filmov
tv
DataTypes in JS
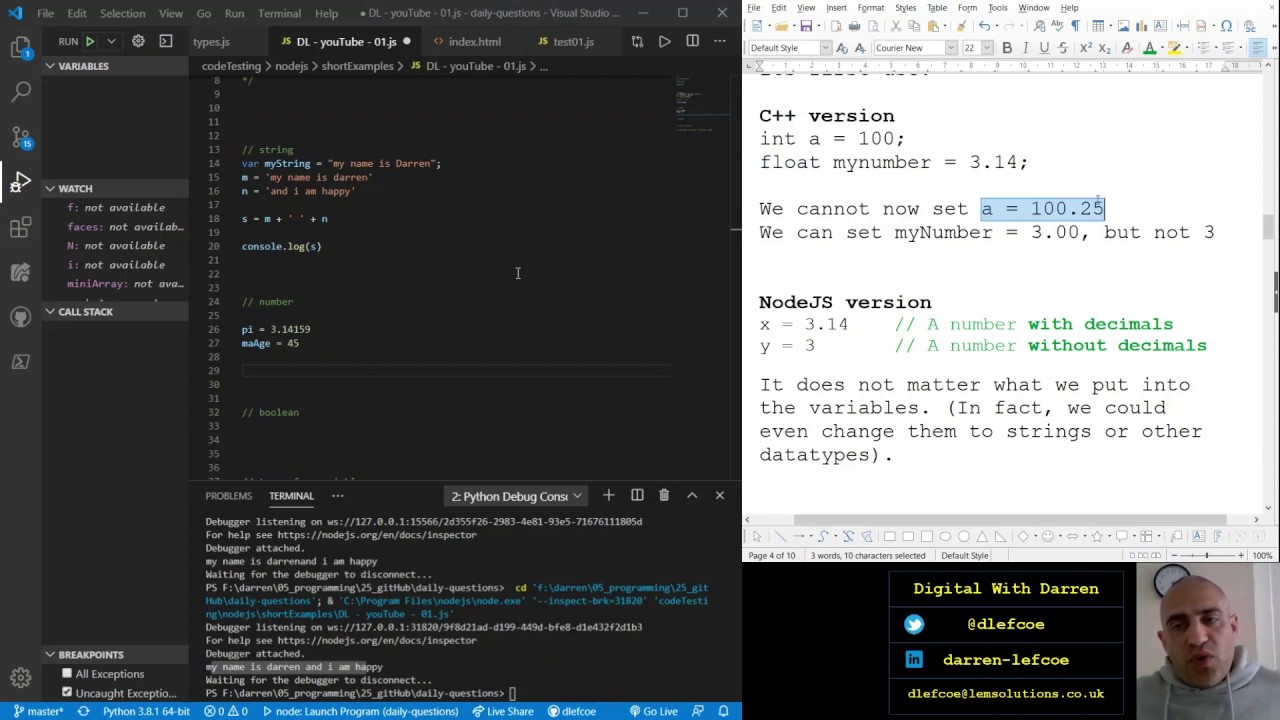
Показать описание
In this video, i will be reviewing 3 different data types in nodejs or javascript:
string
number
boolean
The difference between the types is what the variable holds.
string - this is a string of charaters.
'abcd', 'hello world', “how are you”
`the world is round`
an example of defining a string in nodejs (with full syntax) is:
var myString = ”my name is darren”;
in shorthand it is:
m = ‘my name is darren’
number - this could be an integer number, such as 1,2,3,4,10, 100, 1000, 1000000 etc..
integers can also be negatives.
-1, -100, -2000000
and the number zero:
or this number could be a floating number such as 2.5, 3.8, pi = 3.14159
and equally all the negative versions.
We declare a number in nodejs in the following way:
var pi = 3.14159;
var myAge = 45;
or in short form we could just write:
pi = 3.14159
myAge = 45
NodeJS and JavaScript is loosly-typed language.
To demonstrate what this means, we compare to a strongly-typed language, like C++ that requires every variable to be declared with its type before its first use.
C++ version
int a = 100;
float mynumber = 3.14;
We cannot now set a = 100.25
We can set myNumber = 3.00, but not 3
NodeJS version
x = 3.14 // A number with decimals
y = 3 // A number without decimals
It does not matter what we put into the variables. (In fact, we could even change them to strings or other datatypes).
