filmov
tv
Leetcode - Sort Characters By Frequency (Python)
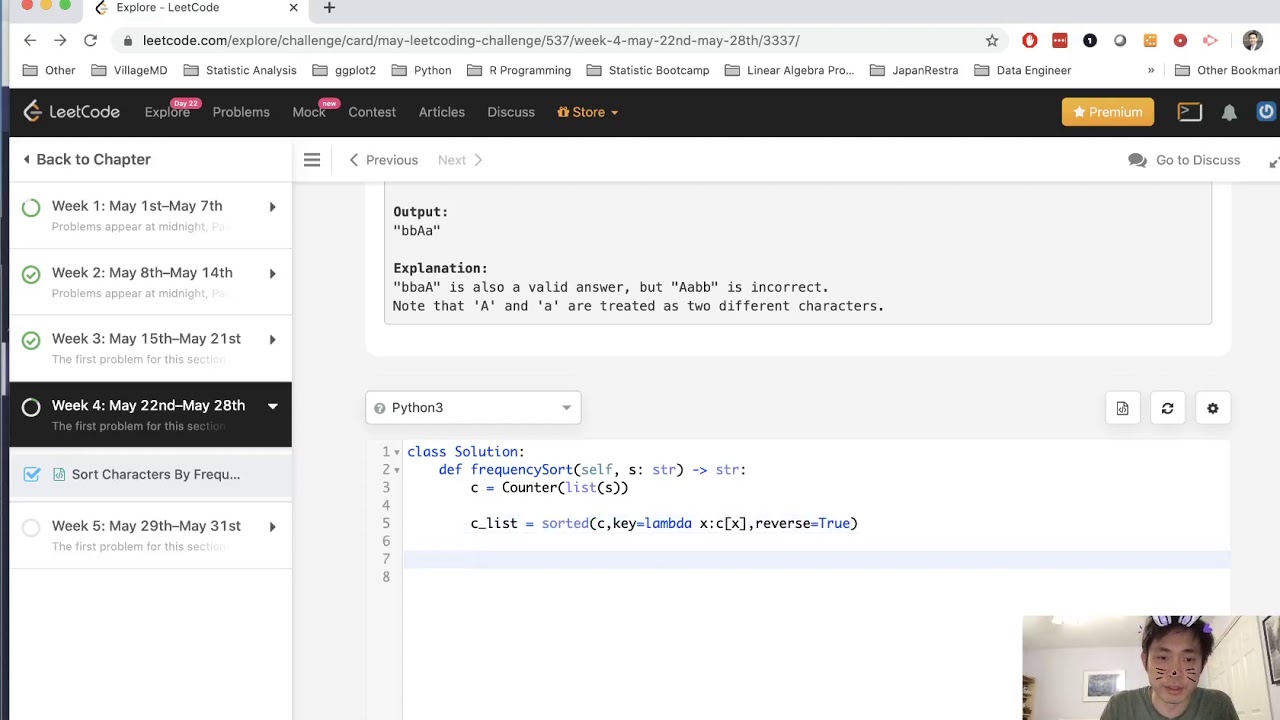
Показать описание
May 2020 Leetcode Challenge
Leetcode - Sort Characters By Frequency (Python3)
Leetcode - Sort Characters By Frequency (Python3)
Sort Characters By Frequency - Leetcode 451 - Python
Sort Characters By Frequency | Leetcode #451
Leetcode - Sort Characters By Frequency (Python)
Sort Characters by Frequency | LeetCode 451 | C++, Java, Python | May LeetCoding Day 22
Sort Characters By Frequency | LeetCode 451 | Top Interview Question | Java DSA | Geekster Amazon
HOW TO Sort Characters By Frequency - Leetcode 451
Sort Characters By Frequency | Sort Characters By Frequency LeetCode Java | LeetCode - 451
Sort Characters by Frequency
LeetCode: Sort Characters By Frequency
Leetcode 451. Sort Characters By Frequency | JavaScript | Hash Table
451. Sort Characters By Frequency | 3 Ways | Sorting | Hash Table | Bucket Sort | Adobe | Apple
LeetCode 451 Sort Characters By Frequency - python
LeetCode - Sort Characters By Frequency
Leetcode 451 Sort Characters By Frequency
Leetcode | 451. Sort Characters By Frequency | Medium | Java [2 Methods]
LeetCode 451 - Sort Characters By Frequency: A Microsoft Journey
Leetcode 451 | Sort Characters By Frequency | C++ | Coding
Leetcode Question 451: Sort Characters By Frequency
Sort Characters By Frequency | Day 22 | [May LeetCoding Challenge] [Leetcode 451] [2020]
[Java] Leetcode 451. Sort Characters By Frequency [Top K Elements #2]
451. Sort Characters By Frequency - Day 22/31 Leetcode October Challenge
LeetCode 451 - Sort Characters By Frequency - Python
Sort characters by frequency | leetcode 451
Sort Characters By Frequency Leetcode 451
Комментарии