filmov
tv
Control a water pump by Arduino
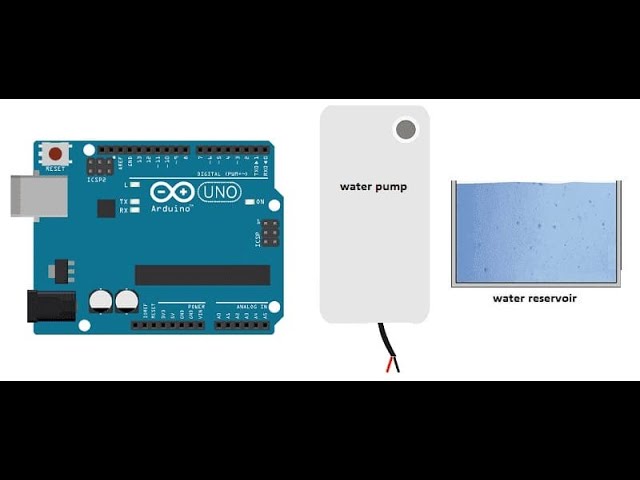
Показать описание
A water pump is a mechanical device used to move water from one location to another. There are different types of water pumps, including centrifugal pumps, submersible pumps, and jet pumps. Water pumps are commonly used in various applications, such as irrigation, water supply systems, and industrial processes.
Arduino UNO is a microcontroller board based on the ATmega328P microcontroller. It is one of the most popular and widely used boards in the Arduino family of microcontroller boards. The board is designed to be easy to use and is popular among hobbyists and professionals alike.
A relay is an electrical switch that is operated by an electromagnet. It is used to switch high voltage or high current devices using a low voltage or low current signal. It consists of a coil, contacts, and a housing.
Controlling a water pump with an Arduino board is a relatively simple process. Here are the basic steps you can follow:
Gather your materials:
- Arduino board (such as the Arduino Uno or Nano)
- Relay module
- Water pump
- Jumper wires
- Power supply (battery or wall adapter)
Connect the relay module to the Arduino:
1- Connect the VCC pin of the relay module to the 5V pin of the Arduino
2- Connect the GND pin of the relay module to the GND pin of the Arduino
3- Connect the IN pin of the relay module to a digital pin of the Arduino (e.g. pin 7)
4- Connect the water pump to the relay module:
5- Connect the positive wire of the water pump to the NO (Normally Open) pin of the relay module
6- Connect the negative wire of the water pump to the GND pin of the relay module
7- Write the Arduino code:
- Open the Arduino IDE and create a new sketch
- Define the pin number used to control the relay module as a constant variable
- In the setup() function, set the pin used to control the relay module as an OUTPUT pin
- In the loop() function, use the digitalWrite() function to turn the relay module on or off, depending on the desired state of the water pump
Here's an example code:
const int relayPin = 7; // Define the pin used to control the relay module
void setup() {
pinMode(relayPin, OUTPUT); // Set the relay pin as an OUTPUT pin
}
void loop() {
digitalWrite(relayPin, HIGH); // Turn on the relay module and pump
delay(5000); // Wait for 5 seconds
digitalWrite(relayPin, LOW); // Turn off the relay module and pump
delay(5000); // Wait for another 5 seconds
}
This code will turn on the water pump for 5 seconds, then turn it off for another 5 seconds, and repeat the process continuously. You can modify the code to adjust the duration of the pump's on and off periods, or use other sensors or inputs to control the pump's operation.
Комментарии