This has the advantage of flexibility, but the disadvantage of errors creeping into the code where the programmer may have confused the datatype.
Incrementing a number
in the myAge example, next year, the person who is 45 will be 46. We could change this as follows:
myAge = myAge + 1
the shorthand in JS is to use:
myAge++
Floating point error
There is a floating point error in nodejs
x = 0.2 + 0.1 // x will be 0.30000000000000004
we can bypass this with the toFixed() keyword function.
but if we do:
x = x * 3 // what does this give?
then we are open to the same floating point error
We can fix this with:
x = (x * 3).toFixed(3)
This is a rather annoying feature, bt we must know that it is there.
Examples:
US Federal deficit = Trillions
10,000,000,000,000
Distance from earth to moon:
384,400,000 meters
Distance from earth to mars:
212,000,000,000 meters
with such large numbers, the floating point error might become meaningful.
boolean - this is either True or False.
A boolean variable can only take one of two states: TRUE or FALSE
in JS full syntax:
var am_i_happy = true;
in JS shorthand:
is_sky_red = false
(as a side note i have also used a different variable writing method using snake_case as opposed to camelCase).
we could bypass boolean with 2 integers, but like to distinguish.
true = 1
false = 0
Typeof() keyword
Because nodeJS is a loosely typed language, we might pass a string where we meant to pass a number.
n = 20 // this is a number
or
n = “20” // this is a string
In nodeJS or javascript, we can check the type using the typeof() keyword.
for example:
x = 10 // this is a number
xType = typeof(x)
now log to console to see result.
string
number
boolean
The difference between the types is what the variable holds.
string - this is a string of charaters.
'abcd', 'hello world', “how are you”
`the world is round`
an example of defining a string in nodejs (with full syntax) is:
var myString = ”my name is darren”;
in shorthand it is:
m = ‘my name is darren’
number - this could be an integer number, such as 1,2,3,4,10, 100, 1000, 1000000 etc..
integers can also be negatives.
-1, -100, -2000000
and the number zero:
or this number could be a floating number such as 2.5, 3.8, pi = 3.14159
and equally all the negative versions.
We declare a number in nodejs in the following way:
var pi = 3.14159;
var myAge = 45;
or in short form we could just write:
pi = 3.14159
myAge = 45
NodeJS and JavaScript is loosly-typed language.
To demonstrate what this means, we compare to a strongly-typed language, like C++ that requires every variable to be declared with its type before its first use.
C++ version
int a = 100;
float mynumber = 3.14;
We cannot now set a = 100.25
We can set myNumber = 3.00, but not 3
NodeJS version
x = 3.14 // A number with decimals
y = 3 // A number without decimals
It does not matter what we put into the variables. (In fact, we could even change them to strings or other datatypes).
This has the advantage of flexibility, but the disadvantage of errors creeping into the code where the programmer may have confused the datatype.
Incrementing a number
in the myAge example, next year, the person who is 45 will be 46. We could change this as follows:
myAge = myAge + 1
the shorthand in JS is to use:
myAge++
Floating point error
There is a floating point error in nodejs
x = 0.2 + 0.1 // x will be 0.30000000000000004
we can bypass this with the toFixed() keyword function.
but if we do:
x = x * 3 // what does this give?
then we are open to the same floating point error
We can fix this with:
x = (x * 3).toFixed(3)
This is a rather annoying feature, bt we must know that it is there.
Examples:
US Federal deficit = Trillions
10,000,000,000,000
Distance from earth to moon:
384,400,000 meters
Distance from earth to mars:
212,000,000,000 meters
with such large numbers, the floating point error might become meaningful.
boolean - this is either True or False.
A boolean variable can only take one of two states: TRUE or FALSE
in JS full syntax:
var am_i_happy = true;
in JS shorthand:
is_sky_red = false
(as a side note i have also used a different variable writing method using snake_case as opposed to camelCase).
we could bypass boolean with 2 integers, but like to distinguish.
true = 1
false = 0
Typeof() keyword
Because nodeJS is a loosely typed language, we might pass a string where we meant to pass a number.
n = 20 // this is a number
or
n = “20” // this is a string
In nodeJS or javascript, we can check the type using the typeof() keyword.
for example:
x = 10 // this is a number
xType = typeof(x)
now log to console to see result.