filmov
tv
Transform Dataframe Columns into a Nested Dictionary in Python with Pandas
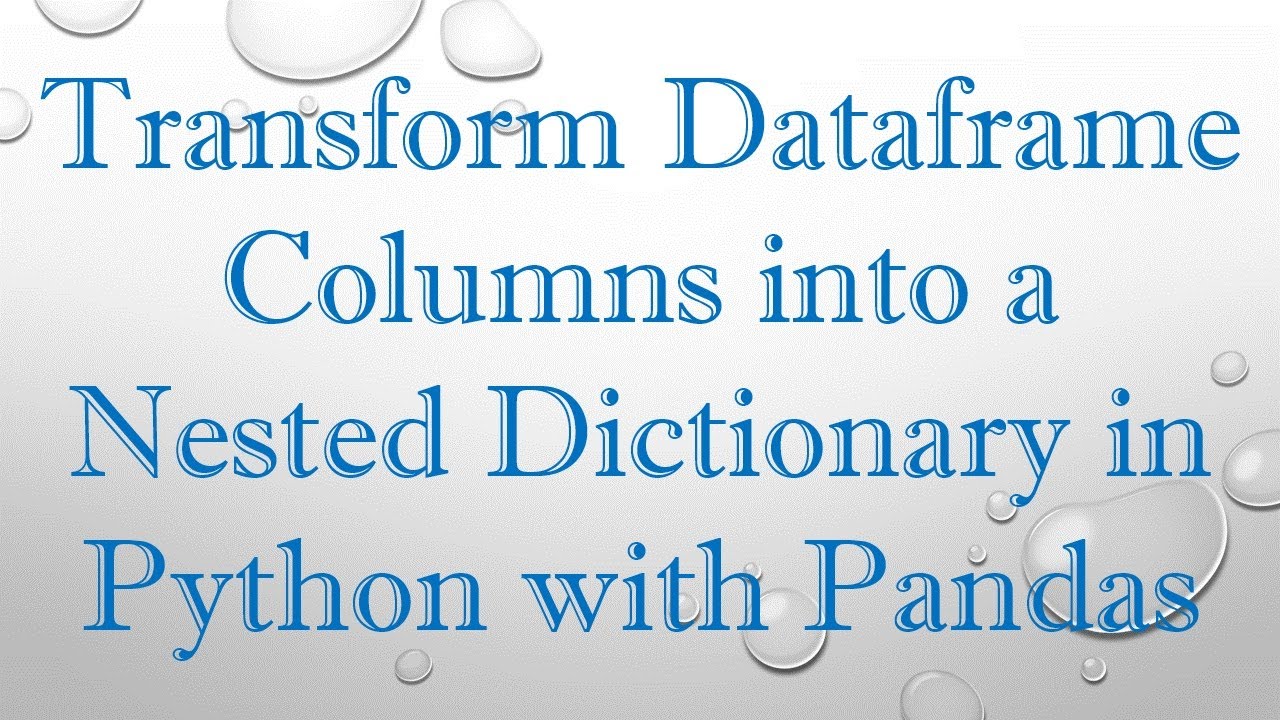
Показать описание
Learn how to convert DataFrame columns containing specific strings into a nested dictionary using Pandas in Python. This step-by-step guide simplifies the process for beginners.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to convert dataframe columns which contains specific string to each columns to a nested dictionary?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Transforming Dataframe Columns into a Nested Dictionary Using Pandas
When working with data in Python, particularly with the Pandas library, you may encounter situations where column names contain related information that you'd like to organize better. A common scenario involves having columns named with suffixes—like _avg and _std—that denote summary statistics for a given variable. You might want to transform these columns into a neat nested dictionary, making your data easier to work with.
In this guide, we will explore how to convert a DataFrame containing such columns into a nested dictionary. For instance, if you have a DataFrame with columns like subscription_id_avg, subscription_id_std, etc., we'll show you how to represent this information as:
[[See Video to Reveal this Text or Code Snippet]]
The Problem at Hand
Suppose you have a DataFrame with approximately 84 columns, each representing a different statistic related to some variable, like a subscription ID. The columns follow a consistent naming pattern, where the last four characters of the column names are the suffixes we are interested in (e.g., _avg, _std). Our goal is to transform this DataFrame into a concise nested dictionary format.
