filmov
tv
Creating Temporary Directories in Python
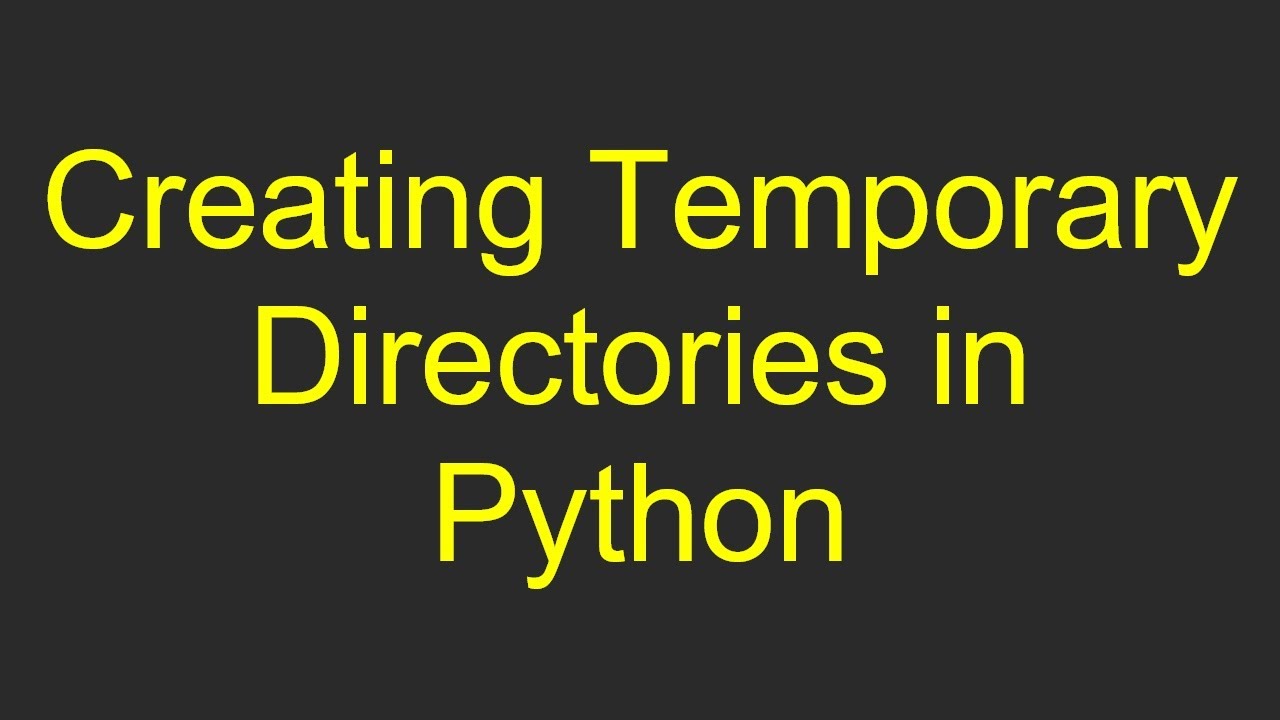
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to create a temporary directory in Python using various methods. This guide will help you understand the concept and implementation of temporary directories in Python for efficient and secure file handling.
---
Creating Temporary Directories in Python: A Quick Guide
When working with files and directories, especially in the context of temporary data, creating a temporary directory can be highly beneficial. Python provides several tools that make it easy to create and manage temporary directories. This post will guide you through the process, ensuring you understand how to create a temporary directory in Python effectively.
Understanding Temporary Directories
A temporary directory is a directory created for storing temporary files generated by your program. These directories are usually cleaned up automatically once they're no longer needed, helping to manage resources efficiently and avoid cluttering your file system with unnecessary data.
Using the tempfile Module
Python's tempfile module provides a convenient way to create temporary directories. This module is part of the Python Standard Library and can be used out-of-the-box without the need for any additional installation.
Here is a simple example of how to create a temporary directory using the tempfile module:
[[See Video to Reveal this Text or Code Snippet]]
In this example:
tempfile.TemporaryDirectory() creates a temporary directory.
The with statement ensures the directory is deleted once the block of code is exited, making cleanup effortless.
You can perform any necessary file operations within this temporary directory.
Advantages of Using Temporary Directories
Security
Temporary directories offer a secure place to handle sensitive data, ensuring that the data is not left lying around the file system.
Resource Management
Automatic cleanup prevents resource leakage, which could lead to file system clutter and potential resource exhaustion.
Convenience
Using temporary directories means you don't have to manually manage the lifecycle of the files and directories. The context manager handles it for you in a Pythonic manner.
Isolation
Temporary directories isolate temporary files from your main project directory, reducing conflict and potential errors in file handling.
Conclusion
Creating and using temporary directories in Python is straightforward, thanks to the tempfile module. This practice supports secure, efficient, and clutter-free file handling in your projects. Whether you are handling sensitive data or just looking for a convenient way to manage temporary files, Python's built-in tools will have you covered.
Understanding the basics of creating a temporary directory will ensure you can implement this in your projects, helping you write more secure and maintainable code.
Happy coding!
---
Summary: Learn how to create a temporary directory in Python using various methods. This guide will help you understand the concept and implementation of temporary directories in Python for efficient and secure file handling.
---
Creating Temporary Directories in Python: A Quick Guide
When working with files and directories, especially in the context of temporary data, creating a temporary directory can be highly beneficial. Python provides several tools that make it easy to create and manage temporary directories. This post will guide you through the process, ensuring you understand how to create a temporary directory in Python effectively.
Understanding Temporary Directories
A temporary directory is a directory created for storing temporary files generated by your program. These directories are usually cleaned up automatically once they're no longer needed, helping to manage resources efficiently and avoid cluttering your file system with unnecessary data.
Using the tempfile Module
Python's tempfile module provides a convenient way to create temporary directories. This module is part of the Python Standard Library and can be used out-of-the-box without the need for any additional installation.
Here is a simple example of how to create a temporary directory using the tempfile module:
[[See Video to Reveal this Text or Code Snippet]]
In this example:
tempfile.TemporaryDirectory() creates a temporary directory.
The with statement ensures the directory is deleted once the block of code is exited, making cleanup effortless.
You can perform any necessary file operations within this temporary directory.
Advantages of Using Temporary Directories
Security
Temporary directories offer a secure place to handle sensitive data, ensuring that the data is not left lying around the file system.
Resource Management
Automatic cleanup prevents resource leakage, which could lead to file system clutter and potential resource exhaustion.
Convenience
Using temporary directories means you don't have to manually manage the lifecycle of the files and directories. The context manager handles it for you in a Pythonic manner.
Isolation
Temporary directories isolate temporary files from your main project directory, reducing conflict and potential errors in file handling.
Conclusion
Creating and using temporary directories in Python is straightforward, thanks to the tempfile module. This practice supports secure, efficient, and clutter-free file handling in your projects. Whether you are handling sensitive data or just looking for a convenient way to manage temporary files, Python's built-in tools will have you covered.
Understanding the basics of creating a temporary directory will ensure you can implement this in your projects, helping you write more secure and maintainable code.
Happy coding!