filmov
tv
Generate All Possible Combinations from a Cell Array in Matlab Using Recursion
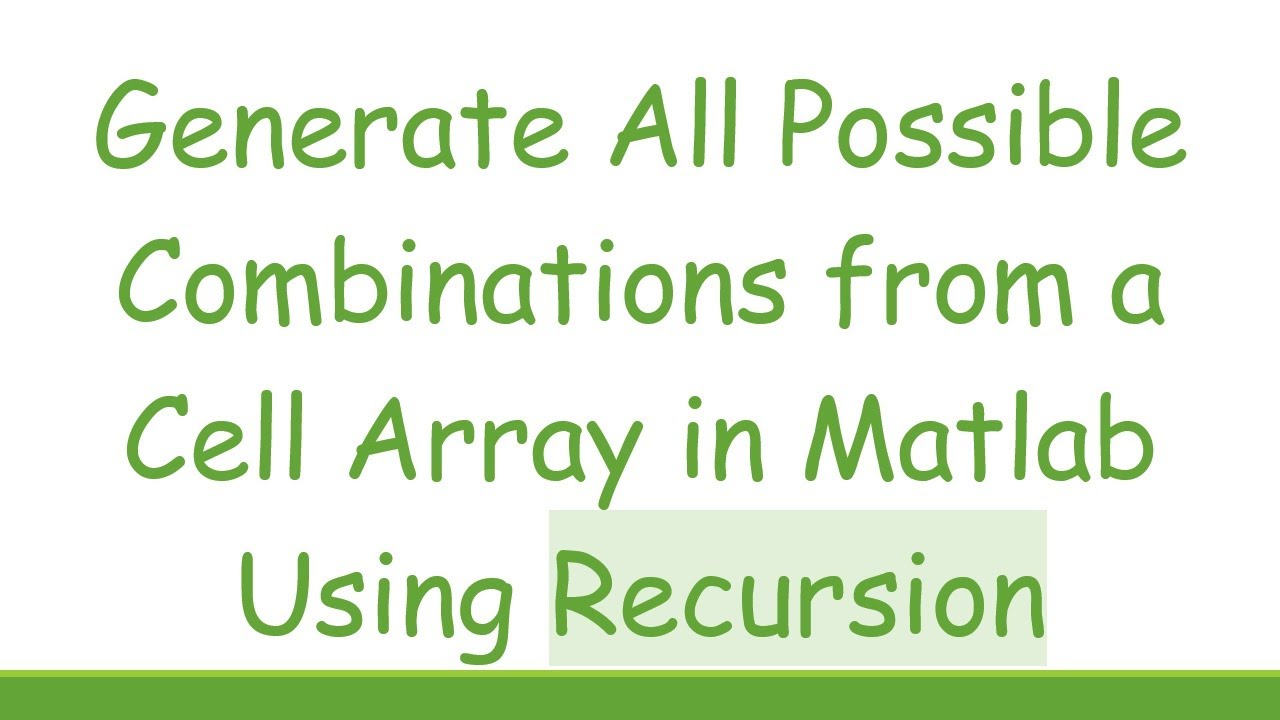
Показать описание
Learn how to create a recursive function in Matlab to list every possible combination of elements from a cell array of vectors effectively.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Recursive function to list every element from cell arrays in Matlab
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Generating All Possible Combinations from a Cell Array in Matlab
If you work with arrays in Matlab, you may come across a situation where you need to generate all possible combinations of elements from a cell array comprised of vectors. This is particularly useful in situations where you are dealing with multi-dimensional data, and you need to navigate through various combinations to derive meaningful insights. Let’s walk through how to tackle this challenge using recursion in Matlab.
The Problem Statement
Consider a cell array defined as follows:
[[See Video to Reveal this Text or Code Snippet]]
From this array, we want to generate all combinations of elements such that the output would look similar to this:
[[See Video to Reveal this Text or Code Snippet]]
Notably, this means we need to include one element from each vector in the array while creating all possible combinations.
The Recursive Solution
To obtain these combinations, we will use a recursive function that processes the cell array step by step. Here’s how to approach the solution:
Step 1: Define the Base Case
When working with recursion, it's essential to define a base case. If the cell array contains only one element, the combinations available will simply be the elements of that array converted into a cell format:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Recursive Combination Logic
Next, we will extract elements from the first vector and recursively call the function to obtain the combinations of the remaining vectors:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Combine Results
Once we have both sets of elements, we can pair them together to form all combinations:
[[See Video to Reveal this Text or Code Snippet]]
Full Function Implementation
Below is the complete function based on our structured approach:
[[See Video to Reveal this Text or Code Snippet]]
Additional Considerations
Performance: Using numel instead of length is generally more efficient. It allows the function to work seamlessly even if the input arrays are multi-dimensional.
Error Handling: It’s advisable to enhance the function by testing if the input is indeed a cell array and that each element within it is a numeric array to avoid runtime errors.
Conclusion
By utilizing recursion, we can effectively generate all possible combinations from cell arrays in Matlab. With this approach, users can efficiently manipulate and analyze multi-dimensional data sets with ease. This technique stands as a crucial tool for Matlab programmers dealing with complex data structures. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Recursive function to list every element from cell arrays in Matlab
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Generating All Possible Combinations from a Cell Array in Matlab
If you work with arrays in Matlab, you may come across a situation where you need to generate all possible combinations of elements from a cell array comprised of vectors. This is particularly useful in situations where you are dealing with multi-dimensional data, and you need to navigate through various combinations to derive meaningful insights. Let’s walk through how to tackle this challenge using recursion in Matlab.
The Problem Statement
Consider a cell array defined as follows:
[[See Video to Reveal this Text or Code Snippet]]
From this array, we want to generate all combinations of elements such that the output would look similar to this:
[[See Video to Reveal this Text or Code Snippet]]
Notably, this means we need to include one element from each vector in the array while creating all possible combinations.
The Recursive Solution
To obtain these combinations, we will use a recursive function that processes the cell array step by step. Here’s how to approach the solution:
Step 1: Define the Base Case
When working with recursion, it's essential to define a base case. If the cell array contains only one element, the combinations available will simply be the elements of that array converted into a cell format:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Recursive Combination Logic
Next, we will extract elements from the first vector and recursively call the function to obtain the combinations of the remaining vectors:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Combine Results
Once we have both sets of elements, we can pair them together to form all combinations:
[[See Video to Reveal this Text or Code Snippet]]
Full Function Implementation
Below is the complete function based on our structured approach:
[[See Video to Reveal this Text or Code Snippet]]
Additional Considerations
Performance: Using numel instead of length is generally more efficient. It allows the function to work seamlessly even if the input arrays are multi-dimensional.
Error Handling: It’s advisable to enhance the function by testing if the input is indeed a cell array and that each element within it is a numeric array to avoid runtime errors.
Conclusion
By utilizing recursion, we can effectively generate all possible combinations from cell arrays in Matlab. With this approach, users can efficiently manipulate and analyze multi-dimensional data sets with ease. This technique stands as a crucial tool for Matlab programmers dealing with complex data structures. Happy coding!