filmov
tv
Find Range of Data types in C - C Programming Tutorial 11
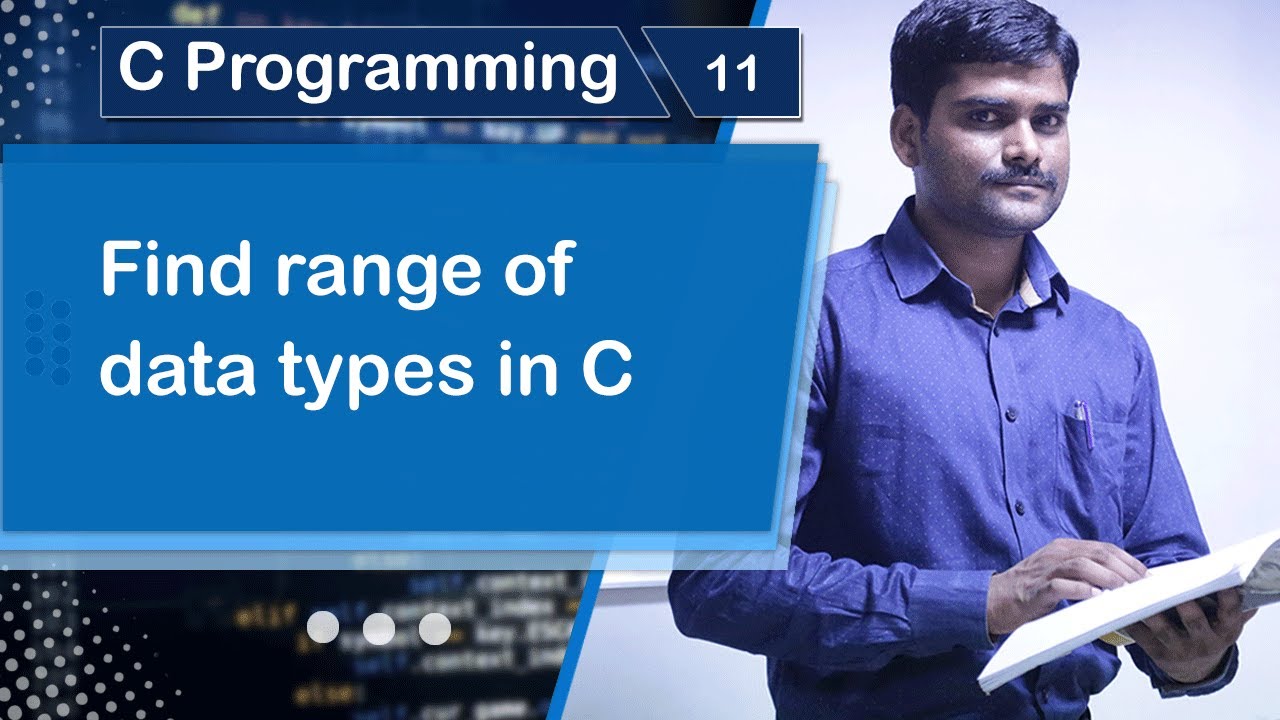
Показать описание
Notes for You:: Find Range of Data types in C - C Programming Tutorial 11
- The data type also indicates the possible range of values can be stored in a specific type of a variable or a constant.
1. int: 4 or 2 bytes : (-32,768 to 32,767 ) or (-2,147,483,648 to 2,147,483,647).
2. float : 4 bytes : 1.2E-38 to 3.4E+38.
3. double: 8 bytes : 2.3E-308 to 1.7E+308.
4. char: 1 byte : -128 to 127.
5. void: 1 or 0 byte : no value or nothing.
Note:
1 byte = 8 bits, 2 bytes = 16 bits, 4 bytes = 32 bits, 8 bytes = 64 bits.
Example Code:
#include <stdio.h>
#include<limits.h>
#include<float.h>
int main()
{
printf("int min=%i\n",INT_MIN);// =-2147483648
printf("int max=%i\n",INT_MAX);// 2147483647
printf("float min=%e\n",FLT_MIN);// 1.175494e-038
printf("float max=%e\n",FLT_MAX);// 3.402823e+038
printf("double min=%e\n",DBL_MIN);// 2.225074e-308
printf("double max=%e\n",DBL_MAX);// 1.797693e+308
printf("char min=%i\n",CHAR_MIN);// -128
printf("char max=%i\n",CHAR_MAX);//127
printf("float precision=%i\n",FLT_DIG);//6
printf("double precision=%i\n",DBL_DIG);//15
printf("long double precision=%i\n",LDBL_DIG);//18
return 0;
}
Note:
- replace < with less-than symbol.
- replace > with greater-than symbol.
=========================================
In the next C programming tutorial you understand; What are Data type Modifiers in C programming language. You understand the short, long, signed and unsigned Data type Modifiers in C language.
In the previous C programming tutorial you understand; C Program to find the Size of int, float, double, char Data types in C language, What is the use of sizeof() function in C language, etc.
=========================================
C Programming Tutorials Playlist:
=========================================
Watch My Other Useful Tutorials:-
Computer Programming Fundamentals Playlist:-
C Practical LAB Exercises Playlist:-
C++ Tutorials Playlist:
=========================================
► Subscribe to our YouTube channel:
► Visit our Website:
=========================================
Hash Tags:-
#ChidresTechTutorials #CProgramming #CProgrammingTutorial
- The data type also indicates the possible range of values can be stored in a specific type of a variable or a constant.
1. int: 4 or 2 bytes : (-32,768 to 32,767 ) or (-2,147,483,648 to 2,147,483,647).
2. float : 4 bytes : 1.2E-38 to 3.4E+38.
3. double: 8 bytes : 2.3E-308 to 1.7E+308.
4. char: 1 byte : -128 to 127.
5. void: 1 or 0 byte : no value or nothing.
Note:
1 byte = 8 bits, 2 bytes = 16 bits, 4 bytes = 32 bits, 8 bytes = 64 bits.
Example Code:
#include <stdio.h>
#include<limits.h>
#include<float.h>
int main()
{
printf("int min=%i\n",INT_MIN);// =-2147483648
printf("int max=%i\n",INT_MAX);// 2147483647
printf("float min=%e\n",FLT_MIN);// 1.175494e-038
printf("float max=%e\n",FLT_MAX);// 3.402823e+038
printf("double min=%e\n",DBL_MIN);// 2.225074e-308
printf("double max=%e\n",DBL_MAX);// 1.797693e+308
printf("char min=%i\n",CHAR_MIN);// -128
printf("char max=%i\n",CHAR_MAX);//127
printf("float precision=%i\n",FLT_DIG);//6
printf("double precision=%i\n",DBL_DIG);//15
printf("long double precision=%i\n",LDBL_DIG);//18
return 0;
}
Note:
- replace < with less-than symbol.
- replace > with greater-than symbol.
=========================================
In the next C programming tutorial you understand; What are Data type Modifiers in C programming language. You understand the short, long, signed and unsigned Data type Modifiers in C language.
In the previous C programming tutorial you understand; C Program to find the Size of int, float, double, char Data types in C language, What is the use of sizeof() function in C language, etc.
=========================================
C Programming Tutorials Playlist:
=========================================
Watch My Other Useful Tutorials:-
Computer Programming Fundamentals Playlist:-
C Practical LAB Exercises Playlist:-
C++ Tutorials Playlist:
=========================================
► Subscribe to our YouTube channel:
► Visit our Website:
=========================================
Hash Tags:-
#ChidresTechTutorials #CProgramming #CProgrammingTutorial
Комментарии