filmov
tv
Return a Boolean In Java / How to Tutorial
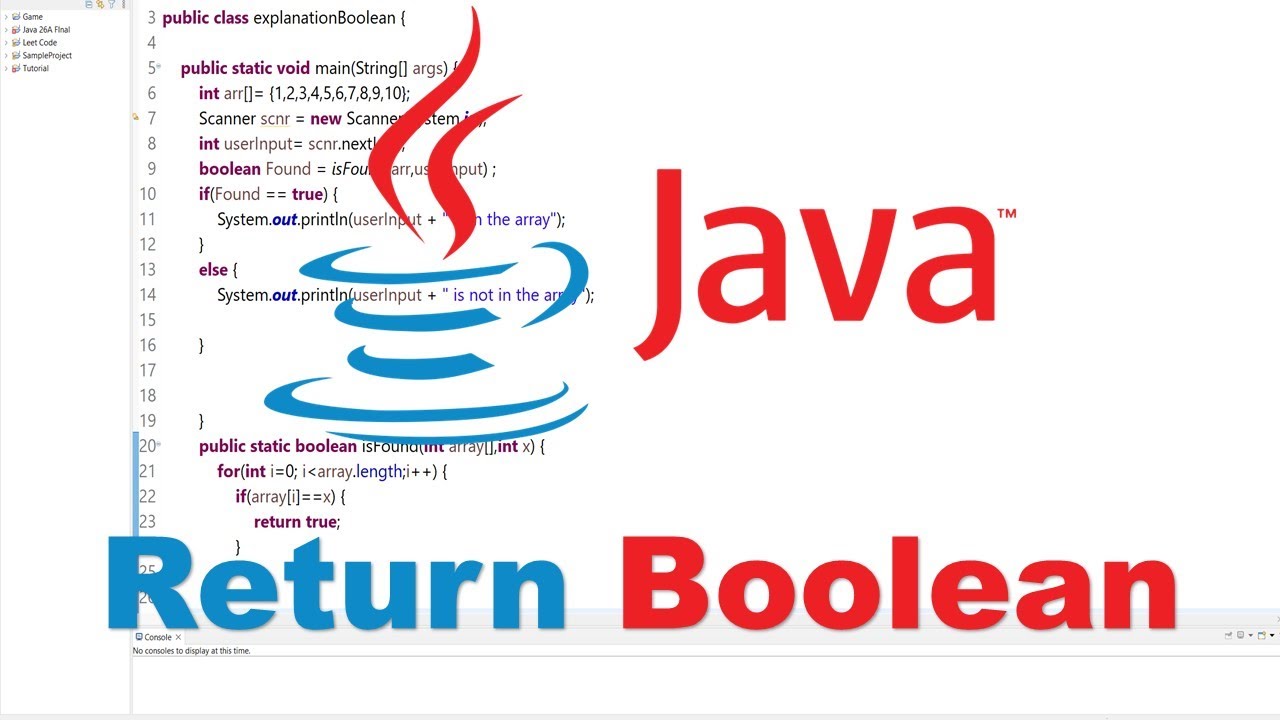
Показать описание
Objective
In this assignment you will be using array and the Scanner class. Before doing the assignment make sure
to go over the module for the week
Problem
Write a program that keeps reading in numbers from the user until the user enters ten distinct
numbers and displays the distinct numbers on the screen. Your program must filter out all the
repeated numbers and keep only one copy of it. For example, if the user enters the numbers
1, 4, 6, 1, 6, 4, 2, 1, 3,9, 6, 17, 2, 11, 45, 10 then the output of your program should be:
1, 4, 6, 2, 3, 9, 17, 11, 45, 10
Note that your program must keep looping until 10 distinct numbers has been entered.
What to turn in
You need to submit a .java file. before submitting your work
make sure that your work satisfies the criteria listed in the given rubric.
Make sure that your program generates the given sample output
Make sure that you have signed the testimony by printing your name
Make sure to write enough comments throughout your program
Requirements
The solution for the assignment must contain multiple methods. A program that has only the
main method will only get partial credit. A list of the methods and their functionality is provided.
Make sure to implement all the methods.
No global variables can be declared. Global variables are the one that are declared outside of all
the methods.
You can use the provided program shell or you can create your own program from the scratch.
Remember that you must include multiple methods in your solution.
Must provide comments throughout your code
Must have proper indentation throughout the code
Must follow the naming convention learned in the previous Java course
Java Packages
You need to import the Java util package since you will be using the Scanner class.
Methods
The following methods should be implemented in the given order.
1. public static Boolean isDistinct(int[] num, int n)
This method accepts an integer number called n and an array of integer values as its parameters. This
method scans through the array. If the content of the variable n is found in the array, the method
returns true, otherwise it returns false. You need to have a for loop to step through the array
2. Public static int getInput(int[] numbers):
This method reads the user’s input and stores them in the array called numbers. This method return the
total numbers entered by the user including the repeated ones.
Create a Scanner object
Declare an integer variable to hold the user’s input
Declare an integer variable (counter) to keep track of the total number of the numbers the user
entered.
Create a for loop and in the for loop do the followings: (for loop goes from 0 to one less than the
size of the array
Begin for loop
Prompt the user to enter an integer number
Increment the counter by 1
Declare a Boolean variable, this is needed in order to call the method found
Call the method found by passing the array and the user’s input to it. This method call will
return a boolean and the returned value needs to be stored in the boolean variable you just
declared.
