filmov
tv
How to Reverse String in JavaScript | Learn JavaScript & Algorithms
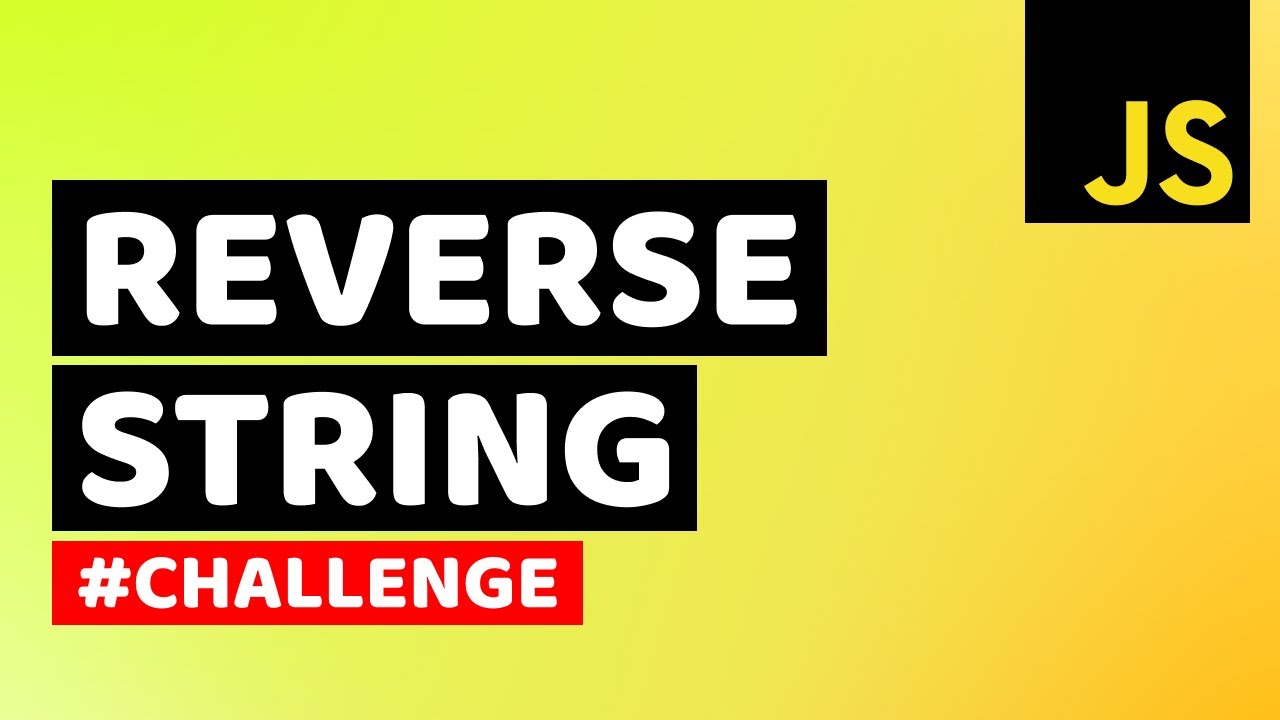
Показать описание
How to reverse string in javascript? Reversing a string is a good algorithmic challenge to solve using JavaScript. Challenge is to create a function that takes a string as an argument, and returns a new string with the reversed order of characters. There are a number of ways to reverse a string in javascript.
Define a function named "reverseString" that takes in a single parameter, "str". Use JavaScript methods such as "split", "reverse", and "join" to reverse the order of the characters in the string.
The split method is called on the input string, and it splits the string into an array of individual characters. The reverse method is then called on the resulting array, which reverses the order of the elements. The join method is called on the reversed array, which combines all the elements in the array back into a single string. Call the function with the argument, which when is passed to the function, returns reversed string.
Basically we can use reverse method to reverse the order of the elements in an array using javascript. But we are dealing with string here. That is why we split string, reverse order of string characters and then join the characters.
We can also use reduce method to reverse a string. Define a function named "reverseString" that takes in a single parameter, "str". Use JavaScript methods split, reduce to reverse the order of the characters in the string.
The reduce method is being called on the resulting array from split. It takes two parameters, a callback function and an initial value. The callback function takes two parameters, "rev" and "char" where "rev" is the accumulator which is used to store the reversed characters, and "char" is the current element of the array. The arrow function returns the concatenation of "char" and "rev" which keeps adding the characters to the "rev" in reverse order. The initial value passed to reduce is an empty string. Call the function with the argument, which when passed to the function, will return reversed string. And we have reversed string.
We can also use a for of loop to reverse a string. Define a function named "reverseString" that takes in a single parameter, "str". Declare a variable named "reversed" and assign an empty string to it.
