filmov
tv
Async Generators - Javascript In Depth
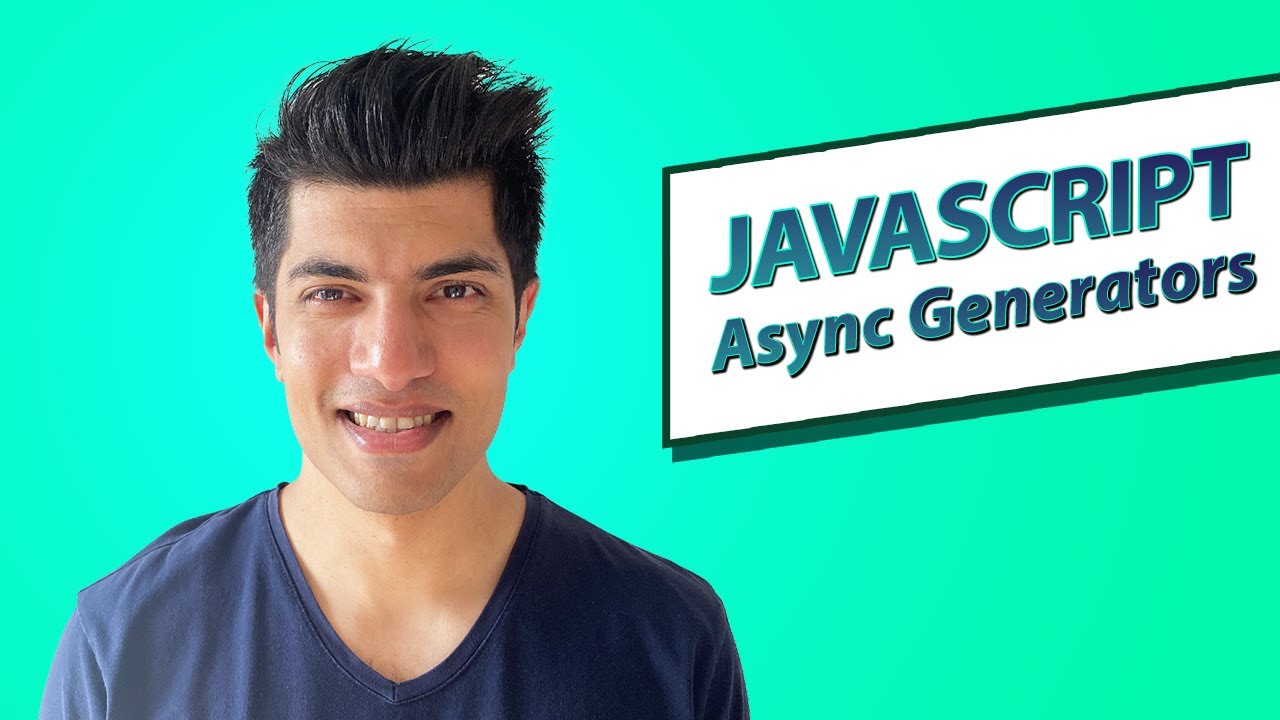
Показать описание
We take a look at Async Generators in Javascript together. This builds on the concept of synchronous generators that we looked at earlier and allow us to work with promises in generators in a consistent and easy way. We also compare these new async generators to regular generators that return promises.
We see how we can use the new for-await-of loop to easily loop through async generator objects as well.
Chapters:
00:00 Introduction
00:29 Iterable Recap
01:06 For..Of Loop Recap
01:31 Generator Recap
02:10 Generator For Of Loop
03:02 Generators and Promises
04:02 Generator Promise Code Example
17:04 Async Generator Syntax
18:06 Async Generator Code Example
25:00 Infinite Async Generators
33:16 Async Generator Return Keyword
34:20 Async Generator Yield Delegation
35:55 Next Steps
We see how we can use the new for-await-of loop to easily loop through async generator objects as well.
Chapters:
00:00 Introduction
00:29 Iterable Recap
01:06 For..Of Loop Recap
01:31 Generator Recap
02:10 Generator For Of Loop
03:02 Generators and Promises
04:02 Generator Promise Code Example
17:04 Async Generator Syntax
18:06 Async Generator Code Example
25:00 Infinite Async Generators
33:16 Async Generator Return Keyword
34:20 Async Generator Yield Delegation
35:55 Next Steps
Welcome to Async Generators in JavaScript
Learn JavaScript Generators In 12 Minutes
Using async generators to stream data in JavaScript
Asynchronous JavaScript #5 - Generators
Async Generators - Javascript In Depth
Exercises: Async Generators - Javascript In Depth
The Power of JS Generators by Anjana Vakil
JavaScript Async Generators Unleashed: Harnessing Asynchronous Power
Persisting State With Starfx In TODO App With Tauri v2
Async Generators in Real Life - London Node User Group - October 2019
From JavaScript Iterator To Async Generator (in 20 seconds)
Async generators javascript in depth
Async Iterators -Jamie McCrindle
Intro to Javascript Async/Await and Generators on Nodejs
Observable JavaScript playbook, asynchronous generators and hacking the Fun Fun Forum
Remote Online Tech Talk: How to use Generators, Iterators, and Async Functions in JavaScript
Are you bad, good, better or best with Async JS? JS Tutorial: Callbacks, Promises, Generators
JavaScript Generators Tutorial - How to Write Asynchronous JS with ES6 Generators
Javascript Generators - THEY CHANGE EVERYTHING - ES6 Generators Harmony Generators
Async Iterators & Generators - HTTP203 Advent
Next Generation Asynchronous Patterns in JavaScript - Jonathan Mills
Learning ECMAScript 2017 : Generators and Async Functions | packtpub.com
From Callbacks to Generators with Promises - Async JS
Async Iterators & Generators in JavaScript: Master Asynchronous Loops!
Комментарии