If the content of the Boolean variable is true, it means the number entered by the user is
already in the array therefore the loop control variable must be decremented. If the content
of the Boolean variable is false, the user’s input must be stored in the array.
End for loop
return the counter varibale
3. public static void display(int[] num, int counter)
This method get array of integers and the total numbers of the numbers user entered. Use a for loop
to display the content of the array in the given format. Refer to the sample output. Need to use a
is in java
4. public static void main(string[] args)
This method does the following
Declare an array of integers with size 10
Declare an integer variable called counter
Call the method getInput and pass the array you declared to it. Method getInput returns an
Integer indicating the total number of the numbers that the user entered. Make sure to store
the return value in the counter variable
Call the method display and pass the array of numbers and the counter to it to display the
desired result on the screen.
Sample output
Welcome to the distinct numbers.
I will remove the repeated numbers and display the distinct numbers you entered. As long as you have
not entered 10 distinct numbers I will keep prompting you to enter a number
Enter a number: 12
Enter a number: 45
Enter a number: 56
Enter a number: 77
Enter a number: 12
Enter a number: 34
Enter a number: 77
Enter a number: 88
Enter a number: 99
Enter a number: 11
Enter a number: 45
Enter a number: 66
Enter a number: 3
You entered 13 numbers total, I filtered out all the repeated numbers.
Here is the list of distinct numbers you entered.
{12, 45, 56, 77, 34, 88, 99, 11, 66, 3}
In this assignment you will be using array and the Scanner class. Before doing the assignment make sure
to go over the module for the week
Problem
Write a program that keeps reading in numbers from the user until the user enters ten distinct
numbers and displays the distinct numbers on the screen. Your program must filter out all the
repeated numbers and keep only one copy of it. For example, if the user enters the numbers
1, 4, 6, 1, 6, 4, 2, 1, 3,9, 6, 17, 2, 11, 45, 10 then the output of your program should be:
1, 4, 6, 2, 3, 9, 17, 11, 45, 10
Note that your program must keep looping until 10 distinct numbers has been entered.
What to turn in
You need to submit a .java file. before submitting your work
make sure that your work satisfies the criteria listed in the given rubric.
Make sure that your program generates the given sample output
Make sure that you have signed the testimony by printing your name
Make sure to write enough comments throughout your program
Requirements
The solution for the assignment must contain multiple methods. A program that has only the
main method will only get partial credit. A list of the methods and their functionality is provided.
Make sure to implement all the methods.
No global variables can be declared. Global variables are the one that are declared outside of all
the methods.
You can use the provided program shell or you can create your own program from the scratch.
Remember that you must include multiple methods in your solution.
Must provide comments throughout your code
Must have proper indentation throughout the code
Must follow the naming convention learned in the previous Java course
Java Packages
You need to import the Java util package since you will be using the Scanner class.
Methods
The following methods should be implemented in the given order.
1. public static Boolean isDistinct(int[] num, int n)
This method accepts an integer number called n and an array of integer values as its parameters. This
method scans through the array. If the content of the variable n is found in the array, the method
returns true, otherwise it returns false. You need to have a for loop to step through the array
2. Public static int getInput(int[] numbers):
This method reads the user’s input and stores them in the array called numbers. This method return the
total numbers entered by the user including the repeated ones.
Create a Scanner object
Declare an integer variable to hold the user’s input
Declare an integer variable (counter) to keep track of the total number of the numbers the user
entered.
Create a for loop and in the for loop do the followings: (for loop goes from 0 to one less than the
size of the array
Begin for loop
Prompt the user to enter an integer number
Increment the counter by 1
Declare a Boolean variable, this is needed in order to call the method found
Call the method found by passing the array and the user’s input to it. This method call will
return a boolean and the returned value needs to be stored in the boolean variable you just
declared.
If the content of the Boolean variable is true, it means the number entered by the user is
already in the array therefore the loop control variable must be decremented. If the content
of the Boolean variable is false, the user’s input must be stored in the array.
End for loop
return the counter varibale
3. public static void display(int[] num, int counter)
This method get array of integers and the total numbers of the numbers user entered. Use a for loop
to display the content of the array in the given format. Refer to the sample output. Need to use a
is in java
4. public static void main(string[] args)
This method does the following
Declare an array of integers with size 10
Declare an integer variable called counter
Call the method getInput and pass the array you declared to it. Method getInput returns an
Integer indicating the total number of the numbers that the user entered. Make sure to store
the return value in the counter variable
Call the method display and pass the array of numbers and the counter to it to display the
desired result on the screen.
Sample output
Welcome to the distinct numbers.
I will remove the repeated numbers and display the distinct numbers you entered. As long as you have
not entered 10 distinct numbers I will keep prompting you to enter a number
Enter a number: 12
Enter a number: 45
Enter a number: 56
Enter a number: 77
Enter a number: 12
Enter a number: 34
Enter a number: 77
Enter a number: 88
Enter a number: 99
Enter a number: 11
Enter a number: 45
Enter a number: 66
Enter a number: 3
You entered 13 numbers total, I filtered out all the repeated numbers.
Here is the list of distinct numbers you entered.
{12, 45, 56, 77, 34, 88, 99, 11, 66, 3}