filmov
tv
PROBLEM SET 3: OUTDATED | SOLUTION (CS50 PYTHON)
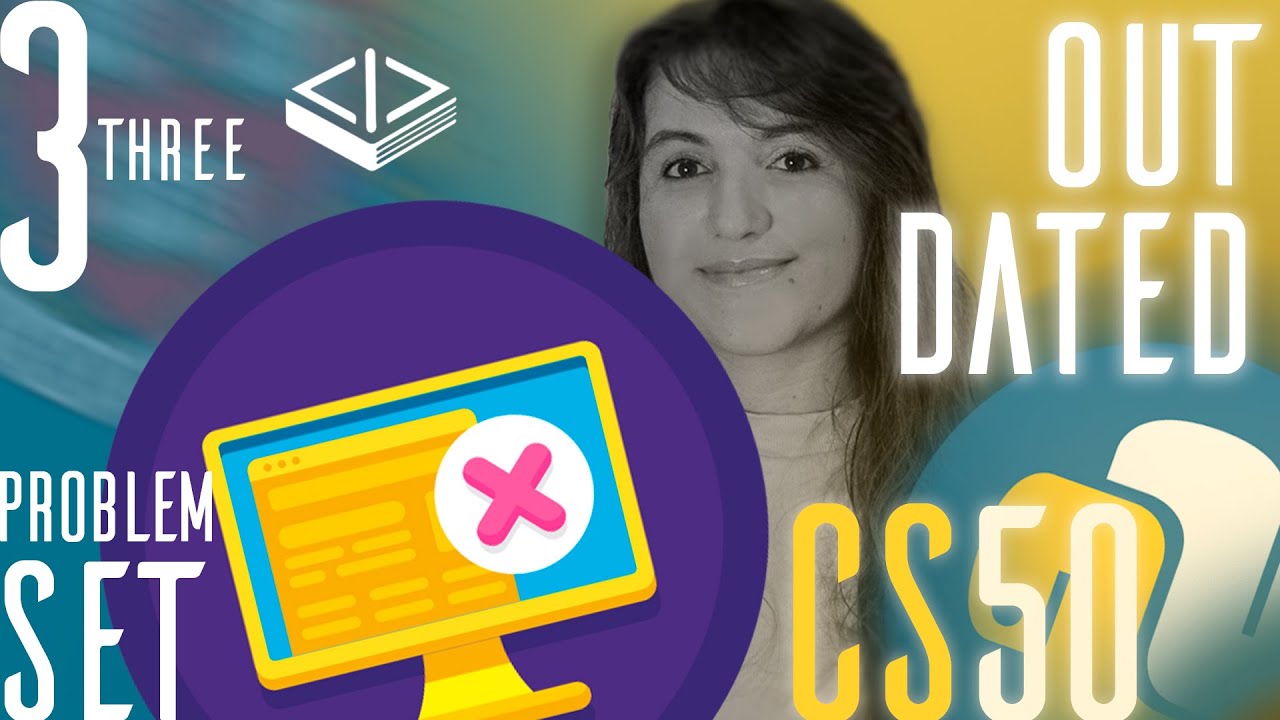
Показать описание
––– DISCLAIMER –––
The following videos are for educational purposes only. Cheating or any other activities are highly discouraged!! Using another person’s code breaks the academic honesty guidelines. This solution is for those who have finished the problem sets and want to watch for educational purposes, learning experience, and exploring alternative ways to approach problems and is NOT meant for those actively doing the problem sets. All problem sets presented in this video are owned by Harvard University.
–––
PROBLEM SET 3: OUTDATED | SOLUTION (CS50 PYTHON)
Outdated CS50P Solution – Problem Set 3
Outdated -Problem Set 3 (CS50's Introduction to Programming with Python)
CS50P - Problem Set 3 - Outdated
SOLVE CS50 PYTHON PROBLEM SET 3 : OUTDATED ( 2024 HARVARD FREE COURSE )
PROBLEM SET 3: OUTDATED | SOLUTION | Pythonista_geek | Codewithme | #CS50P
CS50P - Problem Set 3 - Outdated part 2
Why You SHOULD NOT Take Harvard CS50 in 2024
EP 32: WARNING: Outdated Coaching Tactics Will Hold You Back in 2025 – Here’s How to Stay Ahead
Is CS50P completely different from CS50x? - CS50 Reels #Shorts
Should I Take CS50 or CS50 Python?
How not to go about coding your CS50P Python problems
Arceus X vs All executors (Outdated) #ArceusX #delta #filmorago #roblox #hydrogen #Executor
Solving the 'Outdated Website' Problem Once and For All
Why minecraft says “Outdated server”
[CS50 Python] Solução 03.03: OUTDATED
[OUTDATED] Roblox's New Discord (Call System)
Why hasn't Apple invented this yet?!
[OUTDATED] How to set up TeamSpeak 3 for TRA
How to fix curseforge modpacks not loading in for minecraft 2021 (outdated)
Why Mane Has An Outdated Cracked iPhone
Standable v2.0 | QuickStart Guide (OUTDATED)
Updating project dependencies, npm outdated
Magento: Magento 2: extension installation module outdated issue (3 Solutions!!)
Комментарии