filmov
tv
Understanding Performance Differences: PHP8 Reflection vs. PHP7 Without Reflection
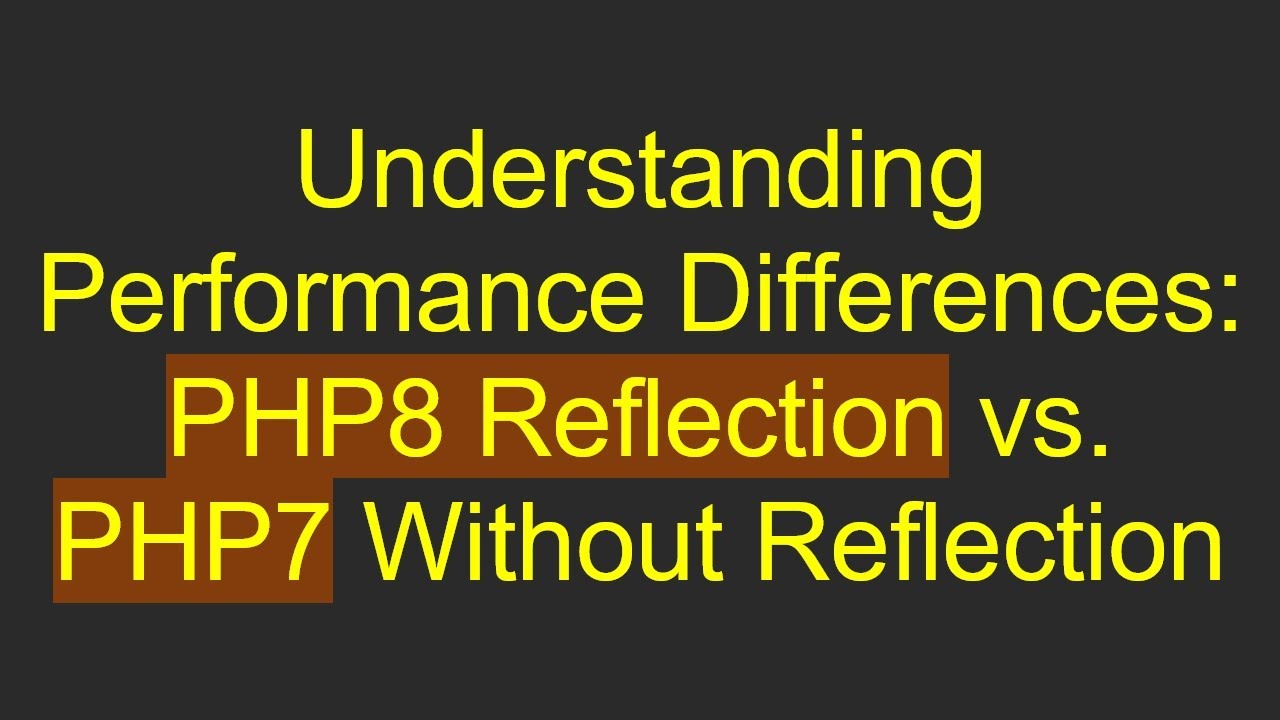
Показать описание
Explore how using `Reflection` in PHP8 can affect performance compared to PHP7, and learn how to optimize your code for better efficiency.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Does PHP8 Reflection more bad performance versus PHP7 without reflection
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Performance Differences: PHP8 Reflection vs. PHP7 Without Reflection
When transitioning code from PHP7 to PHP8, developers often encounter unexpected performance issues. One common concern is the slowdown that may occur when replacing straightforward property access with reflection-based mechanisms. Here, we will dive deep into the performance implications of using reflection in PHP8 and offer strategies for optimizing your code to maintain efficiency.
The Problem
After updating a codebase from PHP 7.4 to PHP 8.2, a developer experienced noticeable performance degradation in their model serialization methods. In the previous version, they used a simple toArray() method directly accessing class properties. The new approach employed reflection to serialize objects, leading to concerns about whether this shift has caused the slowdown.
Example of the Old and New Approaches
Old Approach (PHP7.4)
[[See Video to Reveal this Text or Code Snippet]]
New Approach (PHP8)
Using Reflection:
[[See Video to Reveal this Text or Code Snippet]]
Analyzing the Shift: PHP8 Reflection vs. PHP7 Direct Access
Direct Access vs. Getter Methods
Transitioning from direct property access to methods:
Direct Property Access: Accessing properties directly is usually faster.
Getter Methods: Introducing getter methods can add overhead since it requires a method call, which is inherently slower.
Reflection Overhead
Using reflection introduces additional overhead:
Reflection Operations: These operations are significantly more expensive than direct property access. However, they can often be cached efficiently.
