filmov
tv
Fixing the Define Function Not Returning Values Issue in Python
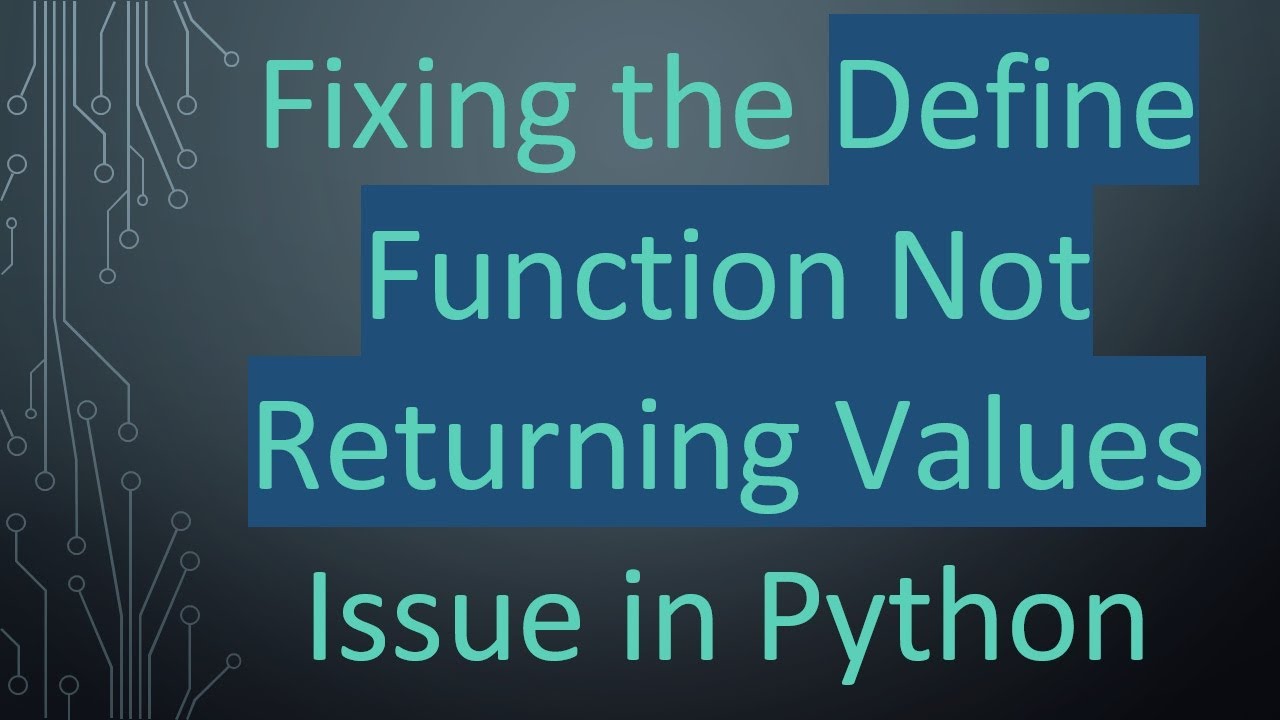
Показать описание
Learn how to solve the common issue of a defined function in Python not returning any values when executed, despite having no visible errors.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Define function not showing errors but does not return anything when function is run
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Troubleshooting Your Python Function: Why It Doesn't Return Values
Have you ever run into a situation where you've defined a Python function, but when you call it, it doesn't seem to execute the code as expected? You are left without any errors, yet without the output you anticipated. This common issue can be frustrating, especially when all you want is to see the results of your hard work. In this guide, we will explore a typical scenario involving a function that is not returning any output and how to resolve that issue.
The Problem at Hand
Imagine you've defined a function meant to output a DataFrame based on certain parameters. The expectation is that running this function would yield a well-structured DataFrame. However, upon execution, the function does not return anything, and you receive a NameError because the result variable is not defined outside the function.
This often leads developers to wonder if they are using the def function correctly. So let's break down the solution to this issue.
Solution Steps
To fix the problem of a function not returning values, follow these steps:
1. Check for Return Statement
One of the most common reasons a function doesn't return values is simply that there isn't a return statement. Without it, Python will execute the function, but it won't provide an output.
Modify your function as follows:
[[See Video to Reveal this Text or Code Snippet]]
In the example above, including return df_results at the end of the function ensures that the DataFrame is returned when the function is executed.
2. Store the Returned Value
Once you've added the return statement, it's equally important to store the returned results into a variable. This way, you can utilize the output of your function outside of its local scope.
Here’s how to do it:
[[See Video to Reveal this Text or Code Snippet]]
3. Understanding Variable Scope
When coding in Python, it's essential to remember variable scope. Variables defined inside a function have a local scope and cannot be directly accessed outside of it unless returned. Therefore, understanding how the variable scope works can help prevent NameError or undefined variable issues.
4. Final Check
After implementing the return statement and storing the output correctly, run your function again. This should resolve the issue, and you should see the expected DataFrame output.
Conclusion
In conclusion, the issue of your function not returning values usually stems from a missing return statement or a misunderstanding of variable scope. By ensuring you return your results and store them appropriately, you can effectively utilize your functions to yield data as intended.
Now it's your turn to review your functions and make sure they're returning values correctly!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Define function not showing errors but does not return anything when function is run
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Troubleshooting Your Python Function: Why It Doesn't Return Values
Have you ever run into a situation where you've defined a Python function, but when you call it, it doesn't seem to execute the code as expected? You are left without any errors, yet without the output you anticipated. This common issue can be frustrating, especially when all you want is to see the results of your hard work. In this guide, we will explore a typical scenario involving a function that is not returning any output and how to resolve that issue.
The Problem at Hand
Imagine you've defined a function meant to output a DataFrame based on certain parameters. The expectation is that running this function would yield a well-structured DataFrame. However, upon execution, the function does not return anything, and you receive a NameError because the result variable is not defined outside the function.
This often leads developers to wonder if they are using the def function correctly. So let's break down the solution to this issue.
Solution Steps
To fix the problem of a function not returning values, follow these steps:
1. Check for Return Statement
One of the most common reasons a function doesn't return values is simply that there isn't a return statement. Without it, Python will execute the function, but it won't provide an output.
Modify your function as follows:
[[See Video to Reveal this Text or Code Snippet]]
In the example above, including return df_results at the end of the function ensures that the DataFrame is returned when the function is executed.
2. Store the Returned Value
Once you've added the return statement, it's equally important to store the returned results into a variable. This way, you can utilize the output of your function outside of its local scope.
Here’s how to do it:
[[See Video to Reveal this Text or Code Snippet]]
3. Understanding Variable Scope
When coding in Python, it's essential to remember variable scope. Variables defined inside a function have a local scope and cannot be directly accessed outside of it unless returned. Therefore, understanding how the variable scope works can help prevent NameError or undefined variable issues.
4. Final Check
After implementing the return statement and storing the output correctly, run your function again. This should resolve the issue, and you should see the expected DataFrame output.
Conclusion
In conclusion, the issue of your function not returning values usually stems from a missing return statement or a misunderstanding of variable scope. By ensuring you return your results and store them appropriately, you can effectively utilize your functions to yield data as intended.
Now it's your turn to review your functions and make sure they're returning values correctly!