filmov
tv
Resolving Python Variable Update Issues Outside of a Module: Best Practices
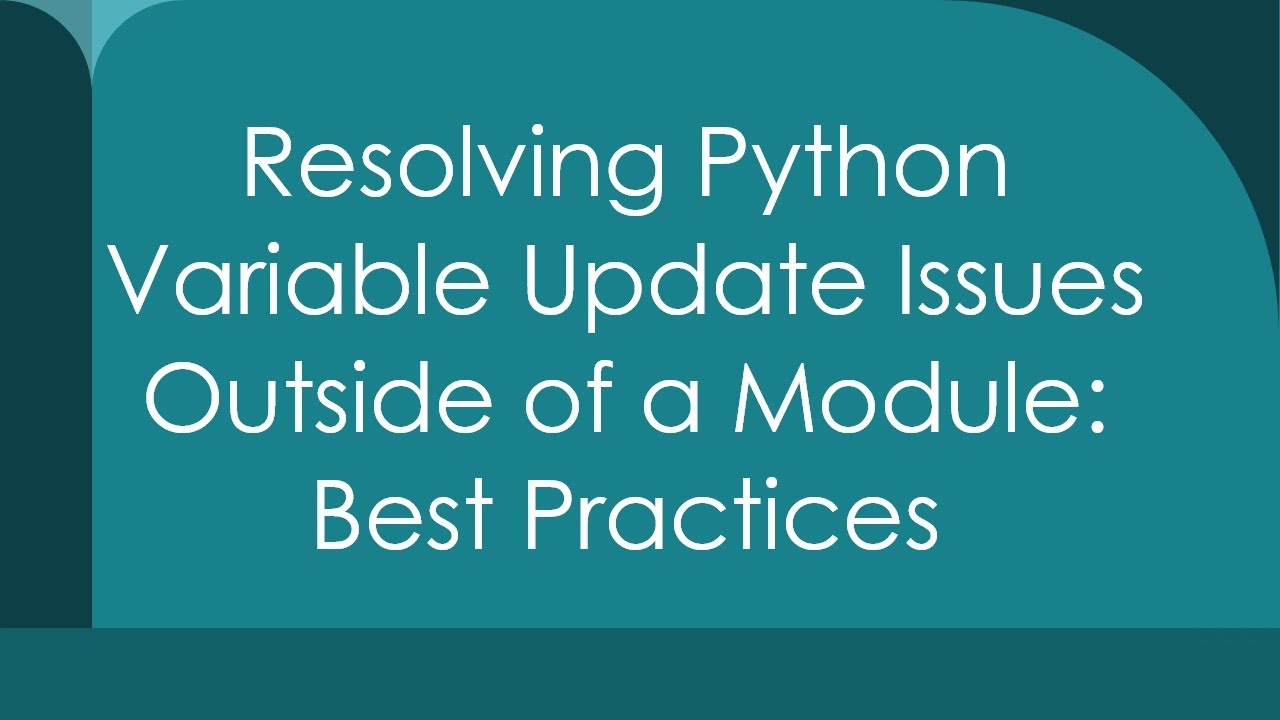
Показать описание
Discover effective methods to handle Python variable scope issues when updating values outside a module, ensuring your code runs smoothly and efficiently.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python variable won't update outside of module
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Python Variable Scope Issues
When working with Python, especially in a modular setup, developers often encounter frustrating situations where variables do not seem to maintain their updated values as expected. This dilemma can lead to unexpected behavior in applications, particularly when working with loops and functions from separate modules.
In this guide, we will explore a common scenario: a variable passed from a main script into a module that, after several iterations, fails to retain its updated state. If you are facing this issue, don’t worry – we are here to help you understand the root cause and propose effective solutions.
The Problem: Variable Not Updating
Code Snippet Overview
Here is a simplified version of the relevant code:
[[See Video to Reveal this Text or Code Snippet]]
Inside store_selector:
[[See Video to Reveal this Text or Code Snippet]]
The objective is to increment store_number as the while loop iterates through potential store selections, but this variable appears to reset after each call to store_selector.
The Solution: Strategies to Update the Variable
There are a couple of effective strategies to ensure that your store_number variable retains its updated value. Let's break them down:
Option 1: Incrementing in the Main Script
[[See Video to Reveal this Text or Code Snippet]]
This solution ensures that store_number is incremented after every complete iteration of your loop, thus updating its state as intended.
Option 2: Return the Updated Value
[[See Video to Reveal this Text or Code Snippet]]
Modified Code in store_selector:
[[See Video to Reveal this Text or Code Snippet]]
Choosing the Right Approach
While both options are valid, it’s generally advisable to choose Option 1 unless there’s a compelling reason to handle the increment within store_selector. If you are considering conditional incrementation or using the value in other parts of the function, then Option 2 could be more suitable.
Conclusion
Handling variable scope issues in Python is crucial for writing clean, efficient code. By understanding how to properly manage variable updates, you can avoid potential pitfalls that come with modular programming. Whether you choose to increment the variable in your main script or return the updated value from the function, ensure that you select the approach that best fits the logic of your application.
We hope this guide has provided clarity on resolving the problem of a non-updating variable in Python. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python variable won't update outside of module
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Python Variable Scope Issues
When working with Python, especially in a modular setup, developers often encounter frustrating situations where variables do not seem to maintain their updated values as expected. This dilemma can lead to unexpected behavior in applications, particularly when working with loops and functions from separate modules.
In this guide, we will explore a common scenario: a variable passed from a main script into a module that, after several iterations, fails to retain its updated state. If you are facing this issue, don’t worry – we are here to help you understand the root cause and propose effective solutions.
The Problem: Variable Not Updating
Code Snippet Overview
Here is a simplified version of the relevant code:
[[See Video to Reveal this Text or Code Snippet]]
Inside store_selector:
[[See Video to Reveal this Text or Code Snippet]]
The objective is to increment store_number as the while loop iterates through potential store selections, but this variable appears to reset after each call to store_selector.
The Solution: Strategies to Update the Variable
There are a couple of effective strategies to ensure that your store_number variable retains its updated value. Let's break them down:
Option 1: Incrementing in the Main Script
[[See Video to Reveal this Text or Code Snippet]]
This solution ensures that store_number is incremented after every complete iteration of your loop, thus updating its state as intended.
Option 2: Return the Updated Value
[[See Video to Reveal this Text or Code Snippet]]
Modified Code in store_selector:
[[See Video to Reveal this Text or Code Snippet]]
Choosing the Right Approach
While both options are valid, it’s generally advisable to choose Option 1 unless there’s a compelling reason to handle the increment within store_selector. If you are considering conditional incrementation or using the value in other parts of the function, then Option 2 could be more suitable.
Conclusion
Handling variable scope issues in Python is crucial for writing clean, efficient code. By understanding how to properly manage variable updates, you can avoid potential pitfalls that come with modular programming. Whether you choose to increment the variable in your main script or return the updated value from the function, ensure that you select the approach that best fits the logic of your application.
We hope this guide has provided clarity on resolving the problem of a non-updating variable in Python. Happy coding!