filmov
tv
How to Remove Elements in jQuery Based on Next Element's Text
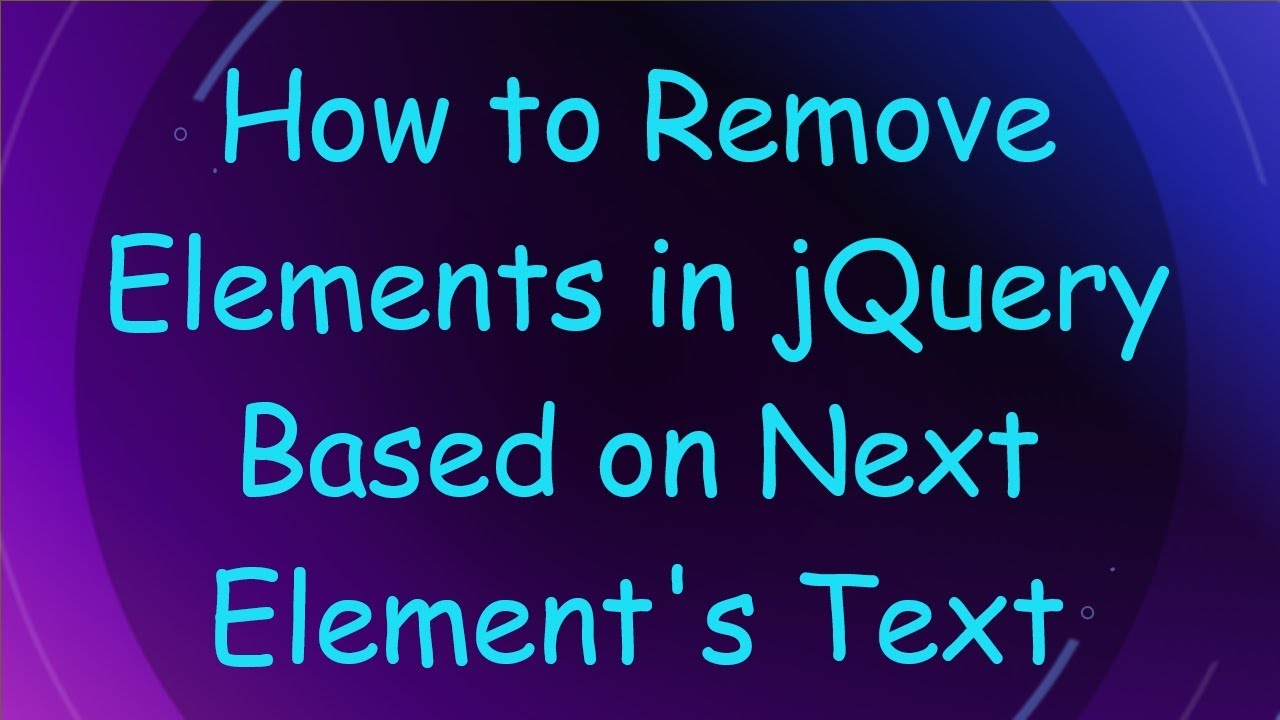
Показать описание
Learn how to efficiently use jQuery to remove elements that contain specific text following a target element in this easy-to-follow guide.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: jQuery - if element after another element contains text remove
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Efficiently Remove Elements in jQuery Based on Content
When working with web development, you might encounter situations where you need to manipulate the Document Object Model (DOM) based on certain conditions. For instance, you may have a div with a specific class, and you need to check if the next sibling element contains certain text. If it does, your goal is to remove that element entirely.
In this guide, we'll explore a practical solution in jQuery for achieving just that. Let's dig into a specific problem and its corresponding solution.
The Problem at Hand
Imagine you have the following HTML structure:
[[See Video to Reveal this Text or Code Snippet]]
In this case, you want to check if the <p> element that follows the <div> with the class wp-caption contains a non-breaking space ( ). If it does, you should remove that <p> element.
The jQuery Solution
Initial Approach
Here’s an initial attempt at solving this problem in jQuery:
[[See Video to Reveal this Text or Code Snippet]]
This code snippet checks if the next sibling of the div contains specific text and, if so, proceeds to remove it. However, there’s a more efficient way to accomplish the same function.
Optimized One-Liner Solution
You can achieve the same result with a simple one-liner in jQuery:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Solution
Next Sibling: The next("p:contains(' ')") method checks if the immediate next sibling is a paragraph <p> containing the specific text .
Removing the Element: The remove() function will delete the identified <p> elements from the DOM if the condition is met.
Why Use the One-Liner?
The optimized solution not only reduces the code length but also improves performance and readability. Here are some advantages of using a concise approach:
Fewer Lines of Code: It eliminates the need for an if statement and loops, which can clutter code.
Default Behavior of jQuery: jQuery inherently applies selectors to each element without needing additional looping, which keeps your code clean.
Practical Implementation
Here's how you can implement this in a full HTML structure:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
In summary, removing elements based on their following siblings in jQuery can be done effectively with a customized solution tailored to your needs. By utilizing the one-liner approach, you not only streamline your code but also enhance its efficiency.
Now that you've learned this technique, why not give it a try in your projects? Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: jQuery - if element after another element contains text remove
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Efficiently Remove Elements in jQuery Based on Content
When working with web development, you might encounter situations where you need to manipulate the Document Object Model (DOM) based on certain conditions. For instance, you may have a div with a specific class, and you need to check if the next sibling element contains certain text. If it does, your goal is to remove that element entirely.
In this guide, we'll explore a practical solution in jQuery for achieving just that. Let's dig into a specific problem and its corresponding solution.
The Problem at Hand
Imagine you have the following HTML structure:
[[See Video to Reveal this Text or Code Snippet]]
In this case, you want to check if the <p> element that follows the <div> with the class wp-caption contains a non-breaking space ( ). If it does, you should remove that <p> element.
The jQuery Solution
Initial Approach
Here’s an initial attempt at solving this problem in jQuery:
[[See Video to Reveal this Text or Code Snippet]]
This code snippet checks if the next sibling of the div contains specific text and, if so, proceeds to remove it. However, there’s a more efficient way to accomplish the same function.
Optimized One-Liner Solution
You can achieve the same result with a simple one-liner in jQuery:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Solution
Next Sibling: The next("p:contains(' ')") method checks if the immediate next sibling is a paragraph <p> containing the specific text .
Removing the Element: The remove() function will delete the identified <p> elements from the DOM if the condition is met.
Why Use the One-Liner?
The optimized solution not only reduces the code length but also improves performance and readability. Here are some advantages of using a concise approach:
Fewer Lines of Code: It eliminates the need for an if statement and loops, which can clutter code.
Default Behavior of jQuery: jQuery inherently applies selectors to each element without needing additional looping, which keeps your code clean.
Practical Implementation
Here's how you can implement this in a full HTML structure:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
In summary, removing elements based on their following siblings in jQuery can be done effectively with a customized solution tailored to your needs. By utilizing the one-liner approach, you not only streamline your code but also enhance its efficiency.
Now that you've learned this technique, why not give it a try in your projects? Happy coding!