filmov
tv
Data Structures in Python: Singly Linked Lists -- Nth-to-Last Node
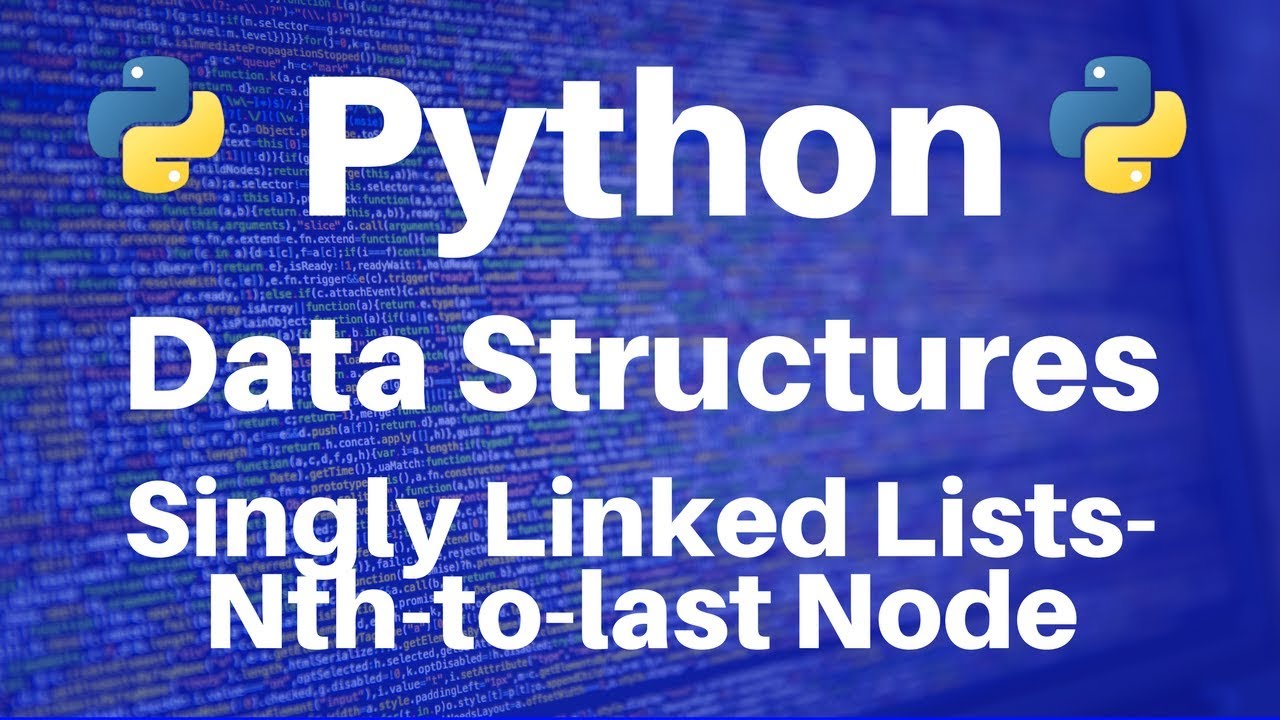
Показать описание
In this video, we investigate how to find the nth-to-last node in a singly linked list.
The software written in this video is available at:
The other video in this series mentioned is how one can calculate the length of a singly linked list:
Do you like the development environment I'm using in this video? It's a customized version of vim that's enhanced for Python development. If you want to see how I set up my vim, I have a series on this here:
If you've found this video helpful and want to stay up-to-date with the latest videos posted on this channel, please subscribe:
The software written in this video is available at:
The other video in this series mentioned is how one can calculate the length of a singly linked list:
Do you like the development environment I'm using in this video? It's a customized version of vim that's enhanced for Python development. If you want to see how I set up my vim, I have a series on this here:
If you've found this video helpful and want to stay up-to-date with the latest videos posted on this channel, please subscribe:
Data Structures in Python: Singly Linked Lists -- Insertion
Python Tutorials - Singly Linked List | Program | Part 1
Linked lists in 4 minutes
Python Data Structures #2: Linked List
Linked List - Data Structures & Algorithms Tutorials in Python #4
Recursive Data Structure in Python - Singly Linked List Introductory Tutorial
This Algorithm is SUPER HELPFUL for Coding Interviews! | Fast & Slow Pointers for Linked Lists
Data Structures in Python: Singly Linked Lists -- Length
Python course tutorials live streaming 10 hours session 322
Data Structures: Singly Linked List in Python 3 (Part 1/10)
Linked Lists - Singly & Doubly Linked - DSA Course in Python Lecture 3
Introduction to Linked List
How Insertion in Linked List Works ? 🤔😏
Learn Linked Lists in 13 minutes 🔗
Python: Linked Lists (fast)
Data Structures in Python : Singly linked list - Example, Algorithm and Code
Learning Data-structure in Python | Implementing Singly Link List
Data Structures in Python: Singly Linked Lists -- Move Tail to Head
Data Structures in Python: Singly Linked Lists -- Nth-to-Last Node
SINGLE LINKED LIST (CREATE AND DISPLAY) USING PYTHON
Data Structures in Python: Singly Linked Lists -- Rotate
Linked List in Python 🐍 with Easiest Explanation & Execution
Data Structures in Python: Singly Linked Lists -- Count Occurrences
Inserting a Node at the Beginning of a Linked List | Log2Base2®
Комментарии