filmov
tv
Efficiently Combine Two Columns in a Pandas DataFrame Using numpy
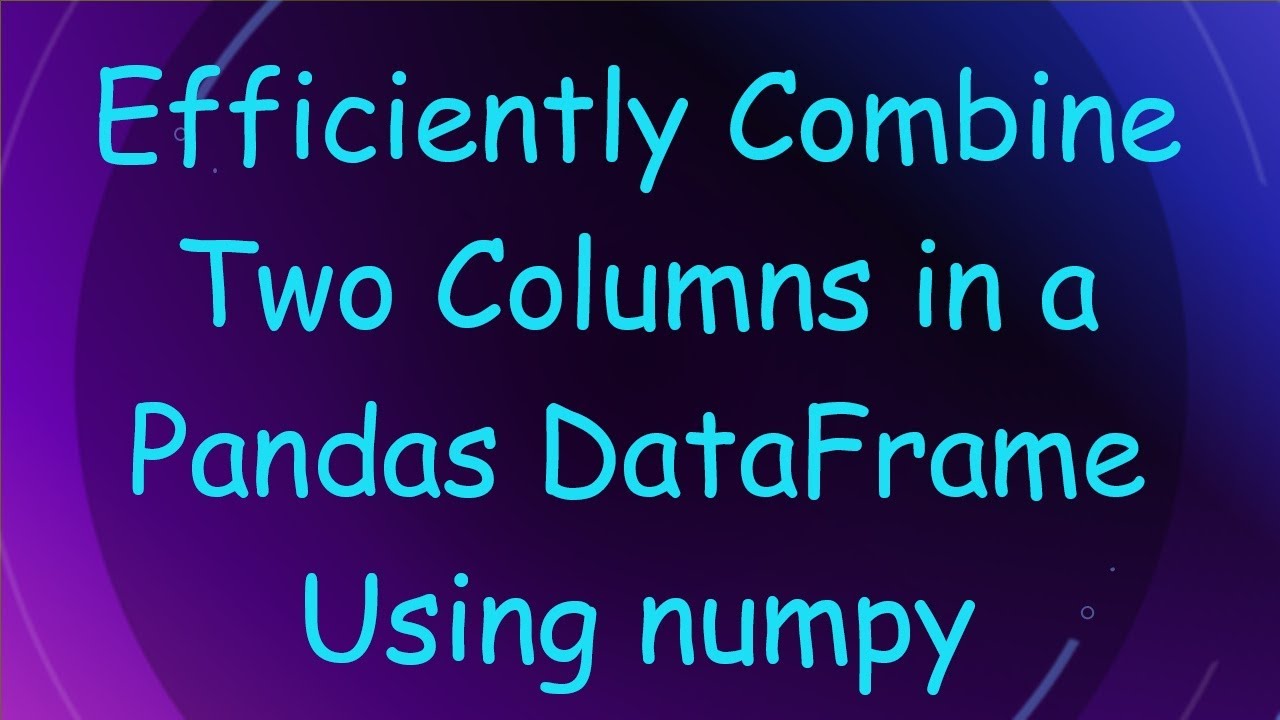
Показать описание
Discover an optimal method to combine two columns in a pandas DataFrame in an alternating order, preserving data integrity and improving efficiency.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Combine two columns in pandas dataframe but in specific order
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Efficiently Combine Two Columns in a Pandas DataFrame Using numpy
When working with large datasets in pandas, sometimes you may find yourself needing to merge two columns in a specific order. For instance, consider a DataFrame with columns containing only zeroes and ones. The challenge you're facing is to combine these columns such that you get an alternating sequence of the values rather than lumping all zeroes together followed by all ones. This guide aims to provide an efficient solution to that problem and demonstrate how you can implement it effectively using Python's pandas library.
The Problem Statement
You have a DataFrame where you want to combine two columns, "Zeroes" and "Ones". The expected output should alternate the values from both columns, resulting in an array like [0, 1, 0, 1, 0, 1] instead of the default output of [0, 0, 0, 1, 1, 1].
Why This Matters
Efficiency: If you're processing over 100,000 rows, a straightforward method may slow down your operations significantly.
