filmov
tv
Maps in C++ (std::map and std::unordered_map)
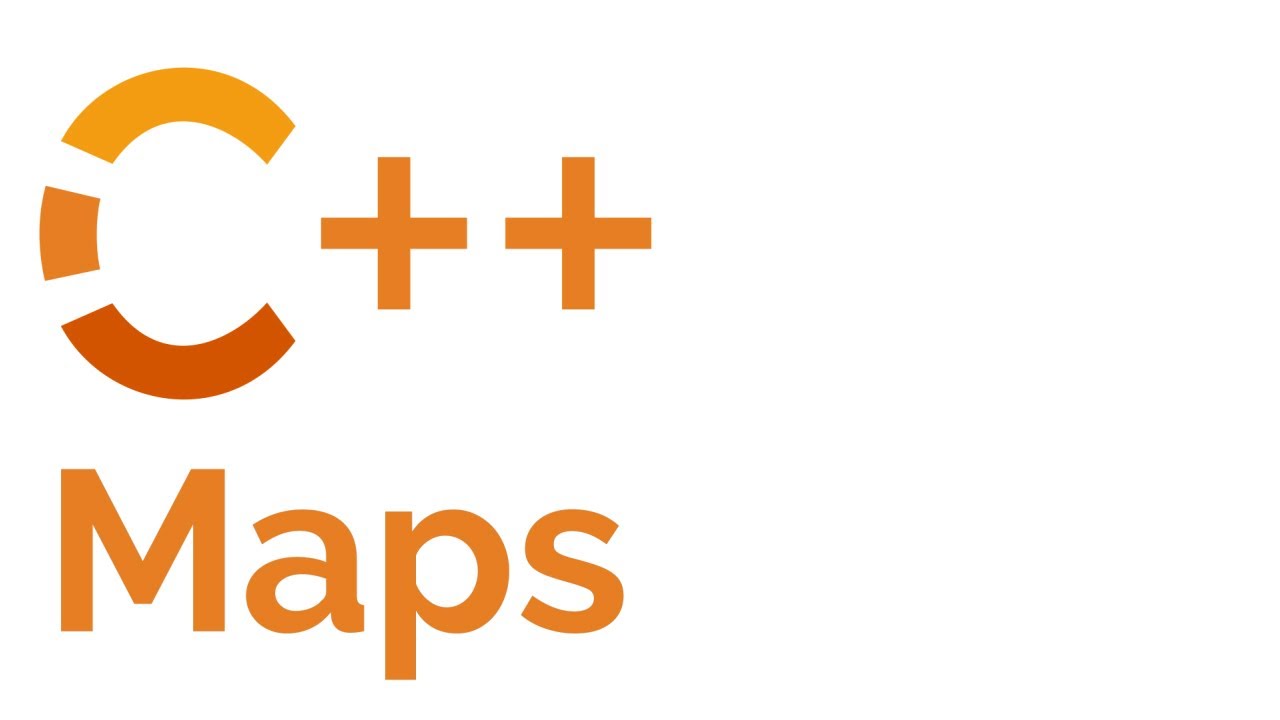
Показать описание
Chapters:
----------------
0:00 - What are maps?
3:40 - Why use maps + example usage
9:30 - Writing a hash function to use a custom type
16:50 - The [] operator
19:00 - The .at() function
20:40 - How to check if key exists in map
21:11 - How to iterate through maps
24:47 - How to remove entries from maps
25:03 - Writing a less-than operator for custom types
28:05 - Performance and which map to use
This video is sponsored by Hostinger.
Maps in C++ (std::map and std::unordered_map)
Map in C++ with practical examples - step by step Data Structures tutorial
A Guide to Maps
C++ Maps
map vs unordered map In C++
Everything about C++ STL MAPS - Part 1 | Competitive Programming Course | Episode 25
C++TUTORIAL||MAPS IN C++ ||FREQUENCY CHECKER WITH MAPS
STL std::map | Modern Cpp Series Ep. 126
C++ Programming Language Tutorial | Map in C++ STL | GeeksforGeeks
Learn C++ With Me #17 - Maps
Map In C++ STL | C++ Tutorials for Beginners #73
C++ STL Tutorial: maps
C++ Grundlagen Tutorial #050 std map
MAP | MULTIMAP | Ассоциативные контейнеры | Библиотека стандартных шаблонов (stl) | Уроки | C++ #10...
C++ Crash Course: Ordered Maps
How do dictionaries (hashmaps) actually work?
C++ Maps - map.cpp
Modern C++ - Maps
STL Maps in C++ | Standard Template Library | Data Structures
Learn Maps STL for CP | Map functions (insert, find , lower_bound , upper_bound ) | Learn C++ stl
Concept Maps using C++23 Library Tech - Indirection to APIs for a Concept - Steve Downey - C++Now 24
Maps-STL HackerRank Solution in C++
The C++ std::map Associative Container
Map | C++ STL (Standard Template Library) | std::map
Комментарии