filmov
tv
Handling Uncaught TypeError in JavaScript Arrays: A Guide to Fixing Undefined Values
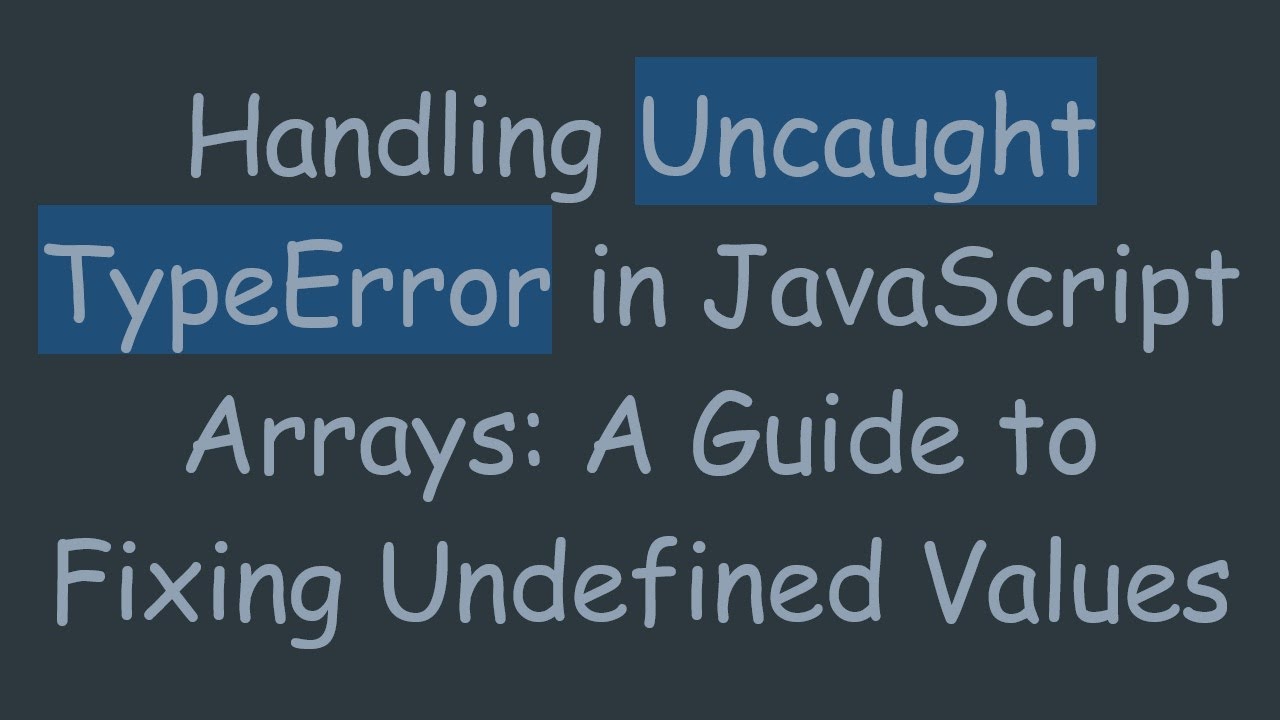
Показать описание
Discover how to effectively resolve the `Uncaught TypeError` in JavaScript when dealing with undefined array values and ensure smooth execution of your code.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Uncaught Type Error Within my Array Statement
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Uncaught TypeError in JavaScript Arrays
If you're a JavaScript developer, you might have encountered the frustrating Uncaught TypeError: Cannot read properties of undefined (reading 'length') error while working with arrays. This type of error arises when you try to access a property of an undefined value. Let’s explore this issue in detail and learn how to fix it effectively.
The Problem
In the code provided, the error occurs because the elements in the array are not populated with data, leading to an attempt to access the length property of an undefined value. The specific line causing the error is:
[[See Video to Reveal this Text or Code Snippet]]
When ovens_init[i] is empty, ovens_init[i][0] evaluates to undefined, and trying to access length on that causes the TypeError.
Sample Code Leading to the Error
[[See Video to Reveal this Text or Code Snippet]]
The Solution
To resolve the above error, follow these steps to improve the code and handle potential undefined scenarios.
1. Adjust the Condition in the Loop
By modifying the condition in the loop, you can ensure that it only evaluates length when there is a valid element:
[[See Video to Reveal this Text or Code Snippet]]
2. Explain the Changes Made
New Condition: The check ovens_init[i].length > 0 ensures that there is at least one item in the array before trying to access ovens_init[i][0].length.
Handling Empty Arrays: This effectively prevents accessing properties of undefined and makes your code more robust.
3. Test with Different Array Configurations
You can populate the array to test how the code behaves with real data.
For instance:
[[See Video to Reveal this Text or Code Snippet]]
In this case, the output for the maximum length will start showing results without throwing errors.
Conclusion
The Uncaught TypeError can be frustrating, but it's often a straightforward problem caused by trying to read properties from an undefined object. By ensuring that you check the existence of elements before attempting to access their properties, you can write more resilient JavaScript code.
By following the suggested steps, you can effectively handle these errors:
Modify conditions to check for array length.
Test your code with various data configurations.
If you are still facing issues, be sure to review the way you initialize and populate your arrays. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Uncaught Type Error Within my Array Statement
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Uncaught TypeError in JavaScript Arrays
If you're a JavaScript developer, you might have encountered the frustrating Uncaught TypeError: Cannot read properties of undefined (reading 'length') error while working with arrays. This type of error arises when you try to access a property of an undefined value. Let’s explore this issue in detail and learn how to fix it effectively.
The Problem
In the code provided, the error occurs because the elements in the array are not populated with data, leading to an attempt to access the length property of an undefined value. The specific line causing the error is:
[[See Video to Reveal this Text or Code Snippet]]
When ovens_init[i] is empty, ovens_init[i][0] evaluates to undefined, and trying to access length on that causes the TypeError.
Sample Code Leading to the Error
[[See Video to Reveal this Text or Code Snippet]]
The Solution
To resolve the above error, follow these steps to improve the code and handle potential undefined scenarios.
1. Adjust the Condition in the Loop
By modifying the condition in the loop, you can ensure that it only evaluates length when there is a valid element:
[[See Video to Reveal this Text or Code Snippet]]
2. Explain the Changes Made
New Condition: The check ovens_init[i].length > 0 ensures that there is at least one item in the array before trying to access ovens_init[i][0].length.
Handling Empty Arrays: This effectively prevents accessing properties of undefined and makes your code more robust.
3. Test with Different Array Configurations
You can populate the array to test how the code behaves with real data.
For instance:
[[See Video to Reveal this Text or Code Snippet]]
In this case, the output for the maximum length will start showing results without throwing errors.
Conclusion
The Uncaught TypeError can be frustrating, but it's often a straightforward problem caused by trying to read properties from an undefined object. By ensuring that you check the existence of elements before attempting to access their properties, you can write more resilient JavaScript code.
By following the suggested steps, you can effectively handle these errors:
Modify conditions to check for array length.
Test your code with various data configurations.
If you are still facing issues, be sure to review the way you initialize and populate your arrays. Happy coding!