filmov
tv
Kivy simple calculator | Python kivy | Code
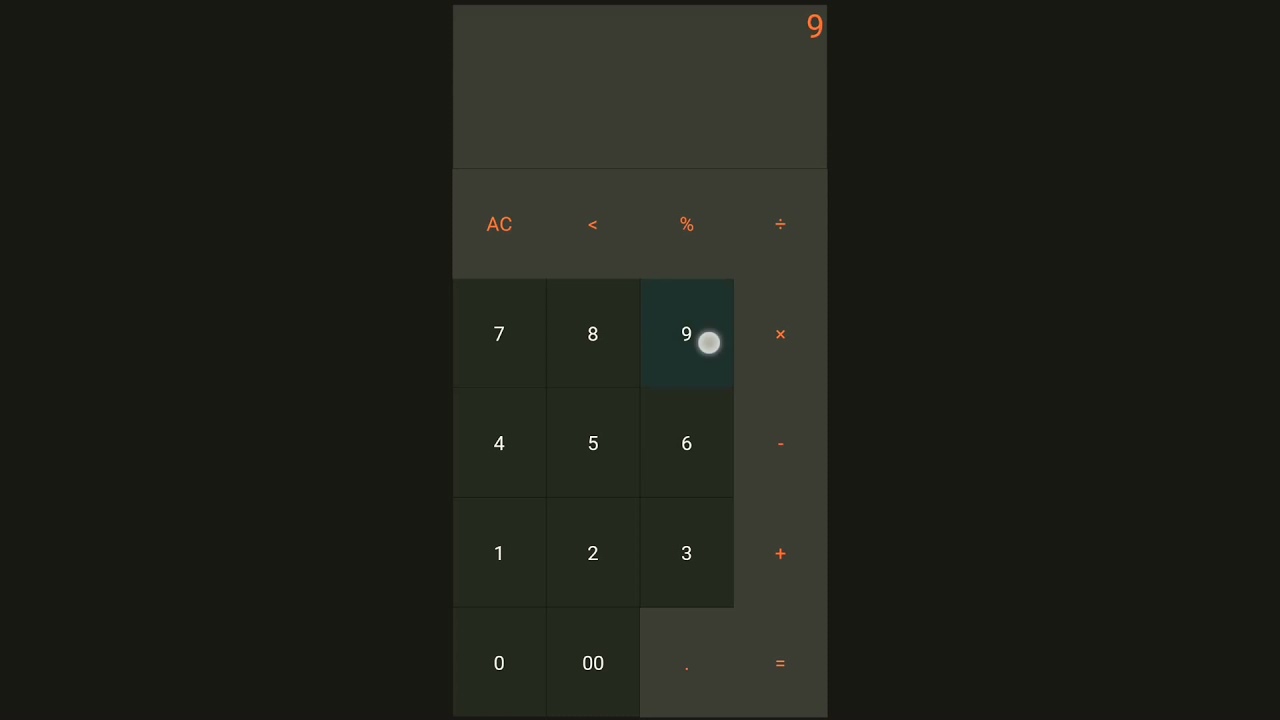
Показать описание
This is a simple calculator made with Python library called kivy.
The whole calculator code is present in the comments section.
___________
|subscribe|
````````````
Music used in the video-
Song: Aeden & Joellé - I Feel Crazy [NCS Release]
Music provided by NoCopyrightSounds
#python #code #kivy #programming #coding #GUI
The whole calculator code is present in the comments section.
___________
|subscribe|
````````````
Music used in the video-
Song: Aeden & Joellé - I Feel Crazy [NCS Release]
Music provided by NoCopyrightSounds
#python #code #kivy #programming #coding #GUI
Build A Simple Calculator App - Python Kivy GUI Tutorial #15
Kivy simple calculator | Python kivy | Code
Calculator 04 kivy tutorial #kivy #python
Creating a calculator in Python using Kivy | Follow - Along coding
Simple Calculator Using Kivy In Python | Kivy Tutorial
A Calculator App Built With Kivy Python
Kivy Calculator | Python
How to create a GUI Calculator with Python and Kivy || Random Coding Turorial
Calculator by python | Using Kivy module | Simple python project 2021
Make a Scientific Calculator with python's Kivy and Kivymd | Kivy | kivymd | Python | DEMO |
Calculator Using Python and Kivy
Kivy-python/Simple Calculator/Addition button/#kivy
2. Simple Calculator in Kivy (Python Framework)
Simple Android Calculator App in Python
Calculator in Python using Kivy.
Building The Best Calculator With Python Kivy [python language 2023]
Kivy Calculator
PYTHON Calculator using KIVY!!!!!
Beautiful Calculator - Kivy ♥️🐍 | Python | Pydroid3
How to make a Scientific Calculator with Kivy?
How to make a simple Calculator in python/#kivy #13
simple Calculator by Python Kivy running on Android
Creating a calculator in Python using Kivy !
Calculator App using Kivy in Python | Python Kivy tutorial | Great Learning
Комментарии