filmov
tv
Streamlining JavaScript with Arrays: A Better Way to Handle Long if Blocks
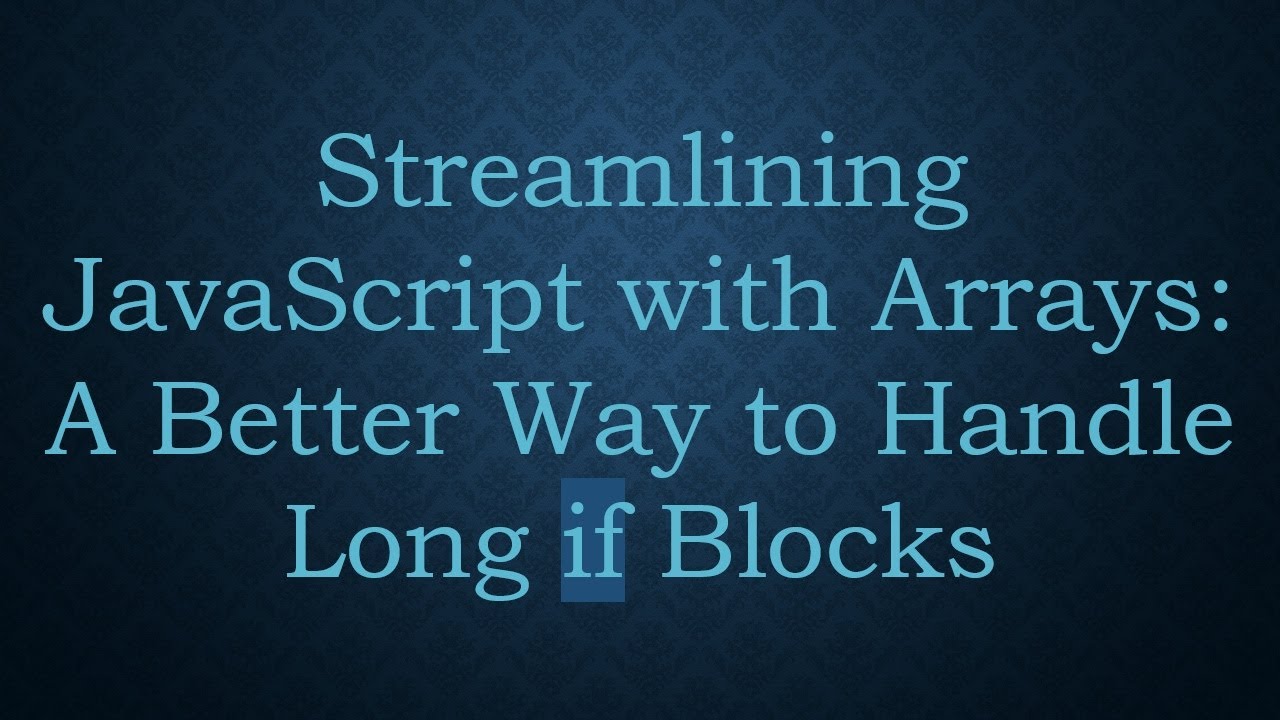
Показать описание
Discover how to optimize long JavaScript `if` blocks by using arrays and objects for cleaner, more efficient code!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Is there a better way to write out long JavaScript "if" blocks
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Streamlining JavaScript with Arrays: A Better Way to Handle Long if Blocks
As developers, we often find ourselves wrestling with repetitive patterns in our code—especially when dealing with conditional statements. In this guide, we’re diving into a common problem faced by many newcomers to JavaScript: how to efficiently write long if blocks.
The Problem: Long if Statements in JavaScript
Consider the following example where multiple if statements are used to set parameters based on URL parameters:
[[See Video to Reveal this Text or Code Snippet]]
While this code accomplishes the task, it appears inefficient and cumbersome—especially when more parameters need to be added. Not to mention, having repetitive blocks of code can make maintenance and readability more challenging.
