filmov
tv
How to Use zip() for Element-wise Division in Python Lists
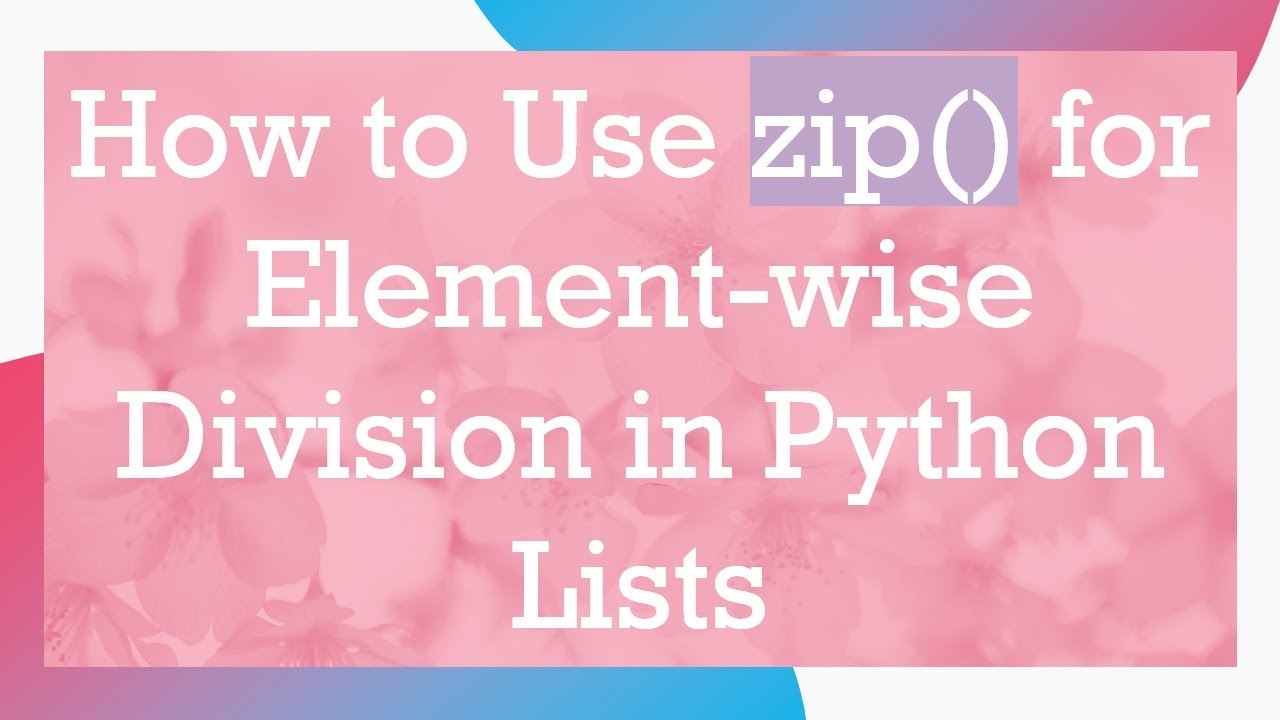
Показать описание
Learn how to efficiently divide elements from two lists using `zip()` and index-based methods in Python.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How do I divide by the corresponding element in the list while using zip()
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Element-wise Division with zip() in Python
In the world of data processing and manipulation with Python, there are numerous scenarios where we need to combine multiple lists and perform operations on the corresponding elements. One common task is performing calculations that require element-wise operations, especially when calculating means or averages. A particular user encountered an issue trying to calculate (i1 + i2) / [i] using two lists. In this guide, we will explore the problem and provide a clear solution to achieve the desired outcome.
Problem Overview
The user's requirement was to take two lists of numbers, perform a multiplication based on corresponding elements, and then divide that product by the index of the elements before computing the mean. The user attempted to use the zip() function in Python to combine the lists but stumbled upon an error when trying to incorporate indices in his computation.
Solution Steps
Using enumerate() with zip()
One effective method to access the indices of elements in a list while also zipping them up is by using the enumerate() function. This way, we can iterate through the paired elements, capturing both the indices and the values simultaneously. Below is how to implement it:
[[See Video to Reveal this Text or Code Snippet]]
Iterating Over Indices
If you prefer to work directly with indices instead of using enumerate, you can also achieve the same result by iterating over the range of the minimum length of the two lists (to avoid index errors). Here’s how you can do it:
[[See Video to Reveal this Text or Code Snippet]]
Note: In this implementation, we included a conditional to avoid dividing by zero at index 0.
Simplified Approach When Lengths Are Equal
If we know that the two lists are of equal length, we can simplify the code without the checks for minimum length. Here’s how that looks:
[[See Video to Reveal this Text or Code Snippet]]
Again, we ensure that we don't divide by zero.
Conclusion
By using either the enumerate() function or iteration through indices, we can successfully perform element-wise operations on lists in Python without encountering errors. This approach not only makes our code simpler but also prevents potential pitfalls associated with list indexing. Whether you're processing numerical datasets or handling complex computations, understanding these methods will enhance your Python programming skills. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How do I divide by the corresponding element in the list while using zip()
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Element-wise Division with zip() in Python
In the world of data processing and manipulation with Python, there are numerous scenarios where we need to combine multiple lists and perform operations on the corresponding elements. One common task is performing calculations that require element-wise operations, especially when calculating means or averages. A particular user encountered an issue trying to calculate (i1 + i2) / [i] using two lists. In this guide, we will explore the problem and provide a clear solution to achieve the desired outcome.
Problem Overview
The user's requirement was to take two lists of numbers, perform a multiplication based on corresponding elements, and then divide that product by the index of the elements before computing the mean. The user attempted to use the zip() function in Python to combine the lists but stumbled upon an error when trying to incorporate indices in his computation.
Solution Steps
Using enumerate() with zip()
One effective method to access the indices of elements in a list while also zipping them up is by using the enumerate() function. This way, we can iterate through the paired elements, capturing both the indices and the values simultaneously. Below is how to implement it:
[[See Video to Reveal this Text or Code Snippet]]
Iterating Over Indices
If you prefer to work directly with indices instead of using enumerate, you can also achieve the same result by iterating over the range of the minimum length of the two lists (to avoid index errors). Here’s how you can do it:
[[See Video to Reveal this Text or Code Snippet]]
Note: In this implementation, we included a conditional to avoid dividing by zero at index 0.
Simplified Approach When Lengths Are Equal
If we know that the two lists are of equal length, we can simplify the code without the checks for minimum length. Here’s how that looks:
[[See Video to Reveal this Text or Code Snippet]]
Again, we ensure that we don't divide by zero.
Conclusion
By using either the enumerate() function or iteration through indices, we can successfully perform element-wise operations on lists in Python without encountering errors. This approach not only makes our code simpler but also prevents potential pitfalls associated with list indexing. Whether you're processing numerical datasets or handling complex computations, understanding these methods will enhance your Python programming skills. Happy coding!