filmov
tv
Understanding and Resolving Java's `java.lang.OutOfMemoryError: Java heap space`
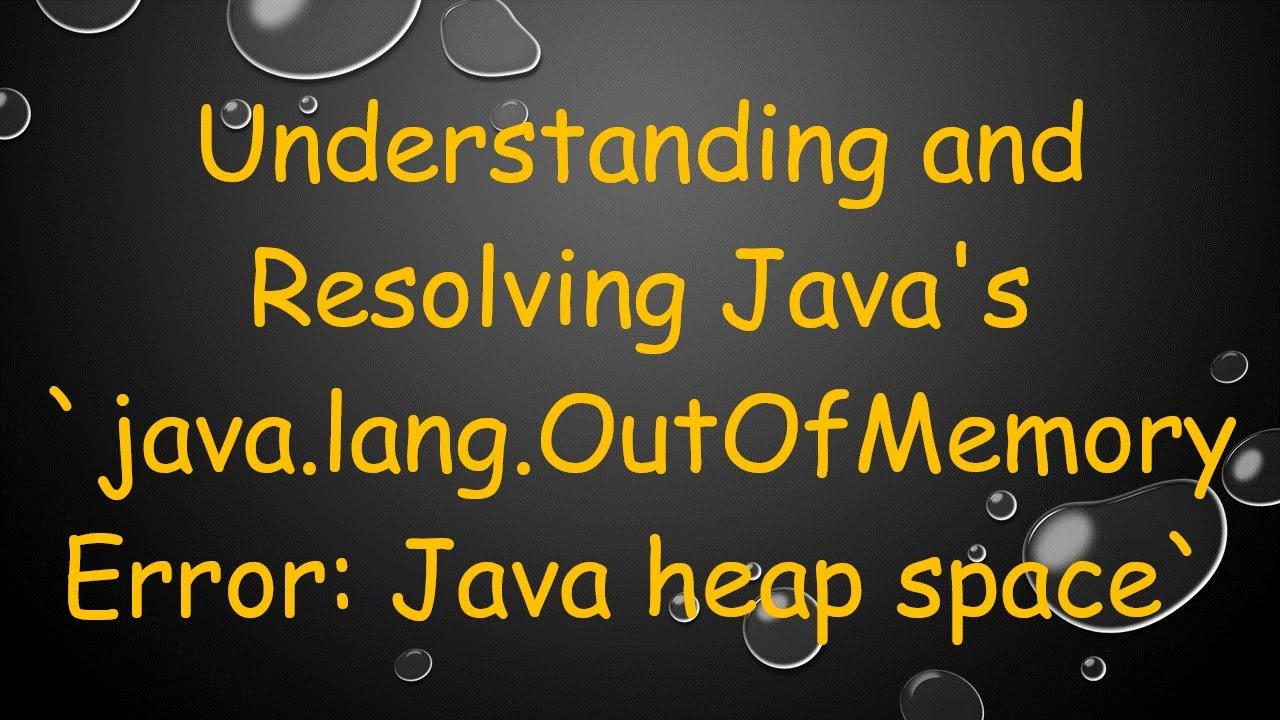
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
---
This error occurs when the Java Virtual Machine (JVM) is unable to allocate an object because it has exhausted its available heap memory. The heap space is where Java applications dynamically allocate memory for objects at runtime. When this space overflows, the JVM throws an OutOfMemoryError.
Several factors can lead to this error:
Memory Leaks: When an application unintentionally retains references to objects that are no longer needed, these objects cannot be garbage collected, eventually consuming all available heap memory.
High Memory Demand: Applications with high memory requirements, particularly those handling large datasets or performing extensive computations, might exhaust the heap space.
Default Heap Size Limits: The default configuration of the JVM may allocate insufficient heap memory for the needs of your application.
Infinite Loop or Large Data Structures: Mismanaged loops that create excessive objects or data structures that grow uncontrollably can also lead to heap space exhaustion.