Understanding the Solution
To achieve the desired nested dictionary from the DataFrame, we will use a simple Python loop and some string manipulation techniques. Here’s how to break down the solution:
Step 1: Initialize an Empty Dictionary
We start by creating an empty dictionary that will eventually hold our nested structure.
Step 2: Iterating Through Column Names
We will loop through the names of the DataFrame's columns and extract the relevant parts of the names to build our nested structure. The key steps are:
Extracting the Base Name: By slicing the string, we can remove the last four characters to obtain the base name (e.g., from subscription_id_avg to subscription_id).
Determining the Suffix: The last three characters will help denote whether it’s the average or standard deviation (avg or std).
Step 3: Building the Nested Dictionary
In this step, we will check if the base name already exists in our dictionary. If it does not exist, we will create a new entry. If it does exist, we will add the new statistic to the existing base entry. Here’s the code that accomplishes these steps:
[[See Video to Reveal this Text or Code Snippet]]
Final Considerations
This method will work effectively if _avg and _std are the only suffixes present in the column names. If there are additional suffixes or variations, you may need to expand the logic accordingly.
Conclusion
By following the above steps, you can efficiently convert DataFrame columns into a well-structured nested dictionary, making your data even more manageable and insightful. Utilizing such structures can often result in clearer data handling and enhanced data manipulation efforts in your Python projects.
I hope this guide helps you streamline your data transformation process! Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to convert dataframe columns which contains specific string to each columns to a nested dictionary?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Transforming Dataframe Columns into a Nested Dictionary Using Pandas
When working with data in Python, particularly with the Pandas library, you may encounter situations where column names contain related information that you'd like to organize better. A common scenario involves having columns named with suffixes—like _avg and _std—that denote summary statistics for a given variable. You might want to transform these columns into a neat nested dictionary, making your data easier to work with.
In this guide, we will explore how to convert a DataFrame containing such columns into a nested dictionary. For instance, if you have a DataFrame with columns like subscription_id_avg, subscription_id_std, etc., we'll show you how to represent this information as:
[[See Video to Reveal this Text or Code Snippet]]
The Problem at Hand
Suppose you have a DataFrame with approximately 84 columns, each representing a different statistic related to some variable, like a subscription ID. The columns follow a consistent naming pattern, where the last four characters of the column names are the suffixes we are interested in (e.g., _avg, _std). Our goal is to transform this DataFrame into a concise nested dictionary format.
Understanding the Solution
To achieve the desired nested dictionary from the DataFrame, we will use a simple Python loop and some string manipulation techniques. Here’s how to break down the solution:
Step 1: Initialize an Empty Dictionary
We start by creating an empty dictionary that will eventually hold our nested structure.
Step 2: Iterating Through Column Names
We will loop through the names of the DataFrame's columns and extract the relevant parts of the names to build our nested structure. The key steps are:
Extracting the Base Name: By slicing the string, we can remove the last four characters to obtain the base name (e.g., from subscription_id_avg to subscription_id).
Determining the Suffix: The last three characters will help denote whether it’s the average or standard deviation (avg or std).
Step 3: Building the Nested Dictionary
In this step, we will check if the base name already exists in our dictionary. If it does not exist, we will create a new entry. If it does exist, we will add the new statistic to the existing base entry. Here’s the code that accomplishes these steps:
[[See Video to Reveal this Text or Code Snippet]]
Final Considerations
This method will work effectively if _avg and _std are the only suffixes present in the column names. If there are additional suffixes or variations, you may need to expand the logic accordingly.
Conclusion
By following the above steps, you can efficiently convert DataFrame columns into a well-structured nested dictionary, making your data even more manageable and insightful. Utilizing such structures can often result in clearer data handling and enhanced data manipulation efforts in your Python projects.
I hope this guide helps you streamline your data transformation process! Happy coding!