filmov
tv
Combining Type Annotations and Type Inference in TypeScript - #7 #typescript #TypeAnnotations
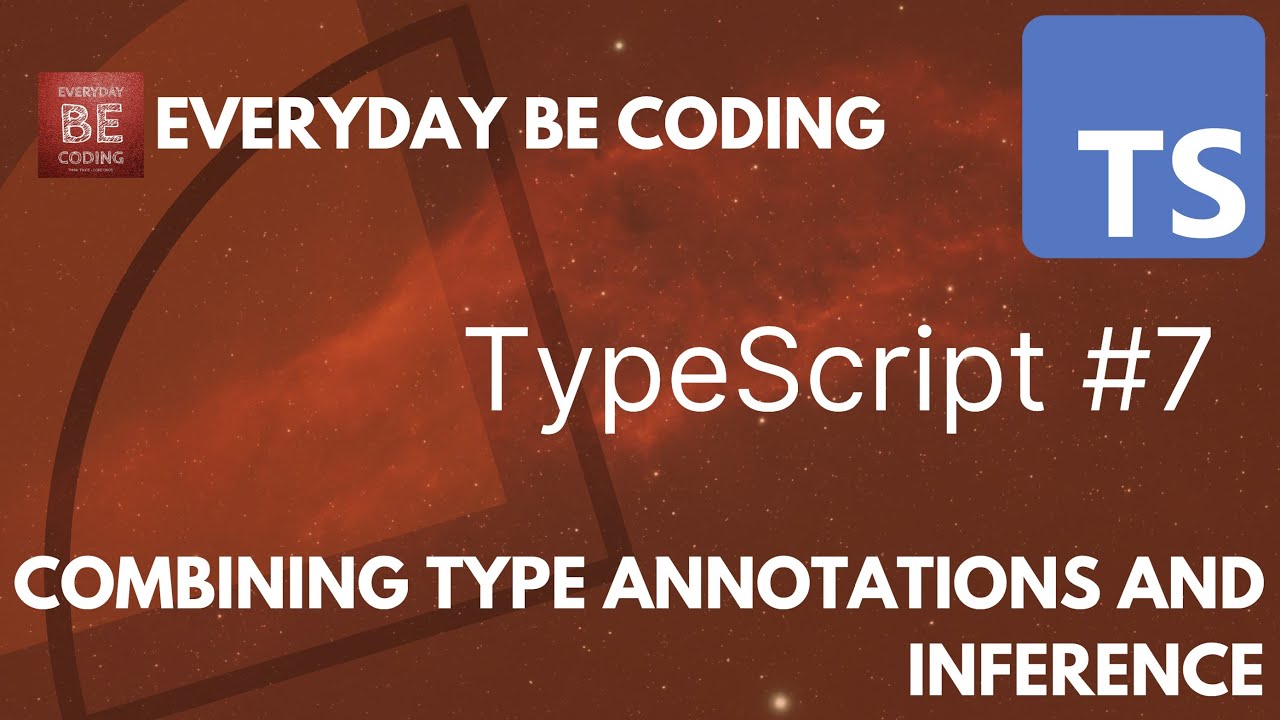
Показать описание
#typescript #TypeAnnotations
#TypeInference #JavaScript #WebDevelopment #Coding #Programming
#SoftwareEngineering #FrontEndDevelopment #TechTutorial #CodeNewbie
#LearnToCode #Developer #WebDev #ProgrammingTips #TypeScriptTips #JSFramework #TechEducation #CodingLife #FullStackDeveloper
#CodeNewbie #LearnToCode #Developer #WebDev #ProgrammingTips
#TypeScriptTips #JSFramework #TechEducation #CodingLife #FullStackDeveloper
"Type annotations and type inference in TypeScript" are fundamental concepts that help TypeScript serve its main purpose: to add static typing to JavaScript, making the code easier to manage and less prone to runtime errors. Let's break down each concept:
Type Annotations
Type annotations in TypeScript allow you to explicitly specify the type of a variable, function parameter, function return type, and object properties. By adding a type annotation, you tell TypeScript what type of value the variable or expression should hold, which helps during compilation by checking type correctness.
Here are some examples of type annotations:
typescript code:
let username: string = "JohnDoe";
let age: number = 30;
let isActive: boolean = true;
function greet(name: string): string {
return "Hello, " + name;
}
In these examples:
username is annotated as a string.
age is annotated as a number.
isActive is a boolean.
