filmov
tv
Mastering Python: How to Reverse the Linked List Using Recursion
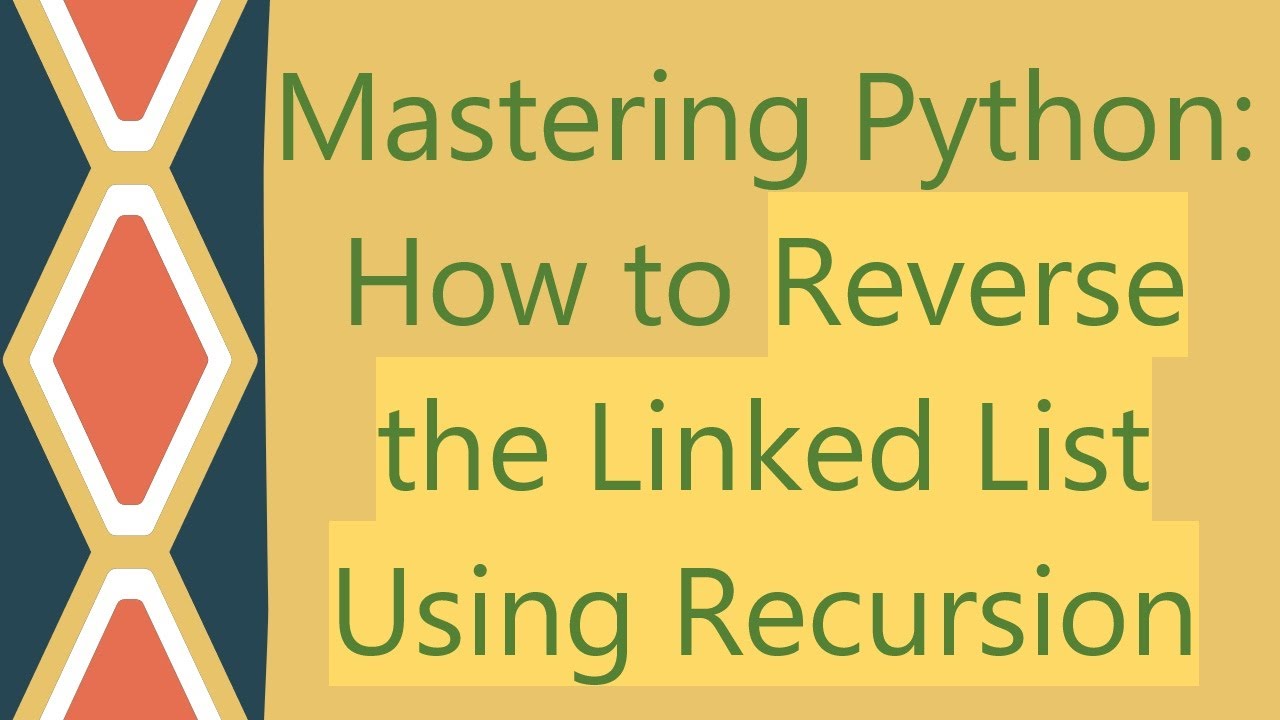
Показать описание
Summary: Learn how to reverse a linked list using recursion in Python with step-by-step instructions and sample code to enhance your programming skills.
---
Mastering Python: How to Reverse the Linked List Using Recursion
Reversing a linked list is a classic problem in computer science that often comes up during technical interviews. While there are several ways to approach this problem, using recursion can provide an elegant and efficient solution. In this guide, we'll guide you through the process of reversing a linked list recursively in Python.
Understanding the Linked List
Before diving into the recursive solution, let's briefly revisit what a linked list is. A linked list is a linear data structure where each element, called a node, contains a value and a reference (or link) to the next node in the sequence. The primary advantage of linked lists over arrays is their dynamic size and ease of insertion or deletion of elements.
Here's a simple class definition for a singly linked list node:
[[See Video to Reveal this Text or Code Snippet]]
The Recursive Approach
To reverse a linked list recursively, you'll need to think in smaller subproblems. Each recursive call will reverse a smaller portion of the list until the entire list is reversed.
The Base Case:
Breaking the Problem Down:
Save the reference to the next node.
Make the next node call the reverse function recursively.
Set the subsequent node’s next to the current node.
Set the current node’s next to None.
Here's how you can implement this in Python:
[[See Video to Reveal this Text or Code Snippet]]
Explanation
Base Case: If the linked list is empty or has just one node, simply return the head as it is already reversed.
Adjust Pointers: Once the sublist is reversed, adjust the pointers:
Testing the Function
To test the recursive function, you can create a sample linked list and print its elements before and after reversing:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Reversing a linked list using recursion in Python is not only an excellent way to brush up on your recursion skills but also provides an elegant solution to a common problem. This approach divides the task into smaller subproblems, making the overall process easier to understand and implement. By mastering this technique, you'll be better prepared for technical interviews and ready to tackle more complex problems involving linked lists.
Happy coding!
---
Mastering Python: How to Reverse the Linked List Using Recursion
Reversing a linked list is a classic problem in computer science that often comes up during technical interviews. While there are several ways to approach this problem, using recursion can provide an elegant and efficient solution. In this guide, we'll guide you through the process of reversing a linked list recursively in Python.
Understanding the Linked List
Before diving into the recursive solution, let's briefly revisit what a linked list is. A linked list is a linear data structure where each element, called a node, contains a value and a reference (or link) to the next node in the sequence. The primary advantage of linked lists over arrays is their dynamic size and ease of insertion or deletion of elements.
Here's a simple class definition for a singly linked list node:
[[See Video to Reveal this Text or Code Snippet]]
The Recursive Approach
To reverse a linked list recursively, you'll need to think in smaller subproblems. Each recursive call will reverse a smaller portion of the list until the entire list is reversed.
The Base Case:
Breaking the Problem Down:
Save the reference to the next node.
Make the next node call the reverse function recursively.
Set the subsequent node’s next to the current node.
Set the current node’s next to None.
Here's how you can implement this in Python:
[[See Video to Reveal this Text or Code Snippet]]
Explanation
Base Case: If the linked list is empty or has just one node, simply return the head as it is already reversed.
Adjust Pointers: Once the sublist is reversed, adjust the pointers:
Testing the Function
To test the recursive function, you can create a sample linked list and print its elements before and after reversing:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Reversing a linked list using recursion in Python is not only an excellent way to brush up on your recursion skills but also provides an elegant solution to a common problem. This approach divides the task into smaller subproblems, making the overall process easier to understand and implement. By mastering this technique, you'll be better prepared for technical interviews and ready to tackle more complex problems involving linked lists.
Happy coding!