filmov
tv
Sliding Window Maximum | Leetcode #239
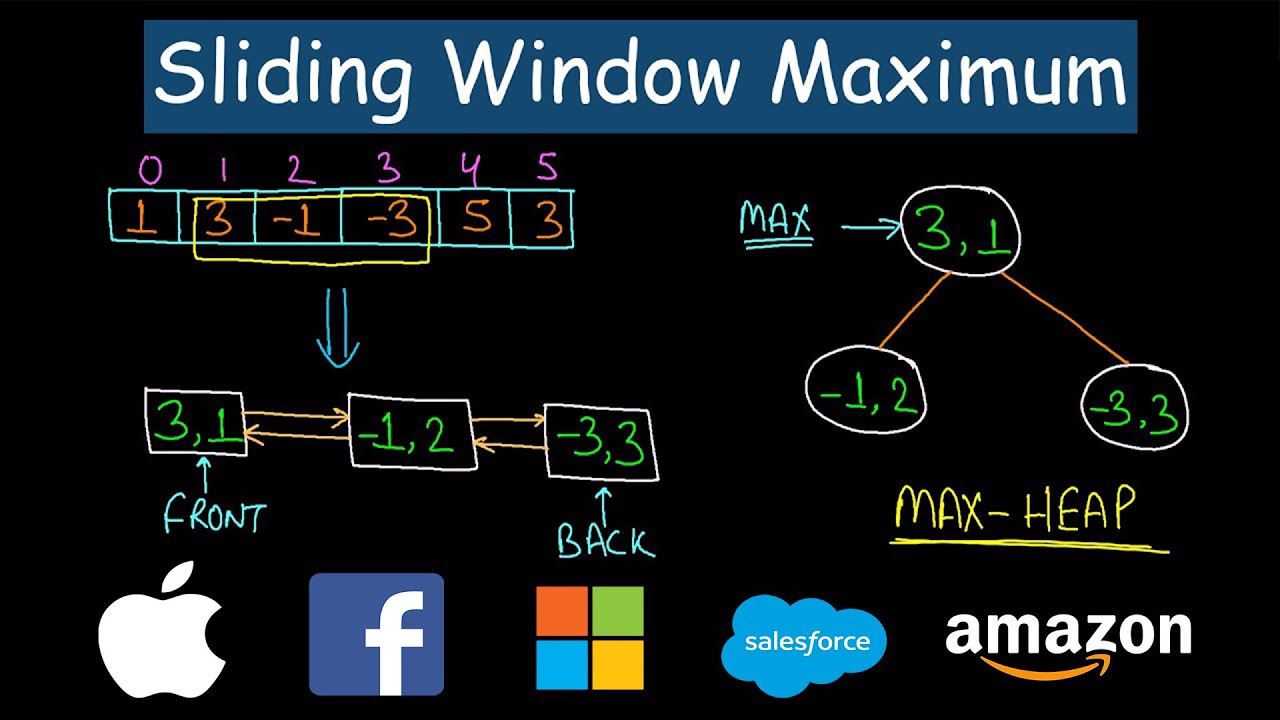
Показать описание
This video explains a very important programming interview problem which is the sliding window maximum.I have explained and compared multiple techniques for this problem from bruteforce to heap and deque.In this problem, given an array and a window size, we are required to return an array of maximum elements for all the windows of given size.First I have explained the bruteforce or naive approach and then optimized the solution using heap data structure.Finally, I have shown the most optimal solution using deque.I have shown all the required intuition using examples.I have also shown CODE for all the explained techniques at the end of the video.
CODE LINK is present below as usual. If you find any difficulty or have any query then do COMMENT below. PLEASE help our channel by SUBSCRIBING and LIKE our video if you found it helpful...CYA :)
==========================================PLEASE DONATE=============================
==============================================================================
=======================================================================
USEFUL LINKS:
RELATED LINKS:
#heap #techdose #deque
CODE LINK is present below as usual. If you find any difficulty or have any query then do COMMENT below. PLEASE help our channel by SUBSCRIBING and LIKE our video if you found it helpful...CYA :)
==========================================PLEASE DONATE=============================
==============================================================================
=======================================================================
USEFUL LINKS:
RELATED LINKS:
#heap #techdose #deque
Комментарии