The function greet takes one parameter name which is a string and returns a string.
Type Inference
Type inference refers to TypeScript's ability to automatically deduce the types of variables and expressions when it has sufficient information. This feature minimizes the amount of explicit type annotations you need to provide, leading to cleaner and more readable code.
Here’s an example of type inference:
typescript code:
let isAdmin = true; // TypeScript infers type boolean
In this case, you don’t need to explicitly declare isAdmin as a boolean because TypeScript can infer it from the assigned value. Similarly, TypeScript uses inference in more complex scenarios, such as determining the return type of functions based on the types of the return statements.
typescript code:
function getLength(item: string | string[]) {
}
Here, TypeScript infers the return type of getLength as number because both string and string[] types have a length property of type number.
Combining Type Annotations and Type Inference
TypeScript developers typically balance between explicitly annotating types and relying on type inference. In general, providing type annotations at function boundaries (for the parameters and return values) is considered good practice, as it ensures your functions' contracts are clear. For local variables within functions, relying on type inference can make code more concise without sacrificing the safety and benefits of static typing.
Understanding and utilizing these features effectively can significantly enhance your development experience with TypeScript by providing a robust framework for catching errors at compile time, improving code documentation, and making refactoring easier.
Chapter :
00:00 Introduction to Type Annotations and Type Inference
00:12 Type Annotations
00:27 Example of Type Annotations
01:02 Type Inference
01:26 Example of Type Inference
01:40 Combining Type Annotations and Type Inference
02:07 Summery
Thank you for watching this video
Everyday Be coding
#TypeInference #JavaScript #WebDevelopment #Coding #Programming
#SoftwareEngineering #FrontEndDevelopment #TechTutorial #CodeNewbie
#LearnToCode #Developer #WebDev #ProgrammingTips #TypeScriptTips #JSFramework #TechEducation #CodingLife #FullStackDeveloper
#CodeNewbie #LearnToCode #Developer #WebDev #ProgrammingTips
#TypeScriptTips #JSFramework #TechEducation #CodingLife #FullStackDeveloper
"Type annotations and type inference in TypeScript" are fundamental concepts that help TypeScript serve its main purpose: to add static typing to JavaScript, making the code easier to manage and less prone to runtime errors. Let's break down each concept:
Type Annotations
Type annotations in TypeScript allow you to explicitly specify the type of a variable, function parameter, function return type, and object properties. By adding a type annotation, you tell TypeScript what type of value the variable or expression should hold, which helps during compilation by checking type correctness.
Here are some examples of type annotations:
typescript code:
let username: string = "JohnDoe";
let age: number = 30;
let isActive: boolean = true;
function greet(name: string): string {
return "Hello, " + name;
}
In these examples:
username is annotated as a string.
age is annotated as a number.
isActive is a boolean.
The function greet takes one parameter name which is a string and returns a string.
Type Inference
Type inference refers to TypeScript's ability to automatically deduce the types of variables and expressions when it has sufficient information. This feature minimizes the amount of explicit type annotations you need to provide, leading to cleaner and more readable code.
Here’s an example of type inference:
typescript code:
let isAdmin = true; // TypeScript infers type boolean
In this case, you don’t need to explicitly declare isAdmin as a boolean because TypeScript can infer it from the assigned value. Similarly, TypeScript uses inference in more complex scenarios, such as determining the return type of functions based on the types of the return statements.
typescript code:
function getLength(item: string | string[]) {
}
Here, TypeScript infers the return type of getLength as number because both string and string[] types have a length property of type number.
Combining Type Annotations and Type Inference
TypeScript developers typically balance between explicitly annotating types and relying on type inference. In general, providing type annotations at function boundaries (for the parameters and return values) is considered good practice, as it ensures your functions' contracts are clear. For local variables within functions, relying on type inference can make code more concise without sacrificing the safety and benefits of static typing.
Understanding and utilizing these features effectively can significantly enhance your development experience with TypeScript by providing a robust framework for catching errors at compile time, improving code documentation, and making refactoring easier.
Chapter :
00:00 Introduction to Type Annotations and Type Inference
00:12 Type Annotations
00:27 Example of Type Annotations
01:02 Type Inference
01:26 Example of Type Inference
01:40 Combining Type Annotations and Type Inference
02:07 Summery
Thank you for watching this video
Everyday Be coding