filmov
tv
Doubly Linked List (in C)
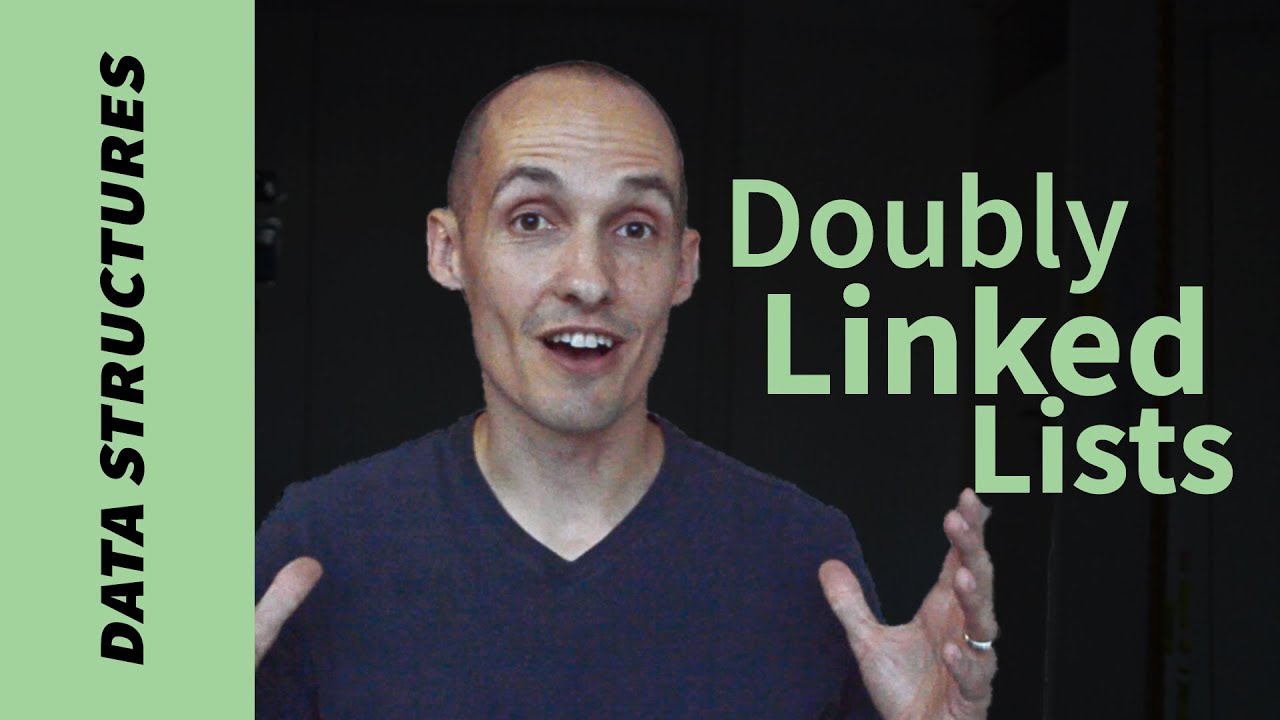
Показать описание
---
Double-linking adds an extra pointer (previous) and makes it easier to modify your linked lists or traverse them in reverse.
***
Welcome! I post videos that help you learn to program and become a more confident software developer. I cover beginner-to-advanced systems topics ranging from network programming, threads, processes, operating systems, embedded systems and others. My goal is to help you get under-the-hood and better understand how computers work and how you can use them to become stronger students and more capable professional developers.
About me: I'm a computer scientist, electrical engineer, researcher, and teacher. I specialize in embedded systems, mobile computing, sensor networks, and the Internet of Things. I teach systems and networking courses at Clemson University, where I also lead the PERSIST research lab.
More about me and what I do:
To Support the Channel:
+ like, subscribe, spread the word
Want me to review your code?
You can also find more info about code reviews here.
Double-linking adds an extra pointer (previous) and makes it easier to modify your linked lists or traverse them in reverse.
***
Welcome! I post videos that help you learn to program and become a more confident software developer. I cover beginner-to-advanced systems topics ranging from network programming, threads, processes, operating systems, embedded systems and others. My goal is to help you get under-the-hood and better understand how computers work and how you can use them to become stronger students and more capable professional developers.
About me: I'm a computer scientist, electrical engineer, researcher, and teacher. I specialize in embedded systems, mobile computing, sensor networks, and the Internet of Things. I teach systems and networking courses at Clemson University, where I also lead the PERSIST research lab.
More about me and what I do:
To Support the Channel:
+ like, subscribe, spread the word
Want me to review your code?
You can also find more info about code reviews here.
Комментарии