This is a common pattern. Usually after we declare a function, immediately we declare a variable with empty strng or array. It stores the results. We return it's final value at the end of function.
Start a for-of loop. The for-of loop iterates over the characters of the input string using the "let char of str" syntax. Where str is the input string. On each iteration, the current character "char" is concatenated to the beginning of the "reversed" string by using the "+" operator. This continues for each character in the input string, and after the loop, the "reversed" variable holds the reversed version of the input string. Return the "reversed" variable. Call the function with the argument
which when passed to the function, will return reversed string.
There were three iterations. First stored, first character from string, 'c' in variable reversed. Second, 'ac' and third 'rac' because we are storing current char and last stored string in reversed variable.
At the end it became opposite of original string. Char returned at the end is r in this case. We are storing char plus string stored in reversed variable in each iteration. Value of reversed is being replaced after each iteration. It is a very interesting process. Console log it and look at it for few good seconds and you will realize the whole process.
These are few ways you can reverse a string using javascript.
* Full Playlist (Coding Challenge, Interview Questions & Leetcode) *
It can be a good javascript interview question or frontend interview question. You may not be required to solve it on paper or whiteboard but the interviewer may ask you to give an idea on how to approach this algorithm. If you have an understanding of how to solve this problem or approach this algorithm, you will be able to answer it and get your next job as a frontend developer or full-stack developer.
Thank You!
👍 LIKE VIDEO
👊 SUBSCRIBE
🔔 PRESS BELL ICON
✍️ COMMENT
#js #javascript #challenge #WebStylePress #WebDevelopment #javascriptinterviewquestions #javascripttutorial #algorithm
Define a function named "reverseString" that takes in a single parameter, "str". Use JavaScript methods such as "split", "reverse", and "join" to reverse the order of the characters in the string.
The split method is called on the input string, and it splits the string into an array of individual characters. The reverse method is then called on the resulting array, which reverses the order of the elements. The join method is called on the reversed array, which combines all the elements in the array back into a single string. Call the function with the argument, which when is passed to the function, returns reversed string.
Basically we can use reverse method to reverse the order of the elements in an array using javascript. But we are dealing with string here. That is why we split string, reverse order of string characters and then join the characters.
We can also use reduce method to reverse a string. Define a function named "reverseString" that takes in a single parameter, "str". Use JavaScript methods split, reduce to reverse the order of the characters in the string.
The reduce method is being called on the resulting array from split. It takes two parameters, a callback function and an initial value. The callback function takes two parameters, "rev" and "char" where "rev" is the accumulator which is used to store the reversed characters, and "char" is the current element of the array. The arrow function returns the concatenation of "char" and "rev" which keeps adding the characters to the "rev" in reverse order. The initial value passed to reduce is an empty string. Call the function with the argument, which when passed to the function, will return reversed string. And we have reversed string.
We can also use a for of loop to reverse a string. Define a function named "reverseString" that takes in a single parameter, "str". Declare a variable named "reversed" and assign an empty string to it.
This is a common pattern. Usually after we declare a function, immediately we declare a variable with empty strng or array. It stores the results. We return it's final value at the end of function.
Start a for-of loop. The for-of loop iterates over the characters of the input string using the "let char of str" syntax. Where str is the input string. On each iteration, the current character "char" is concatenated to the beginning of the "reversed" string by using the "+" operator. This continues for each character in the input string, and after the loop, the "reversed" variable holds the reversed version of the input string. Return the "reversed" variable. Call the function with the argument
which when passed to the function, will return reversed string.
There were three iterations. First stored, first character from string, 'c' in variable reversed. Second, 'ac' and third 'rac' because we are storing current char and last stored string in reversed variable.
At the end it became opposite of original string. Char returned at the end is r in this case. We are storing char plus string stored in reversed variable in each iteration. Value of reversed is being replaced after each iteration. It is a very interesting process. Console log it and look at it for few good seconds and you will realize the whole process.
These are few ways you can reverse a string using javascript.
* Full Playlist (Coding Challenge, Interview Questions & Leetcode) *
It can be a good javascript interview question or frontend interview question. You may not be required to solve it on paper or whiteboard but the interviewer may ask you to give an idea on how to approach this algorithm. If you have an understanding of how to solve this problem or approach this algorithm, you will be able to answer it and get your next job as a frontend developer or full-stack developer.
Thank You!
👍 LIKE VIDEO
👊 SUBSCRIBE
🔔 PRESS BELL ICON
✍️ COMMENT
#js #javascript #challenge #WebStylePress #WebDevelopment #javascriptinterviewquestions #javascripttutorial #algorithm
Комментарии