filmov
tv
How to Count Occurrences of an Element in an Array Using JavaScript
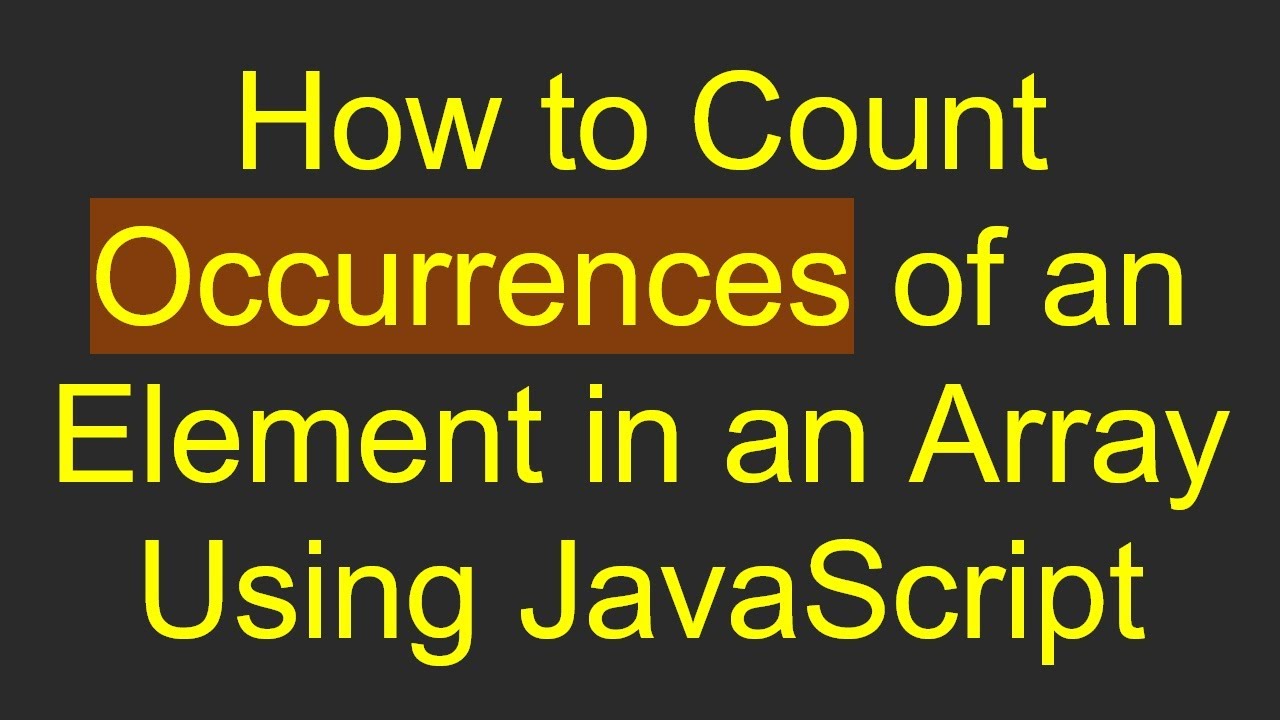
Показать описание
Learn how to effortlessly count the `occurrences` of a specific element in a JavaScript array with this simple guide.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Function that counts the occurrences of an element in an array
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Problem: Counting Occurrences in an Array
Have you ever faced a scenario where you needed to find out how many times a certain element appears in an array? This is a common problem in programming, and knowing how to tackle it can save you a lot of time and effort.
For example, consider the array [5, 7, 12, 5, 3, 3, 5]. If you want to find out how many times the number 3 appears in this array, you would need to write a function that can effectively count its occurrences. In this guide, we will walk through a simple solution implemented in JavaScript.
The Solution: Using Array Methods
To count the occurrences of an element in an array, we can leverage JavaScript's powerful array methods. Specifically, we'll use the filter method combined with the length property. Here's a breakdown of how it works:
Step-by-Step Explanation
Filter the Array: The filter method allows you to create a new array filled with elements that pass a test provided by a function. In this case, we will test if each element is equal to the element we're counting.
Get the Length: After filtering, we can get the number of elements in the new array using the length property. This final count will represent the number of occurrences of the specified element.
The Code Implementation
Let's take a look at the JavaScript code that accomplishes this:
[[See Video to Reveal this Text or Code Snippet]]
Breaking Down the Code
Array Definition: We start by defining our array arr which contains the elements we want to analyze.
Function Definition: The occurrences function takes two parameters: the array to search and the element (el) to count.
Invoking the Function: Finally, we call the function with arr and 3 to count how many times 3 appears. The result is logged to the console.
Conclusion
Counting the occurrences of an element in an array is straightforward when you use the right tools. With JavaScript's filter method and the length property, you can quickly determine how many times a specific value appears. This technique is not just useful for the example we've discussed but can also be applied in a variety of programming scenarios.
Next time you need to count occurrences in an array, you’ll know just what to do!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Function that counts the occurrences of an element in an array
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Problem: Counting Occurrences in an Array
Have you ever faced a scenario where you needed to find out how many times a certain element appears in an array? This is a common problem in programming, and knowing how to tackle it can save you a lot of time and effort.
For example, consider the array [5, 7, 12, 5, 3, 3, 5]. If you want to find out how many times the number 3 appears in this array, you would need to write a function that can effectively count its occurrences. In this guide, we will walk through a simple solution implemented in JavaScript.
The Solution: Using Array Methods
To count the occurrences of an element in an array, we can leverage JavaScript's powerful array methods. Specifically, we'll use the filter method combined with the length property. Here's a breakdown of how it works:
Step-by-Step Explanation
Filter the Array: The filter method allows you to create a new array filled with elements that pass a test provided by a function. In this case, we will test if each element is equal to the element we're counting.
Get the Length: After filtering, we can get the number of elements in the new array using the length property. This final count will represent the number of occurrences of the specified element.
The Code Implementation
Let's take a look at the JavaScript code that accomplishes this:
[[See Video to Reveal this Text or Code Snippet]]
Breaking Down the Code
Array Definition: We start by defining our array arr which contains the elements we want to analyze.
Function Definition: The occurrences function takes two parameters: the array to search and the element (el) to count.
Invoking the Function: Finally, we call the function with arr and 3 to count how many times 3 appears. The result is logged to the console.
Conclusion
Counting the occurrences of an element in an array is straightforward when you use the right tools. With JavaScript's filter method and the length property, you can quickly determine how many times a specific value appears. This technique is not just useful for the example we've discussed but can also be applied in a variety of programming scenarios.
Next time you need to count occurrences in an array, you’ll know just what to do!