Data Integrity: Maintaining the order of data while combining it is crucial for analysis.
The Solution
To combine two columns in a specific order, we'll utilize the numpy library, which is highly efficient for numerical operations. Below are the steps to achieve the desired result:
Step 1: Import Necessary Libraries
First, make sure to import the pandas library, as well as any other necessary libraries.
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Create Your DataFrame
Next, construct your DataFrame with the two columns you want to combine.
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Combine the Columns
You can now use numpy to combine both columns into a single array in an alternating order:
[[See Video to Reveal this Text or Code Snippet]]
Output
When you run the code above, you should see the following output:
[[See Video to Reveal this Text or Code Snippet]]
This shows that the combination works perfectly by alternating the zeroes and ones as desired.
Performance Comparison
If processing speed is a concern, it's good to understand how your method compares to alternatives. Below are some micro-benchmarks you can run to test the performance of different approaches:
Using numpy
[[See Video to Reveal this Text or Code Snippet]]
Average Time: 672 µs
Using List Comprehension
[[See Video to Reveal this Text or Code Snippet]]
Average Time: 2.57 ms
[[See Video to Reveal this Text or Code Snippet]]
Average Time: 2.11 ms
Conclusion
Based on the benchmarks, using numpy is significantly faster for this operation compared to list comprehensions or using itertools. This makes it the optimal choice for large datasets where performance is critical.
In summary, this guide has covered an efficient way to combine two columns in a pandas DataFrame while ensuring the values alternate as intended. Utilizing the power of numpy will help you effectively manage large datasets in your data analysis tasks. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Combine two columns in pandas dataframe but in specific order
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Efficiently Combine Two Columns in a Pandas DataFrame Using numpy
When working with large datasets in pandas, sometimes you may find yourself needing to merge two columns in a specific order. For instance, consider a DataFrame with columns containing only zeroes and ones. The challenge you're facing is to combine these columns such that you get an alternating sequence of the values rather than lumping all zeroes together followed by all ones. This guide aims to provide an efficient solution to that problem and demonstrate how you can implement it effectively using Python's pandas library.
The Problem Statement
You have a DataFrame where you want to combine two columns, "Zeroes" and "Ones". The expected output should alternate the values from both columns, resulting in an array like [0, 1, 0, 1, 0, 1] instead of the default output of [0, 0, 0, 1, 1, 1].
Why This Matters
Efficiency: If you're processing over 100,000 rows, a straightforward method may slow down your operations significantly.
Data Integrity: Maintaining the order of data while combining it is crucial for analysis.
The Solution
To combine two columns in a specific order, we'll utilize the numpy library, which is highly efficient for numerical operations. Below are the steps to achieve the desired result:
Step 1: Import Necessary Libraries
First, make sure to import the pandas library, as well as any other necessary libraries.
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Create Your DataFrame
Next, construct your DataFrame with the two columns you want to combine.
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Combine the Columns
You can now use numpy to combine both columns into a single array in an alternating order:
[[See Video to Reveal this Text or Code Snippet]]
Output
When you run the code above, you should see the following output:
[[See Video to Reveal this Text or Code Snippet]]
This shows that the combination works perfectly by alternating the zeroes and ones as desired.
Performance Comparison
If processing speed is a concern, it's good to understand how your method compares to alternatives. Below are some micro-benchmarks you can run to test the performance of different approaches:
Using numpy
[[See Video to Reveal this Text or Code Snippet]]
Average Time: 672 µs
Using List Comprehension
[[See Video to Reveal this Text or Code Snippet]]
Average Time: 2.57 ms
[[See Video to Reveal this Text or Code Snippet]]
Average Time: 2.11 ms
Conclusion
Based on the benchmarks, using numpy is significantly faster for this operation compared to list comprehensions or using itertools. This makes it the optimal choice for large datasets where performance is critical.
In summary, this guide has covered an efficient way to combine two columns in a pandas DataFrame while ensuring the values alternate as intended. Utilizing the power of numpy will help you effectively manage large datasets in your data analysis tasks. Happy coding!