filmov
tv
Implement Queue using Stacks | Amortised O(1) | Leetcode 232 | Google
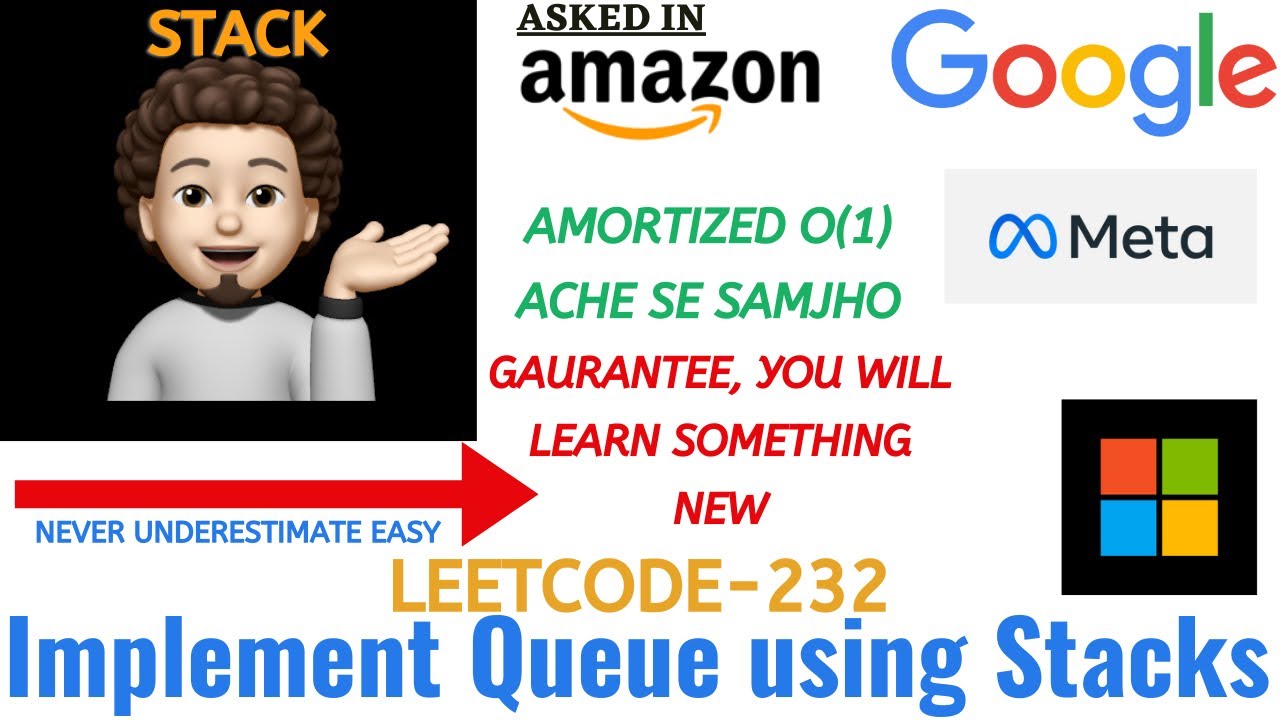
Показать описание
This is the 5th Video of our Playlist "Stack : Popular Interview Problems".In this video we will try to solve a very good and famous Stack problem.
I will explain the intuition so easily that you will never forget and start seeing this as cakewalk EASYYY.
We will do live coding after explanation and see if we are able to pass all the test cases.
Also, please note that my Github solution link below contains both C++ as well as JAVA code.
Problem Name : Implement Queue using Stacks | Amortised O(1) | Leetcode 232 | Google
Company Tags : Meta, Amazon, Netflix, Apple, Google, Amazon
Approach Summary : The solution has methods to perform common queue operations: push(int x): Adds an element to the queue. If the input stack is empty, it sets the peekEl (peek element) to the newly added element. pop(): Removes and returns the front element of the queue. If the output stack is empty, it transfers elements from the input stack to the output stack to reverse their order. The time complexity for the pop operation is amortized O(1). peek(): Returns the front element of the queue without removing it. If the output stack is empty, it returns the precomputed peekEl. empty(): Checks if the queue is empty by verifying both the input and output stacks are empty. The implementation optimizes the pop operation by transferring elements between the two stacks only when necessary, resulting in amortized constant time complexity for the pop operation.
╔═╦╗╔╦╗╔═╦═╦╦╦╦╗╔═╗
║╚╣║║║╚╣╚╣╔╣╔╣║╚╣═╣
╠╗║╚╝║║╠╗║╚╣║║║║║═╣
╚═╩══╩═╩═╩═╩╝╚╩═╩═╝
Timelines
00:00 - Introduction
#coding #helpajobseeker #easyrecipes #leetcode #leetcodequestionandanswers #leetcodesolution #leetcodedailychallenge #leetcodequestions #leetcodechallenge #hindi #india #coding #helpajobseeker #easyrecipes #leetcode #leetcodequestionandanswers #leetcodesolution #leetcodedailychallenge#leetcodequestions #leetcodechallenge #hindi #india #hindiexplanation #hindiexplained #easyexplaination #interview#interviewtips #interviewpreparation #interview_ds_algo #hinglish #github #design #data #google #video #instagram #facebook #leetcode #computerscience #leetcodesolutions #leetcodequestionandanswers #code #learning #dsalgo #dsa #2024 #newyear
I will explain the intuition so easily that you will never forget and start seeing this as cakewalk EASYYY.
We will do live coding after explanation and see if we are able to pass all the test cases.
Also, please note that my Github solution link below contains both C++ as well as JAVA code.
Problem Name : Implement Queue using Stacks | Amortised O(1) | Leetcode 232 | Google
Company Tags : Meta, Amazon, Netflix, Apple, Google, Amazon
Approach Summary : The solution has methods to perform common queue operations: push(int x): Adds an element to the queue. If the input stack is empty, it sets the peekEl (peek element) to the newly added element. pop(): Removes and returns the front element of the queue. If the output stack is empty, it transfers elements from the input stack to the output stack to reverse their order. The time complexity for the pop operation is amortized O(1). peek(): Returns the front element of the queue without removing it. If the output stack is empty, it returns the precomputed peekEl. empty(): Checks if the queue is empty by verifying both the input and output stacks are empty. The implementation optimizes the pop operation by transferring elements between the two stacks only when necessary, resulting in amortized constant time complexity for the pop operation.
╔═╦╗╔╦╗╔═╦═╦╦╦╦╗╔═╗
║╚╣║║║╚╣╚╣╔╣╔╣║╚╣═╣
╠╗║╚╝║║╠╗║╚╣║║║║║═╣
╚═╩══╩═╩═╩═╩╝╚╩═╩═╝
Timelines
00:00 - Introduction
#coding #helpajobseeker #easyrecipes #leetcode #leetcodequestionandanswers #leetcodesolution #leetcodedailychallenge #leetcodequestions #leetcodechallenge #hindi #india #coding #helpajobseeker #easyrecipes #leetcode #leetcodequestionandanswers #leetcodesolution #leetcodedailychallenge#leetcodequestions #leetcodechallenge #hindi #india #hindiexplanation #hindiexplained #easyexplaination #interview#interviewtips #interviewpreparation #interview_ds_algo #hinglish #github #design #data #google #video #instagram #facebook #leetcode #computerscience #leetcodesolutions #leetcodequestionandanswers #code #learning #dsalgo #dsa #2024 #newyear
Комментарии