filmov
tv
JavaScript filter() method in 6 minutes! 🚰
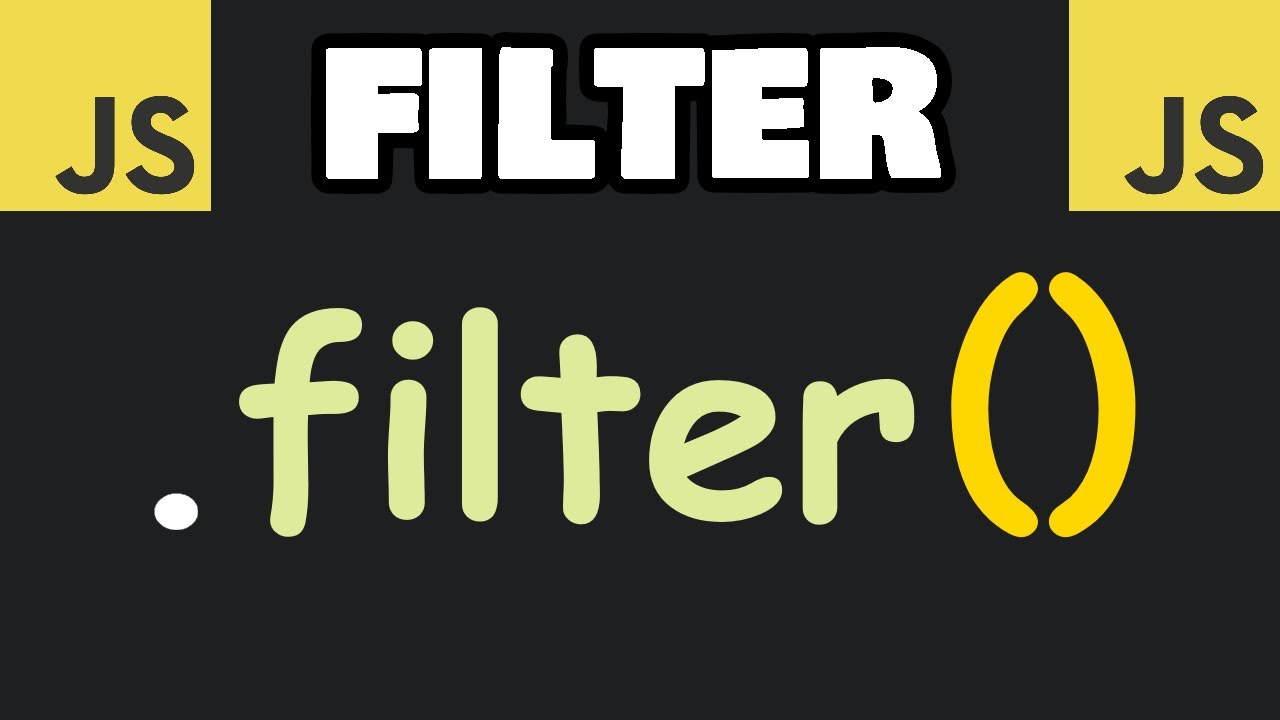
Показать описание
// .filter() = creates a new array by filtering out
// elements with a callback
00:00:00 example 1
00:02:13 example 2
00:04:01 example 3
// ----------- EXAMPLE 1 -----------
let numbers = [1, 2, 3, 4, 5, 6, 7];
function isEven(element){
return element % 2 === 0;
}
function isOdd(element){
return element % 2 !== 0;
}
// elements with a callback
00:00:00 example 1
00:02:13 example 2
00:04:01 example 3
// ----------- EXAMPLE 1 -----------
let numbers = [1, 2, 3, 4, 5, 6, 7];
function isEven(element){
return element % 2 === 0;
}
function isOdd(element){
return element % 2 !== 0;
}
JavaScript filter() method in 6 minutes! 🚰
JavaScript Array Filter
filter Array Method | JavaScript Tutorial
Filter Method | JavaScript
Javascript .filter(), .map() and .reduce() on real world examples
map, filter & reduce 🙏 Namaste JavaScript Ep. 19 🔥
Map, Filter & Reduce EXPLAINED in JavaScript - It's EASY!
How to use the Javascript Array Filter Method #Shorts
Filter out empty items in an ARRAY in a line of Code
How to use the Filter method in JavaScript to search and filter JSON data
Javascript Tutorial - Array Filter
JavaScript Pagination Tutorial #6: Search & Filter Html Table using JavaScript filter() Method
Functional JavaScript Programming 10 - Filter( ) Method (WITH EXAMPLE)
6 👌 : Use Of Map and Filter Function | Method Chaining in JavaScript || Adisma Web
JavaScript Filter Method Tutorial - Google Sheets Apps Scripts - Array Methods Part 7
Day 6: JavaScript Array Filtering - Custom Filter Function
filter method in JavaScript || JavaScript Interview Questions and Answers || filter()
Javascript filter() Metodu
Javascript Array method filter()|#tutorial 6|#coding|#programming
Filter Method - JavaScript | Es 6 | Array Methods |
What is filter() method in JavaScript | Array Methods | JavaSCript
JavaScript array filter method
Map, Filter & Reduce in JavaScript | JavaScript Tutorial in Hindi #20
#6 - Difference between map(), filter() and reduce() in Javascript
Комментарии