (can_modify_row): This function checks how long ago the row was updated. If it's more than the specified interval (e.g., 5 seconds), it returns True.
Updating Function (update_row): When applicable, this function updates the specified row and sets the last_modified column to the current timestamp.
Conclusion
Implementing a 5-second lock mechanism on row updates in SQLite3 with Python is straightforward with the right approach. By introducing a last_modified timestamp, you can control and manage how frequently rows are updated, thus protecting your data from inconsistencies. While the provided code hasn't been tested, it serves as a robust foundation for your own implementation. Feel free to modify and test it according to your needs!
With these steps, you can ensure that your SQLite database operates smoothly, with minimal risk of overlapping updates. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Is it possible in SQlite3 python that if a row has been previously updated, it cannot be updated for like 5 seconds
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Implementing a 5-Second Lock on Row Updates in SQLite3 with Python
In the realm of database management, ensuring data integrity is crucial. A common challenge arises when multiple operations want to update the same record simultaneously. Have you ever faced a scenario where a row in your SQLite3 database needs a brief pause before it can be updated again? For example, a user might attempt to update an order record multiple times in quick succession, leading to potential inconsistencies. This guide addresses how you can implement a mechanism in Python that enforces a 5-second lock on updates to a row in SQLite3.
The Problem
You want to ensure that once a row in your SQLite3 database is updated, it cannot be modified again for a short period—let's say, 5 seconds. This requirement protects the data from being overwritten accidentally or maliciously. But how do you implement this effectively?
The Solution
Yes, it’s entirely possible to achieve this behavior in SQLite3. The essential steps involve:
Adding a last_modified Column: This column will track the last time a row was updated.
Creating Functions to Check and Update Rows: Functions will ensure that rows can only be updated if a specified time interval has passed since the last modification.
Step 1: Modify Your Database Structure
First, you need to create or modify your SQLite table to include the last_modified column, which stores the timestamp of the last update.
[[See Video to Reveal this Text or Code Snippet]]
If your table is already created, you can add the column using:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Implement the Logic in Python
Now it’s time to write the Python code that will check whether a row can be updated. Below is a conceptual implementation using SQLite3 within Python:
[[See Video to Reveal this Text or Code Snippet]]
Code Explanation
Database Connection: Establishes a connection to your SQLite database and creates a cursor to interact with it.
Checking Function (can_modify_row): This function checks how long ago the row was updated. If it's more than the specified interval (e.g., 5 seconds), it returns True.
Updating Function (update_row): When applicable, this function updates the specified row and sets the last_modified column to the current timestamp.
Conclusion
Implementing a 5-second lock mechanism on row updates in SQLite3 with Python is straightforward with the right approach. By introducing a last_modified timestamp, you can control and manage how frequently rows are updated, thus protecting your data from inconsistencies. While the provided code hasn't been tested, it serves as a robust foundation for your own implementation. Feel free to modify and test it according to your needs!
With these steps, you can ensure that your SQLite database operates smoothly, with minimal risk of overlapping updates. Happy coding!