filmov
tv
Efficiently Modify Array Elements in Java with Streams and Lambdas
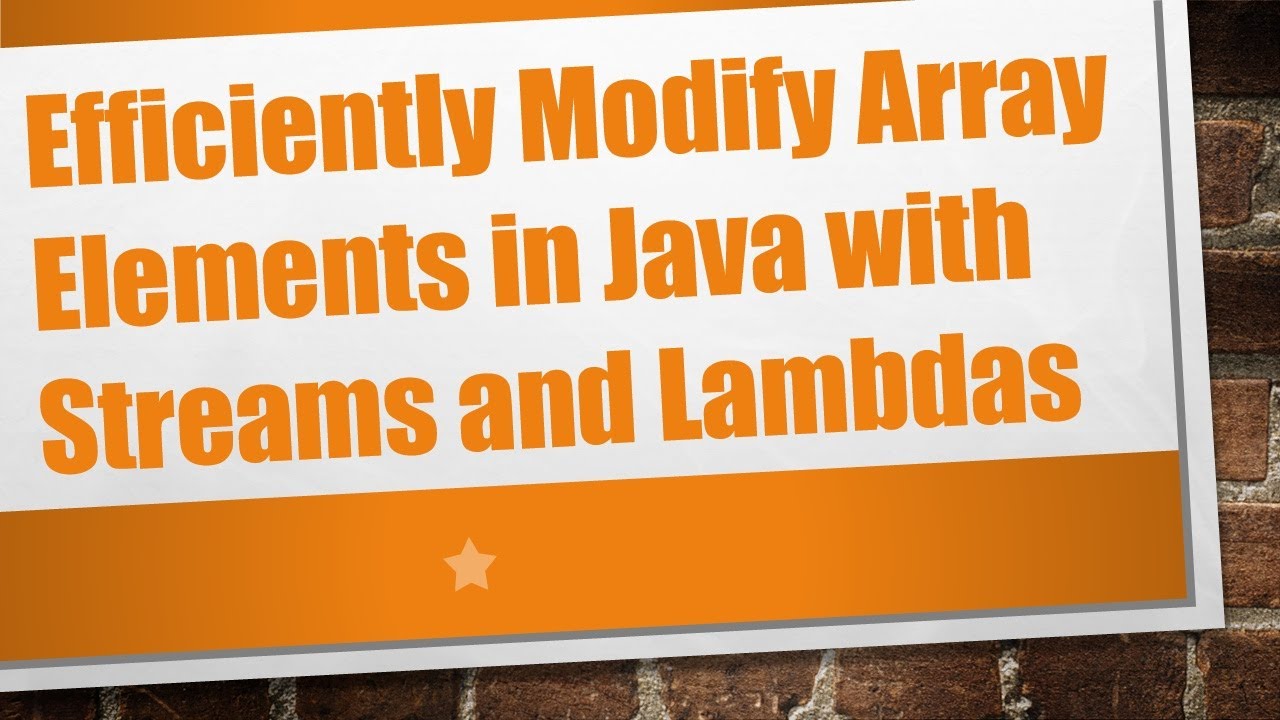
Показать описание
Explore how to change array elements using modern Java methods without unnecessary conversions by leveraging streams and lambdas in your code.
---
Visit these links for original content and any more details, such as alternate solutions, comments, revision history etc. For example, the original title of the Question was: Change array elements with a stream without converting its collection
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Easily Modifying Array Elements in Java with Streams and Lambdas
Java has evolved significantly over the years, especially with the introduction of Streams and Lambda expressions in Java 8. However, many developers still find themselves using traditional methods to manipulate data structures like arrays. This raises an important question: How can you modify array elements in Java using streams without the hassle of converting to a list and back to an array?
In this guide, we'll explore a simple yet effective solution that fits within the modern Java paradigm.
The Problem at Hand
In the provided code snippet, a standard loop is used to append a string to each element of an array:
[[See Video to Reveal this Text or Code Snippet]]
While this approach does the job, many developers cringe at the idea of moving away from cleaner, more expressive coding styles offered by lambdas and streams. A common alternative might look like this:
[[See Video to Reveal this Text or Code Snippet]]
This method appears more modern but requires unnecessary conversions between different collections, which can feel cumbersome and inefficient.
The Modern Solution
Here’s the Improved Code:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Solution
1. Stream Creation:
2. Mapping Elements:
The map() function takes each element (denoted by a) from the stream and modifies it by appending the string "_abc".
3. Collecting Results:
Finally, toArray(String[]::new) converts the stream back into a new array of the same type, allowing us to pass it to the desired function (bar(args)).
Benefits of This Approach
Simplicity: The code remains straightforward and concise, preserving clarity.
Efficiency: Since it avoids multiple conversions between collections, it performs better, especially with larger datasets.
Modern Java Practices: Using streams and lambdas enhances the readability and elegance of your codebase.
Conclusion
By leveraging streams and lambda expressions, you can modify array elements in Java without falling into the trap of unnecessary conversions. The solution outlined above provides a clean way to enhance your code while maintaining high performance. Embracing such modern practices not only makes your code more efficient but also aligns with current development trends in the Java ecosystem.
Make the switch today and elevate your Java programming skills!
---
Visit these links for original content and any more details, such as alternate solutions, comments, revision history etc. For example, the original title of the Question was: Change array elements with a stream without converting its collection
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Easily Modifying Array Elements in Java with Streams and Lambdas
Java has evolved significantly over the years, especially with the introduction of Streams and Lambda expressions in Java 8. However, many developers still find themselves using traditional methods to manipulate data structures like arrays. This raises an important question: How can you modify array elements in Java using streams without the hassle of converting to a list and back to an array?
In this guide, we'll explore a simple yet effective solution that fits within the modern Java paradigm.
The Problem at Hand
In the provided code snippet, a standard loop is used to append a string to each element of an array:
[[See Video to Reveal this Text or Code Snippet]]
While this approach does the job, many developers cringe at the idea of moving away from cleaner, more expressive coding styles offered by lambdas and streams. A common alternative might look like this:
[[See Video to Reveal this Text or Code Snippet]]
This method appears more modern but requires unnecessary conversions between different collections, which can feel cumbersome and inefficient.
The Modern Solution
Here’s the Improved Code:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Solution
1. Stream Creation:
2. Mapping Elements:
The map() function takes each element (denoted by a) from the stream and modifies it by appending the string "_abc".
3. Collecting Results:
Finally, toArray(String[]::new) converts the stream back into a new array of the same type, allowing us to pass it to the desired function (bar(args)).
Benefits of This Approach
Simplicity: The code remains straightforward and concise, preserving clarity.
Efficiency: Since it avoids multiple conversions between collections, it performs better, especially with larger datasets.
Modern Java Practices: Using streams and lambdas enhances the readability and elegance of your codebase.
Conclusion
By leveraging streams and lambda expressions, you can modify array elements in Java without falling into the trap of unnecessary conversions. The solution outlined above provides a clean way to enhance your code while maintaining high performance. Embracing such modern practices not only makes your code more efficient but also aligns with current development trends in the Java ecosystem.
Make the switch today and elevate your Java programming skills!