Successfully diagnosing this error requires identifying the memory usage patterns and pinpointing memory leaks or heavy memory consumers within your application. Here are some tools and techniques to diagnose this issue:
Heap Dump Analysis: Generate a heap dump file using JVM options (e.g., -XX:+HeapDumpOnOutOfMemoryError). This file can be analyzed using tools like Eclipse MAT (Memory Analyzer Tool) to identify memory leaks or objects consuming excessive memory.
Profiling Tools: Utilize profiling tools such as VisualVM, JProfiler, or YourKit to monitor memory usage and find suspicious memory patterns.
Garbage Collection Logs: Enable GC logging (e.g., using -Xlog:gc* for Java 9+) to understand the garbage collection behavior and how frequently the JVM is running out of memory.
Once the root cause is identified, implementing solutions to mitigate this error involves adopting various strategies:
Increase Heap Size: Adjust the maximum heap size allocated to the JVM using the -Xmx option. This provides more heap memory for the application to use. For example, -Xmx2g configures the heap size to 2 GB.
Optimize Code: Review the application code to eliminate memory leaks, optimize memory usage, and improve data handling to avoid creating large unnecessary objects.
Use Efficient Data Structures: Replace memory-inefficient data structures with more memory-efficient alternatives, such as using ArrayList instead of LinkedList when random access is more frequent.
Monitor and Tune GC: Tuning the garbage collector settings (e.g., using the -XX:MaxGCPauseMillis= flag) can help ensure that memory is efficiently reclaimed.
Implement Caching Strategies: Implement appropriate caching mechanisms to reuse objects and prevent repeated allocation of the same data.
Conclusion
---
---
This error occurs when the Java Virtual Machine (JVM) is unable to allocate an object because it has exhausted its available heap memory. The heap space is where Java applications dynamically allocate memory for objects at runtime. When this space overflows, the JVM throws an OutOfMemoryError.
Several factors can lead to this error:
Memory Leaks: When an application unintentionally retains references to objects that are no longer needed, these objects cannot be garbage collected, eventually consuming all available heap memory.
High Memory Demand: Applications with high memory requirements, particularly those handling large datasets or performing extensive computations, might exhaust the heap space.
Default Heap Size Limits: The default configuration of the JVM may allocate insufficient heap memory for the needs of your application.
Infinite Loop or Large Data Structures: Mismanaged loops that create excessive objects or data structures that grow uncontrollably can also lead to heap space exhaustion.
Successfully diagnosing this error requires identifying the memory usage patterns and pinpointing memory leaks or heavy memory consumers within your application. Here are some tools and techniques to diagnose this issue:
Heap Dump Analysis: Generate a heap dump file using JVM options (e.g., -XX:+HeapDumpOnOutOfMemoryError). This file can be analyzed using tools like Eclipse MAT (Memory Analyzer Tool) to identify memory leaks or objects consuming excessive memory.
Profiling Tools: Utilize profiling tools such as VisualVM, JProfiler, or YourKit to monitor memory usage and find suspicious memory patterns.
Garbage Collection Logs: Enable GC logging (e.g., using -Xlog:gc* for Java 9+) to understand the garbage collection behavior and how frequently the JVM is running out of memory.
Once the root cause is identified, implementing solutions to mitigate this error involves adopting various strategies:
Increase Heap Size: Adjust the maximum heap size allocated to the JVM using the -Xmx option. This provides more heap memory for the application to use. For example, -Xmx2g configures the heap size to 2 GB.
Optimize Code: Review the application code to eliminate memory leaks, optimize memory usage, and improve data handling to avoid creating large unnecessary objects.
Use Efficient Data Structures: Replace memory-inefficient data structures with more memory-efficient alternatives, such as using ArrayList instead of LinkedList when random access is more frequent.
Monitor and Tune GC: Tuning the garbage collector settings (e.g., using the -XX:MaxGCPauseMillis= flag) can help ensure that memory is efficiently reclaimed.
Implement Caching Strategies: Implement appropriate caching mechanisms to reuse objects and prevent repeated allocation of the same data.
Conclusion