The Solution: Using Arrays and Objects for Cleaner Code
Fortunately, there is a more efficient way to handle this scenario by leveraging arrays and objects. The goal is to collect all the parameter names in an array and then iterate through that array, allowing us to dynamically check for the presence of each parameter and store it in an object.
Step-by-Step Breakdown
Array of Allowed Parameters
First, we create an array that contains the names of the parameters we want to check:
[[See Video to Reveal this Text or Code Snippet]]
Create an Object for Parsed Parameters
Then, we set up an empty object to hold the parsed values:
[[See Video to Reveal this Text or Code Snippet]]
Iterate Over the Array
We can use the forEach method to loop through each parameter name in the allowedParameters array. For each parameter, we check if it exists in the URL parameters. If it does, we retrieve its value, parse it to an integer, and store it in the parsedParameters object:
[[See Video to Reveal this Text or Code Snippet]]
Using the Parsed Parameters
Now that we have our parameters stored in an object, accessing them is straightforward:
[[See Video to Reveal this Text or Code Snippet]]
Benefits of This Approach
Clean and Concise Code: Reduces redundancy and keeps your code neat.
Flexibility: Easily scalable by adding more parameters to the array.
Maintainability: Simplifies future edits and debugging.
Wrap-Up
By converting long if blocks into a more manageable structure using arrays and objects in JavaScript, you can dramatically improve the readability and efficiency of your code. This approach is particularly useful for those working with dynamic parameters, such as URL query strings.
With this streamlined technique, you can not only enhance your programming skills but also foster habits that lead to cleaner, more professional code.
Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Is there a better way to write out long JavaScript "if" blocks
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Streamlining JavaScript with Arrays: A Better Way to Handle Long if Blocks
As developers, we often find ourselves wrestling with repetitive patterns in our code—especially when dealing with conditional statements. In this guide, we’re diving into a common problem faced by many newcomers to JavaScript: how to efficiently write long if blocks.
The Problem: Long if Statements in JavaScript
Consider the following example where multiple if statements are used to set parameters based on URL parameters:
[[See Video to Reveal this Text or Code Snippet]]
While this code accomplishes the task, it appears inefficient and cumbersome—especially when more parameters need to be added. Not to mention, having repetitive blocks of code can make maintenance and readability more challenging.
The Solution: Using Arrays and Objects for Cleaner Code
Fortunately, there is a more efficient way to handle this scenario by leveraging arrays and objects. The goal is to collect all the parameter names in an array and then iterate through that array, allowing us to dynamically check for the presence of each parameter and store it in an object.
Step-by-Step Breakdown
Array of Allowed Parameters
First, we create an array that contains the names of the parameters we want to check:
[[See Video to Reveal this Text or Code Snippet]]
Create an Object for Parsed Parameters
Then, we set up an empty object to hold the parsed values:
[[See Video to Reveal this Text or Code Snippet]]
Iterate Over the Array
We can use the forEach method to loop through each parameter name in the allowedParameters array. For each parameter, we check if it exists in the URL parameters. If it does, we retrieve its value, parse it to an integer, and store it in the parsedParameters object:
[[See Video to Reveal this Text or Code Snippet]]
Using the Parsed Parameters
Now that we have our parameters stored in an object, accessing them is straightforward:
[[See Video to Reveal this Text or Code Snippet]]
Benefits of This Approach
Clean and Concise Code: Reduces redundancy and keeps your code neat.
Flexibility: Easily scalable by adding more parameters to the array.
Maintainability: Simplifies future edits and debugging.
Wrap-Up
By converting long if blocks into a more manageable structure using arrays and objects in JavaScript, you can dramatically improve the readability and efficiency of your code. This approach is particularly useful for those working with dynamic parameters, such as URL query strings.
With this streamlined technique, you can not only enhance your programming skills but also foster habits that lead to cleaner, more professional code.
Happy coding!