filmov
tv
Understanding the Difference Between std::string and std::string_view in C++
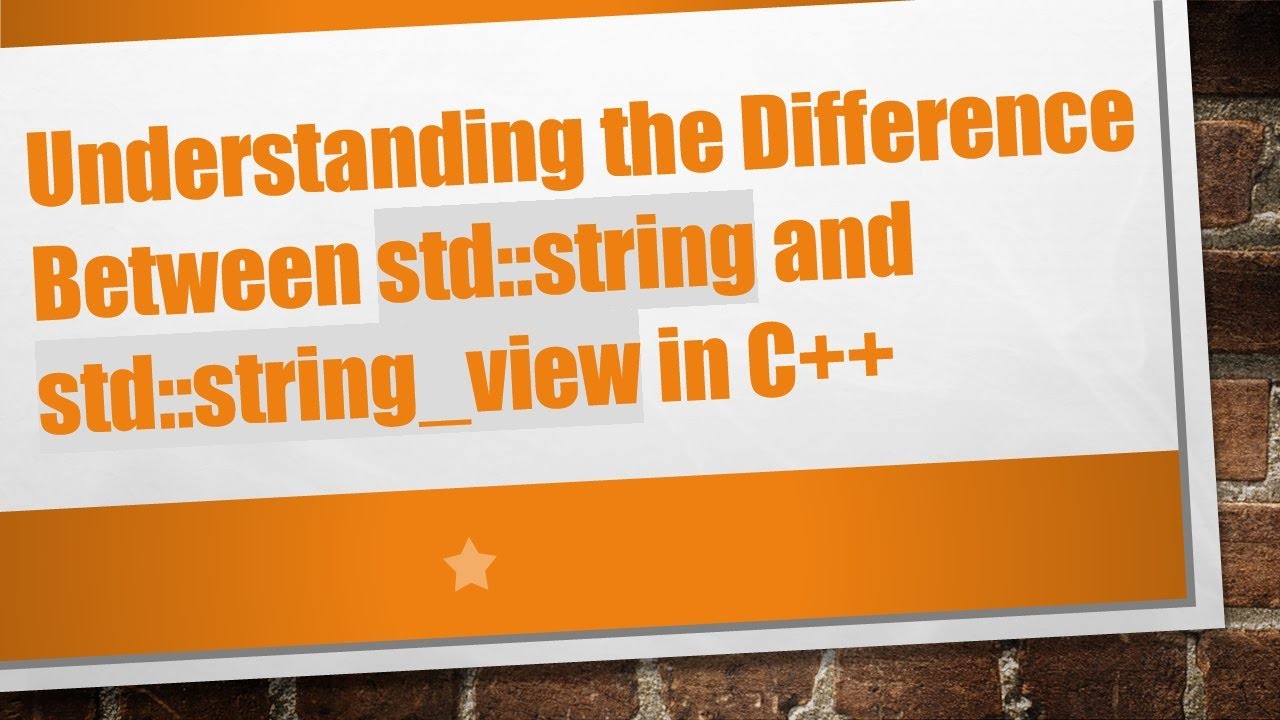
Показать описание
Discover whether you can replace `const std::string &` with `std::string_view` in C++ functions, and learn the implications of doing so.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Replace const std::string passed by reference, with std::string_view
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Difference Between std::string and std::string_view in C++
In C++, handling string data efficiently is crucial for performance, particularly when it comes to large datasets or environments with limited memory. A common question developers face is whether they can substitute const std::string & with std::string_view when passing strings to functions. This article dives into this topic, exploring each type's features and when one may be a better choice over the other.
What's the Problem?
Consider the following function signature in C++:
[[See Video to Reveal this Text or Code Snippet]]
The function accepts a string by reference, meaning no copy is made, and the content of the string isn’t modified. The question arises: Is it equivalent to use std::string_view instead?
[[See Video to Reveal this Text or Code Snippet]]
The presence of std::string_view suggests a more efficient way to handle strings as it also allows for read-only access. How does this compare in terms of functionality, memory usage, and runtime performance? Let's break this down.
Key Differences Between const std::string & and std::string_view
1. Null-Termination Requirement
const std::string &: This type ensures that the string is null-terminated. Therefore, if you're going to pass the string to a C function that expects null-terminated strings, using const std::string & is beneficial.
std::string_view: While it allows reading string data, it does not guarantee null-termination. This can lead to issues when interfacing with C APIs that expect null-terminated strings.
