filmov
tv
Applet Skeleton || Applet methods || init, start, paint, stop and destroy methods || Java Tutorial
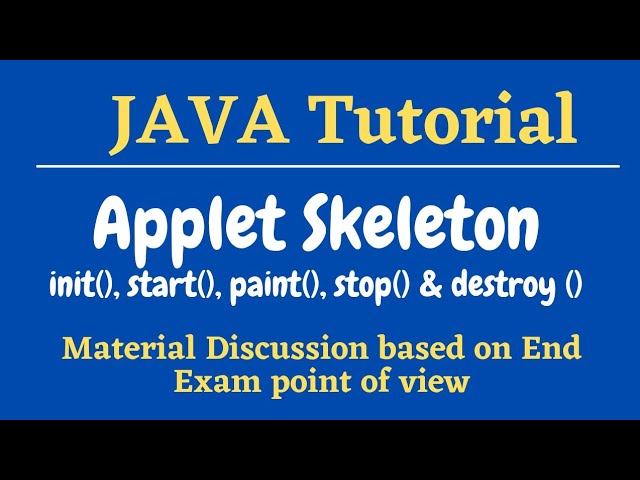
Показать описание
An Applet Skeleton
• Most applets override a set of methods shown below:
o init( ),
o start( ),
o stop( ), and
o destroy( )
• AWT-based applets will also override the paint () method, which is defined by the AWT Component class. This method is called when the applet’s output must be redisplayed.
• Example Program:
// An Applet skeleton.
/*
applet code="AppletSkel" width=300 height=100
/applet
*/
public class AppletSkel extends Applet
{
// Called first.
public void init()
{
// initialization
}
/* Called second, after init(). Also called whenever the applet is restarted. */
public void start()
{
// start or resume execution
}
// Called when the applet is stopped.
public void stop()
{
// suspends execution
}
/* Called when applet is terminated. This is the last method executed. */
public void destroy ()
{
// perform shutdown activities
}
// Called when an applet's window must be restored.
public void paint (Graphics g)
{
// redisplay contents of window
}
}
OUTPUT:
Applet Initialization and Termination
When an applet begins, the following methods are called, in this sequence:
1. init( )
2. start( )
3. paint( )
When an applet is terminated, the following sequence of method calls takes place:
1. stop( )
2. destroy( )
• init( )
o The init( ) method is the first method to be called. This is where you should initialize variables. This method is called only once during the run time of your applet.
• start( )
o The start( ) method is called after init( ).
o It is called to restart an applet after it has been stopped.
o start( ) is called each time an applet’s HTML document is displayed onscreen. So, if a user leaves a web page and comes back, the applet resumes execution at start( ).
• paint( )
o The paint( ) method is called each time your applet’s output must be redrawn.
• stop( )
o The stop( ) method is called when a web browser leaves the HTML document containing the applet—when it goes to another page, for example.
• destroy( )
o The destroy( ) method is called when the environment determines that your applet needs to be removed completely from memory.
At this point, you should free up any resources the applet may be using. The stop( ) method is always called before destroy( ).
• Most applets override a set of methods shown below:
o init( ),
o start( ),
o stop( ), and
o destroy( )
• AWT-based applets will also override the paint () method, which is defined by the AWT Component class. This method is called when the applet’s output must be redisplayed.
• Example Program:
// An Applet skeleton.
/*
applet code="AppletSkel" width=300 height=100
/applet
*/
public class AppletSkel extends Applet
{
// Called first.
public void init()
{
// initialization
}
/* Called second, after init(). Also called whenever the applet is restarted. */
public void start()
{
// start or resume execution
}
// Called when the applet is stopped.
public void stop()
{
// suspends execution
}
/* Called when applet is terminated. This is the last method executed. */
public void destroy ()
{
// perform shutdown activities
}
// Called when an applet's window must be restored.
public void paint (Graphics g)
{
// redisplay contents of window
}
}
OUTPUT:
Applet Initialization and Termination
When an applet begins, the following methods are called, in this sequence:
1. init( )
2. start( )
3. paint( )
When an applet is terminated, the following sequence of method calls takes place:
1. stop( )
2. destroy( )
• init( )
o The init( ) method is the first method to be called. This is where you should initialize variables. This method is called only once during the run time of your applet.
• start( )
o The start( ) method is called after init( ).
o It is called to restart an applet after it has been stopped.
o start( ) is called each time an applet’s HTML document is displayed onscreen. So, if a user leaves a web page and comes back, the applet resumes execution at start( ).
• paint( )
o The paint( ) method is called each time your applet’s output must be redrawn.
• stop( )
o The stop( ) method is called when a web browser leaves the HTML document containing the applet—when it goes to another page, for example.
• destroy( )
o The destroy( ) method is called when the environment determines that your applet needs to be removed completely from memory.
At this point, you should free up any resources the applet may be using. The stop( ) method is always called before destroy( ).