Now, let's break this down further to understand how we can optimize the use of reflection while minimizing the performance hit.
Optimizing Reflection Usage
To enhance the performance of your reflection-based approach, consider implementing caching. Caching avoids redundant reflection calls, significantly improving the speed of repeated data access.
Suggested Implementation
Here's how you can optimize the use of reflection:
Implement Caching: Cache the results of the reflection operations so that subsequent calls can quickly access pre-computed values.
[[See Video to Reveal this Text or Code Snippet]]
Check the Cache: Before executing reflection, check if the properties/methods have already been cached. If they have, utilize the cached values instead.
[[See Video to Reveal this Text or Code Snippet]]
Measure Performance: Include benchmarking in your code to monitor the runtime of various techniques:
[[See Video to Reveal this Text or Code Snippet]]
Sample Code with Caching
Here is a modified version of the jsonSerialize() function that implements caching:
[[See Video to Reveal this Text or Code Snippet]]
Testing Performance Variations
The following code snippet allows you to assess performance differences between reflection and direct access:
[[See Video to Reveal this Text or Code Snippet]]
By conducting these tests, you can quantitatively determine the impact of each method and adjust your code accordingly.
Conclusion
Transitioning from PHP7 to PHP8 and implementing reflection-based property access can indeed lead to performance implications. However, by introducing caching mechanisms and being strategic about your access methods, you can mitigate potential slowdowns. If you're handling large volumes of data, optimizing your reflection logic is crucial to maintain high performance.
Make sure to always test and profile your code, as the right approach will depend heavily on your specific application and data structures.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Does PHP8 Reflection more bad performance versus PHP7 without reflection
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Performance Differences: PHP8 Reflection vs. PHP7 Without Reflection
When transitioning code from PHP7 to PHP8, developers often encounter unexpected performance issues. One common concern is the slowdown that may occur when replacing straightforward property access with reflection-based mechanisms. Here, we will dive deep into the performance implications of using reflection in PHP8 and offer strategies for optimizing your code to maintain efficiency.
The Problem
After updating a codebase from PHP 7.4 to PHP 8.2, a developer experienced noticeable performance degradation in their model serialization methods. In the previous version, they used a simple toArray() method directly accessing class properties. The new approach employed reflection to serialize objects, leading to concerns about whether this shift has caused the slowdown.
Example of the Old and New Approaches
Old Approach (PHP7.4)
[[See Video to Reveal this Text or Code Snippet]]
New Approach (PHP8)
Using Reflection:
[[See Video to Reveal this Text or Code Snippet]]
Analyzing the Shift: PHP8 Reflection vs. PHP7 Direct Access
Direct Access vs. Getter Methods
Transitioning from direct property access to methods:
Direct Property Access: Accessing properties directly is usually faster.
Getter Methods: Introducing getter methods can add overhead since it requires a method call, which is inherently slower.
Reflection Overhead
Using reflection introduces additional overhead:
Reflection Operations: These operations are significantly more expensive than direct property access. However, they can often be cached efficiently.
Now, let's break this down further to understand how we can optimize the use of reflection while minimizing the performance hit.
Optimizing Reflection Usage
To enhance the performance of your reflection-based approach, consider implementing caching. Caching avoids redundant reflection calls, significantly improving the speed of repeated data access.
Suggested Implementation
Here's how you can optimize the use of reflection:
Implement Caching: Cache the results of the reflection operations so that subsequent calls can quickly access pre-computed values.
[[See Video to Reveal this Text or Code Snippet]]
Check the Cache: Before executing reflection, check if the properties/methods have already been cached. If they have, utilize the cached values instead.
[[See Video to Reveal this Text or Code Snippet]]
Measure Performance: Include benchmarking in your code to monitor the runtime of various techniques:
[[See Video to Reveal this Text or Code Snippet]]
Sample Code with Caching
Here is a modified version of the jsonSerialize() function that implements caching:
[[See Video to Reveal this Text or Code Snippet]]
Testing Performance Variations
The following code snippet allows you to assess performance differences between reflection and direct access:
[[See Video to Reveal this Text or Code Snippet]]
By conducting these tests, you can quantitatively determine the impact of each method and adjust your code accordingly.
Conclusion
Transitioning from PHP7 to PHP8 and implementing reflection-based property access can indeed lead to performance implications. However, by introducing caching mechanisms and being strategic about your access methods, you can mitigate potential slowdowns. If you're handling large volumes of data, optimizing your reflection logic is crucial to maintain high performance.
Make sure to always test and profile your code, as the right approach will depend heavily on your specific application and data structures.