filmov
tv
Fixing UnboundLocalError: How to Correctly Reference Local Variables in Python Functions
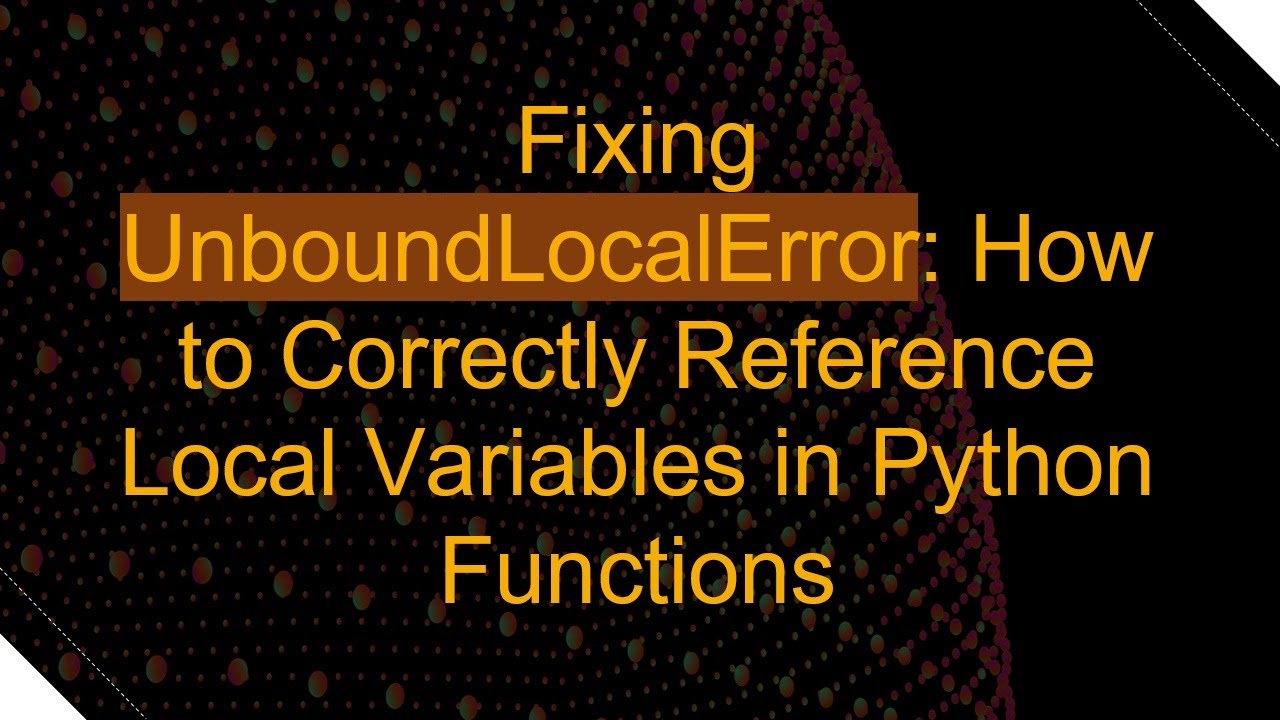
Показать описание
Learn how to resolve the 'local variable referenced before assignment' error in Python by adjusting the scope of your variables within functions.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Error- local variable referenced before assignment
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Fixing UnboundLocalError: How to Correctly Reference Local Variables in Python Functions
Programming can sometimes be frustrating, especially when dealing with seemingly simple errors. One common issue that many Python developers encounter is the UnboundLocalError, particularly when trying to use local variables. In this guide, we will explore this error and provide you with a solution to help you display the first ten lines of a CSV file using a function.
Understanding the Problem
Imagine you've written a function to read a CSV file and print out the first ten lines, but instead of the desired output, you encounter this error:
[[See Video to Reveal this Text or Code Snippet]]
This error typically suggests that a variable (in this case, rows) is being referenced before it has been defined within the local scope of the function.
The problem arises due to how Python handles variable scope. When you assign a value to a variable inside a function, Python treats that variable as local to that function, unless specified otherwise.
Your Initial Code
Let's take a look at the code causing the issue:
[[See Video to Reveal this Text or Code Snippet]]
In this code:
You define rows outside the function, but then inside the function, you try to modify rows which leads to an UnboundLocalError.
The Solution
To fix this error, you need to define rows within the scope of the function where it's being used. Here's how you can correctly implement it:
Corrected Code
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Solution
Scope of Variables:
Define rows inside the lines function to ensure it is treated as a local variable.
Incrementing rows:
Use rows + = 1 for easier readability instead of rows = rows + 1.
Condition Check:
The check with if rows >= 10: ensures the function prints only the first ten rows.
Conclusion
By restructuring how and where you define your variables, you can resolve the UnboundLocalError effectively. This solution not only addresses the current problem but also provides a better understanding of variable scope within functions.
Now you can confidently read from your CSV file and display the first ten lines without encountering any errors!
Remember, programming is a learning journey, and every error you encounter is an opportunity to become a more proficient developer.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Error- local variable referenced before assignment
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Fixing UnboundLocalError: How to Correctly Reference Local Variables in Python Functions
Programming can sometimes be frustrating, especially when dealing with seemingly simple errors. One common issue that many Python developers encounter is the UnboundLocalError, particularly when trying to use local variables. In this guide, we will explore this error and provide you with a solution to help you display the first ten lines of a CSV file using a function.
Understanding the Problem
Imagine you've written a function to read a CSV file and print out the first ten lines, but instead of the desired output, you encounter this error:
[[See Video to Reveal this Text or Code Snippet]]
This error typically suggests that a variable (in this case, rows) is being referenced before it has been defined within the local scope of the function.
The problem arises due to how Python handles variable scope. When you assign a value to a variable inside a function, Python treats that variable as local to that function, unless specified otherwise.
Your Initial Code
Let's take a look at the code causing the issue:
[[See Video to Reveal this Text or Code Snippet]]
In this code:
You define rows outside the function, but then inside the function, you try to modify rows which leads to an UnboundLocalError.
The Solution
To fix this error, you need to define rows within the scope of the function where it's being used. Here's how you can correctly implement it:
Corrected Code
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Solution
Scope of Variables:
Define rows inside the lines function to ensure it is treated as a local variable.
Incrementing rows:
Use rows + = 1 for easier readability instead of rows = rows + 1.
Condition Check:
The check with if rows >= 10: ensures the function prints only the first ten rows.
Conclusion
By restructuring how and where you define your variables, you can resolve the UnboundLocalError effectively. This solution not only addresses the current problem but also provides a better understanding of variable scope within functions.
Now you can confidently read from your CSV file and display the first ten lines without encountering any errors!
Remember, programming is a learning journey, and every error you encounter is an opportunity to become a more proficient developer.