filmov
tv
Python Lambda if statements
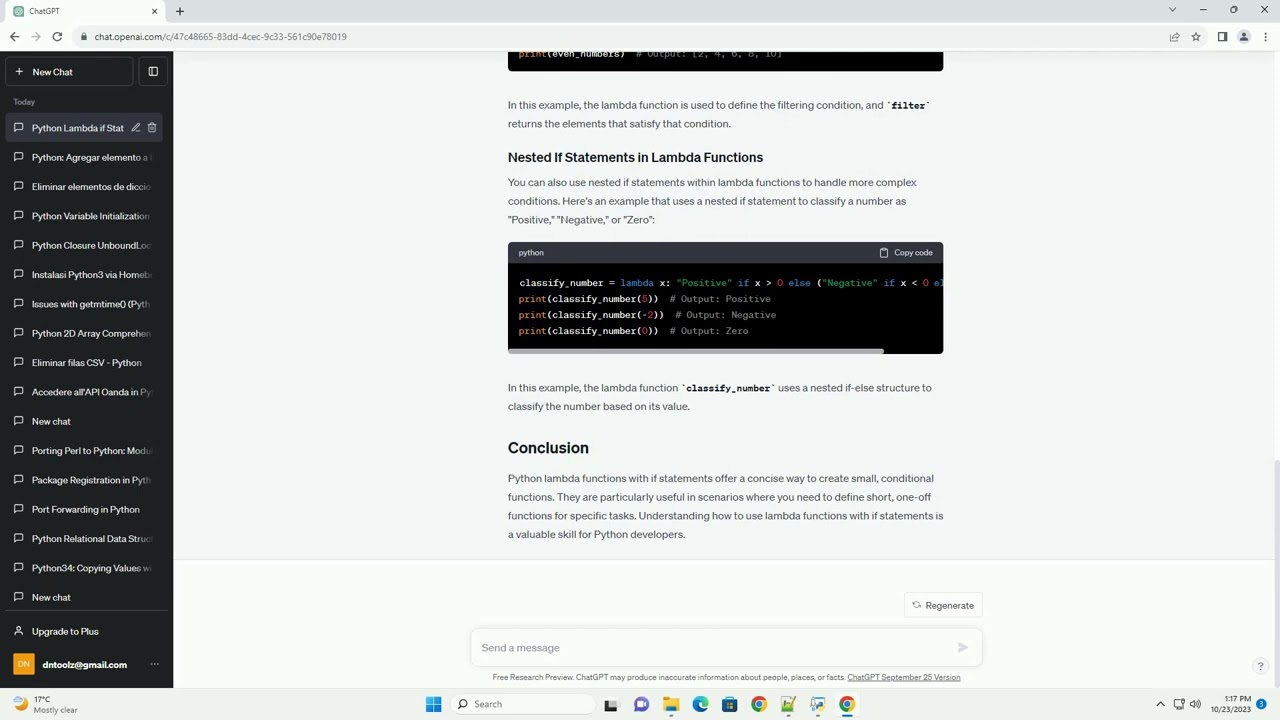
Показать описание
In Python, lambda functions are small, anonymous functions that can have any number of arguments but can only have one expression. Lambda functions are often used for short, simple operations where a full function definition is not necessary. One common use case for lambda functions is in combination with conditional if statements. In this tutorial, we will explore how to use lambda functions with if statements in Python, and we'll provide code examples to illustrate their usage.
A lambda function is defined using the lambda keyword, followed by the parameters and an expression. The syntax is as follows:
Here's a simple lambda function that takes two arguments and returns their sum:
Lambda functions can be used with if statements to create conditional expressions. You can think of it as a compact way to define a simple function that performs different operations based on a condition.
Let's start with a basic example of a lambda function with an if statement. Suppose we want to create a lambda function that returns the larger of two numbers. We can use the if statement in the lambda function as follows:
In this example, the lambda function find_larger takes two arguments, x and y. It returns x if x is greater than y, otherwise, it returns y.
Lambda functions can also be used in higher-order functions like map, filter, and reduce. Here's an example using filter and a lambda function to filter out even numbers from a list:
In this example, the lambda function is used to define the filtering condition, and filter returns the elements that satisfy that condition.
You can also use nested if statements within lambda functions to handle more complex conditions. Here's an example that uses a nested if statement to classify a number as "Positive," "Negative," or "Zero":
In this example, the lambda function classify_number uses a nested if-else structure to classify the number based on its value.
Python lambda functions with if statements offer a concise way to create small, conditional functions. They are particularly useful in scenarios where you need to define short, one-off functions for specific tasks. Understanding how to use lambda functions with if statements is a valuable skill for Python developers.
ChatGPT
A lambda function is defined using the lambda keyword, followed by the parameters and an expression. The syntax is as follows:
Here's a simple lambda function that takes two arguments and returns their sum:
Lambda functions can be used with if statements to create conditional expressions. You can think of it as a compact way to define a simple function that performs different operations based on a condition.
Let's start with a basic example of a lambda function with an if statement. Suppose we want to create a lambda function that returns the larger of two numbers. We can use the if statement in the lambda function as follows:
In this example, the lambda function find_larger takes two arguments, x and y. It returns x if x is greater than y, otherwise, it returns y.
Lambda functions can also be used in higher-order functions like map, filter, and reduce. Here's an example using filter and a lambda function to filter out even numbers from a list:
In this example, the lambda function is used to define the filtering condition, and filter returns the elements that satisfy that condition.
You can also use nested if statements within lambda functions to handle more complex conditions. Here's an example that uses a nested if statement to classify a number as "Positive," "Negative," or "Zero":
In this example, the lambda function classify_number uses a nested if-else structure to classify the number based on its value.
Python lambda functions with if statements offer a concise way to create small, conditional functions. They are particularly useful in scenarios where you need to define short, one-off functions for specific tasks. Understanding how to use lambda functions with if statements is a valuable skill for Python developers.
ChatGPT