2. Compatibility with C++ Functions and Libraries
const std::string &: In some cases, you might need to pass strings to functions or libraries that specifically require const std::string &. Changing this to std::string_view could result in code that doesn’t compile or behaves unexpectedly.
std::string_view: This is more flexible for many modern C++ functions and works well when you're dealing with string manipulations within the bounds of C++.
3. Memory Management and Performance
Passing by Reference vs. Value: const std::string & is passed by reference, meaning that the compiler must access the original string data location every time properties like size, capacity, or data are accessed.
std::string_view: This is passed by value. It stores a size and a pointer to the string data, enabling faster access times since there's no need to reference the actual object each time. Memory overhead is minimized as well because it does not manage capacity.
When to Use Each
Here are two scenarios where you should prefer const std::string &:
Interfacing with C legacy code: If you need to call C functions that require null-terminated strings, stick with const std::string &.
Needing to store the string: If the string needs to be stored for later use or interfacing with a specific C++ library, const std::string & keeps the full std::string functionalities intact.
For all other cases, std::string_view is generally a better option, especially when dealing with functions that only need to read the string without modifying it.
Conclusion
In summary, while both const std::string & and std::string_view provide read-only access to string data, they're not interchangeable in every context. Being aware of their differences regarding null-termination, compatibility with C functions, and performance can help optimize your code effectively. Choose wisely based on your specific application's needs to take full advantage of what C++ has to offer.
Understanding these distinctions will empower you in writing more effective, efficient, and error-free C++ code.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Replace const std::string passed by reference, with std::string_view
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Difference Between std::string and std::string_view in C++
In C++, handling string data efficiently is crucial for performance, particularly when it comes to large datasets or environments with limited memory. A common question developers face is whether they can substitute const std::string & with std::string_view when passing strings to functions. This article dives into this topic, exploring each type's features and when one may be a better choice over the other.
What's the Problem?
Consider the following function signature in C++:
[[See Video to Reveal this Text or Code Snippet]]
The function accepts a string by reference, meaning no copy is made, and the content of the string isn’t modified. The question arises: Is it equivalent to use std::string_view instead?
[[See Video to Reveal this Text or Code Snippet]]
The presence of std::string_view suggests a more efficient way to handle strings as it also allows for read-only access. How does this compare in terms of functionality, memory usage, and runtime performance? Let's break this down.
Key Differences Between const std::string & and std::string_view
1. Null-Termination Requirement
const std::string &: This type ensures that the string is null-terminated. Therefore, if you're going to pass the string to a C function that expects null-terminated strings, using const std::string & is beneficial.
std::string_view: While it allows reading string data, it does not guarantee null-termination. This can lead to issues when interfacing with C APIs that expect null-terminated strings.
2. Compatibility with C++ Functions and Libraries
const std::string &: In some cases, you might need to pass strings to functions or libraries that specifically require const std::string &. Changing this to std::string_view could result in code that doesn’t compile or behaves unexpectedly.
std::string_view: This is more flexible for many modern C++ functions and works well when you're dealing with string manipulations within the bounds of C++.
3. Memory Management and Performance
Passing by Reference vs. Value: const std::string & is passed by reference, meaning that the compiler must access the original string data location every time properties like size, capacity, or data are accessed.
std::string_view: This is passed by value. It stores a size and a pointer to the string data, enabling faster access times since there's no need to reference the actual object each time. Memory overhead is minimized as well because it does not manage capacity.
When to Use Each
Here are two scenarios where you should prefer const std::string &:
Interfacing with C legacy code: If you need to call C functions that require null-terminated strings, stick with const std::string &.
Needing to store the string: If the string needs to be stored for later use or interfacing with a specific C++ library, const std::string & keeps the full std::string functionalities intact.
For all other cases, std::string_view is generally a better option, especially when dealing with functions that only need to read the string without modifying it.
Conclusion
In summary, while both const std::string & and std::string_view provide read-only access to string data, they're not interchangeable in every context. Being aware of their differences regarding null-termination, compatibility with C functions, and performance can help optimize your code effectively. Choose wisely based on your specific application's needs to take full advantage of what C++ has to offer.
Understanding these distinctions will empower you in writing more effective, efficient, and error-free C++ code.