filmov
tv
Encode and Decode Strings - Leetcode 271 - Python
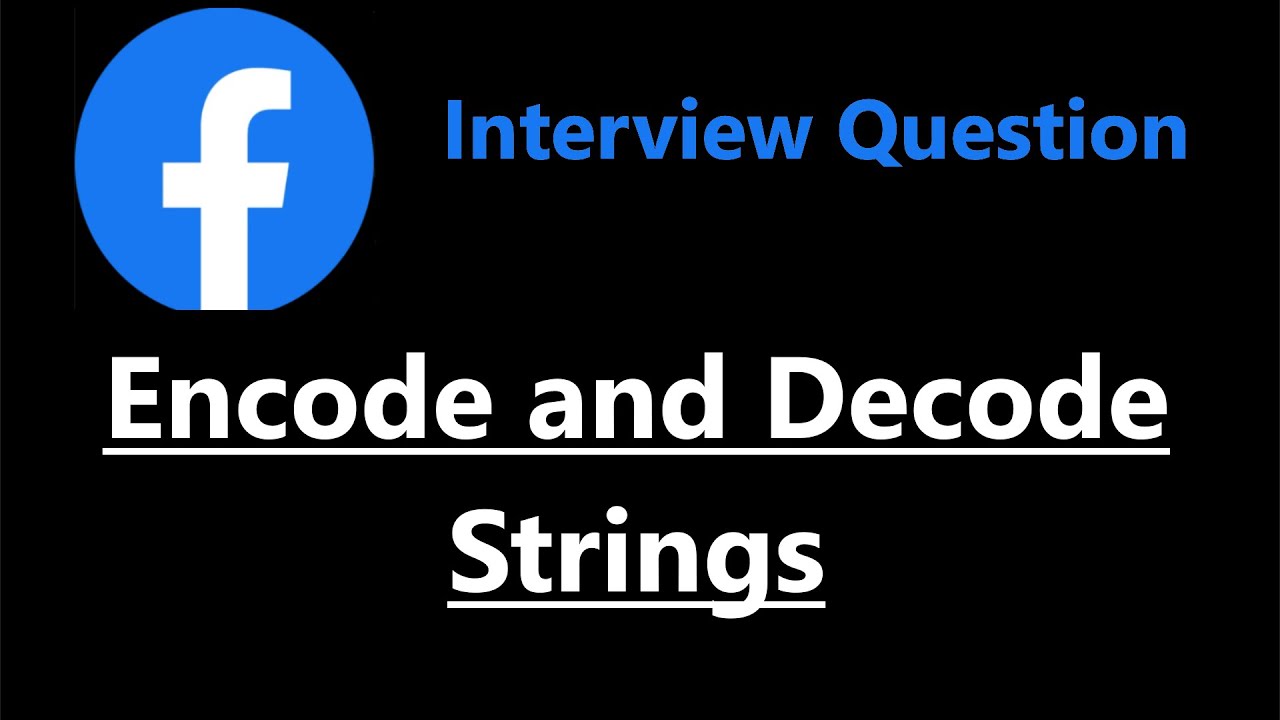
Показать описание
0:00 - Read the problem
1:47 - Drawing Explanation
7:04 - Coding Explanation
leetcode 271
#sorted #array #python
Encode and Decode Strings - Leetcode 271 - Python
Encode and Decode Strings - Leetcode 271
Encode and Decode Strings - Leetcode 271 - Python
[Java] Leetcode 271. Encode and Decode Strings [String #4]
Meta Interview Question - Encode and Decode Strings - LeetCode 271
Encode and Decode Strings | 271 Leetcode Premium | Simple & Optimal String approach | Python
659 · Encode and Decode Strings || NEETCODE 150
Leetcode 271. Encode and Decode Strings. Python (Easy)
Encoding and Decoding Strs | Easiest Solution | Leetcode
LeetCode 271 | Encode and Decode Strings | String | Java
Python standard library: Encoding and decoding strings
Quickly encode and decode Base64 text / strings
BLIND75: Encode and Decode Strings - Leetcode 271 - Python
Decode Strings (LeetCode 394) | Full solution with animations and examples | Study Algorithms
Encode and Decode Strings | Encode and Decode String with live coding | Easy explanation
LeetCode Decode String Solution Explained - Java
Decode String - Leetcode 394 - Python
How to Encode and Decode Strings with Base64 in Javascript
Encode and Decode Strings | Решение на Python | LeetCode 271
Leetcode - Encode and Decode TinyURL (Python)
Encode and Decode Strings: 271 - Leetcode Premium String interview @ google, meta, apple, linked-in
271. Encode and Decode Strings - Week 2/5 Leetcode August Challenge
c# program to encode and decode a string | decode base64 string c#
Encode and Decode Strings - Leetcode 271
Комментарии