filmov
tv
Converting Roman Numerals to Decimal in Python
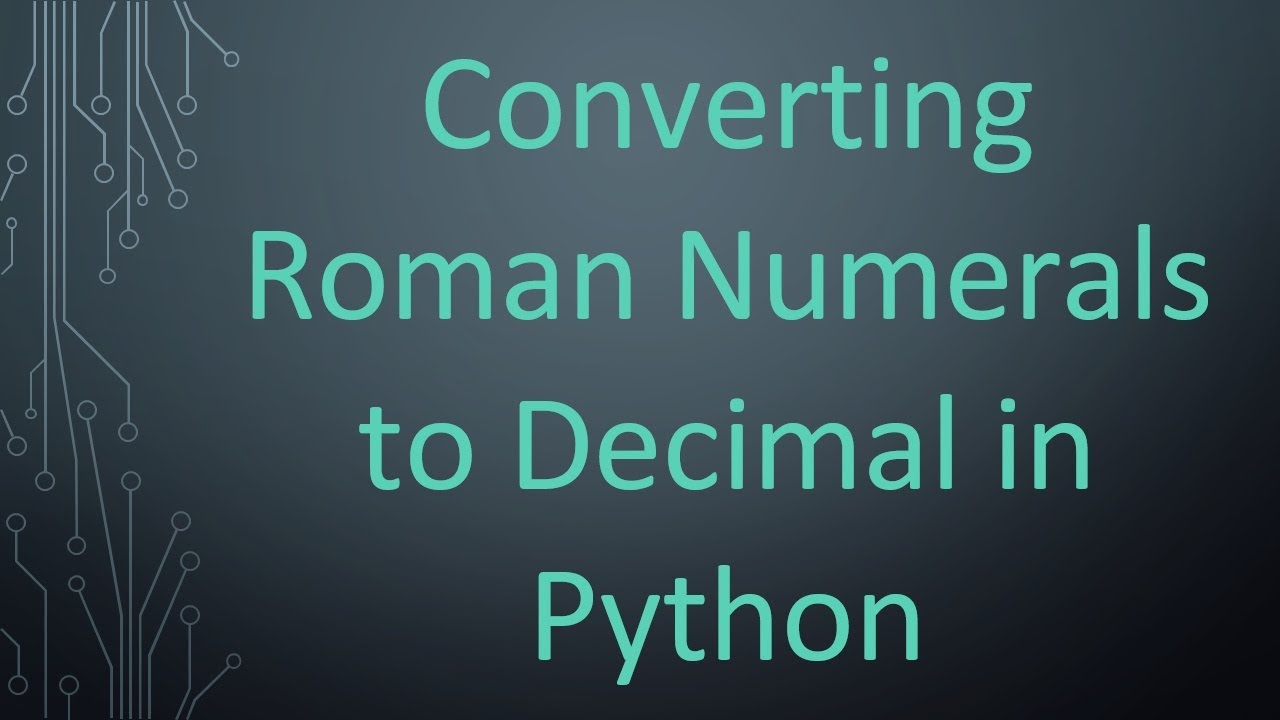
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to effectively convert Roman numerals to decimal numbers using Python, including key concepts and sample code.
---
Converting Roman Numerals to Decimal in Python
In historical contexts, Roman numerals were widely used for various purposes, ranging from marking calendar years to enumerating chapters in books. If you are dealing with data that includes Roman numerals, you might need to convert them to modern decimal numbers for easier computation and analysis. This guide will guide you on how to convert Roman numerals to numbers, specifically in Python.
Understanding Roman Numerals
Before diving into the code, let's recap the essential elements of the Roman numeral system. Roman numerals are usually composed of seven symbols:
I (1)
V (5)
X (10)
L (50)
C (100)
D (500)
M (1000)
These symbols can be combined in various ways to represent other numbers. For example, IV represents 4 (5 - 1), and VI represents 6 (5 + 1). Understanding this combination principle is key when aiming to convert Roman numerals to decimal values.
Converting Roman Numerals to Decimal in Python
To convert Roman numerals to decimal numbers in Python, you can implement a function that iterates through the Roman numeral string and sums the corresponding decimal values. Here's sample code to achieve this:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
Dictionary Lookup: A dictionary roman_values maps each Roman numeral to its respective decimal value.
Reversed Iteration: The function iterates through the Roman numeral string in reverse order. This helps in handling cases where smaller values precede larger values (e.g., IV for 4).
Conditional Summation: It compares the current value with the previous one to decide whether to add or subtract the current value.
Updating Total: Depending on the comparison, the current value is added to or subtracted from the total.
Example Usage: Finally, we verify the function by converting the Roman numeral MCMXCIV to its decimal equivalent, which is 1994.
Conclusion
Learning how to convert Roman numerals to numbers can be quite useful, especially when dealing with historical data, educational resources, or even game development. With the provided Python function, you can easily handle the conversion and integrate it into your projects.
Feel free to experiment with different Roman numeral strings and see how the conversion function performs. Happy coding!
---
Summary: Learn how to effectively convert Roman numerals to decimal numbers using Python, including key concepts and sample code.
---
Converting Roman Numerals to Decimal in Python
In historical contexts, Roman numerals were widely used for various purposes, ranging from marking calendar years to enumerating chapters in books. If you are dealing with data that includes Roman numerals, you might need to convert them to modern decimal numbers for easier computation and analysis. This guide will guide you on how to convert Roman numerals to numbers, specifically in Python.
Understanding Roman Numerals
Before diving into the code, let's recap the essential elements of the Roman numeral system. Roman numerals are usually composed of seven symbols:
I (1)
V (5)
X (10)
L (50)
C (100)
D (500)
M (1000)
These symbols can be combined in various ways to represent other numbers. For example, IV represents 4 (5 - 1), and VI represents 6 (5 + 1). Understanding this combination principle is key when aiming to convert Roman numerals to decimal values.
Converting Roman Numerals to Decimal in Python
To convert Roman numerals to decimal numbers in Python, you can implement a function that iterates through the Roman numeral string and sums the corresponding decimal values. Here's sample code to achieve this:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
Dictionary Lookup: A dictionary roman_values maps each Roman numeral to its respective decimal value.
Reversed Iteration: The function iterates through the Roman numeral string in reverse order. This helps in handling cases where smaller values precede larger values (e.g., IV for 4).
Conditional Summation: It compares the current value with the previous one to decide whether to add or subtract the current value.
Updating Total: Depending on the comparison, the current value is added to or subtracted from the total.
Example Usage: Finally, we verify the function by converting the Roman numeral MCMXCIV to its decimal equivalent, which is 1994.
Conclusion
Learning how to convert Roman numerals to numbers can be quite useful, especially when dealing with historical data, educational resources, or even game development. With the provided Python function, you can easily handle the conversion and integrate it into your projects.
Feel free to experiment with different Roman numeral strings and see how the conversion function performs. Happy coding!