filmov
tv
How to Dynamically Link Property Types in Kotlin: A Guide to Using typealias
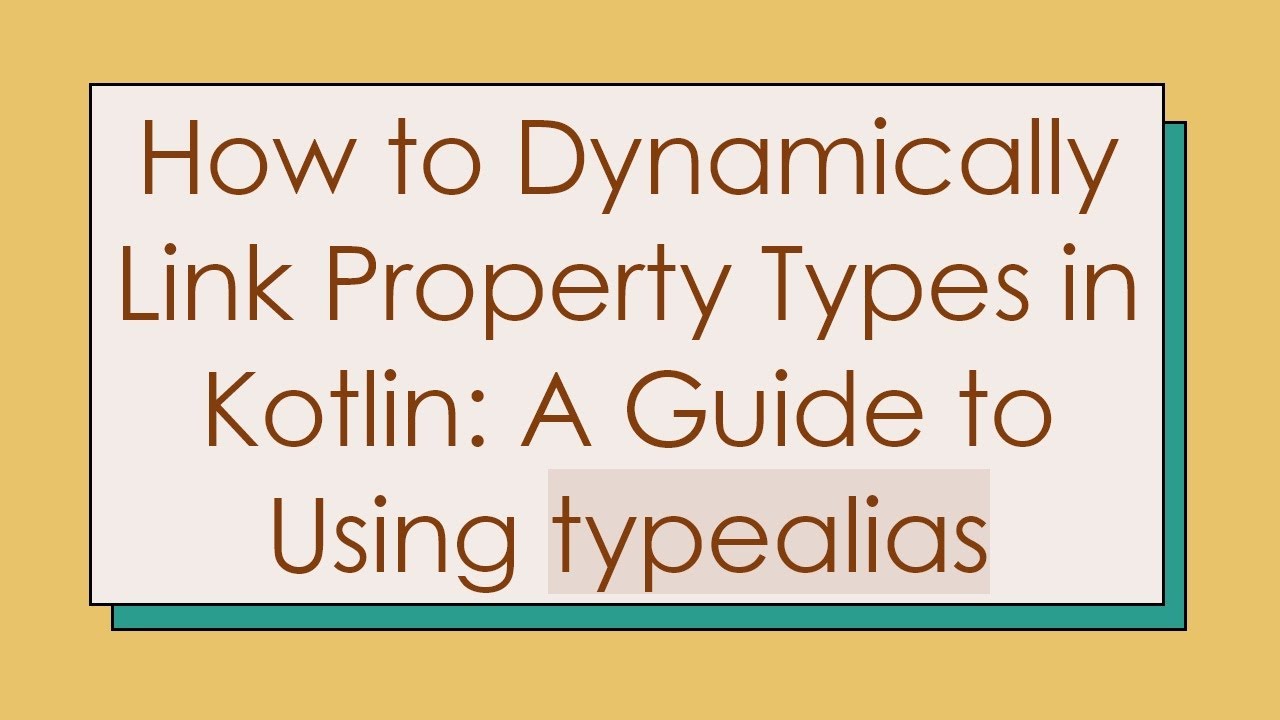
Показать описание
Discover how to use `typealias` in Kotlin to link property types, ensuring scalability and readability in your code.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Set property type from specific class as parameter type
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Dynamically Link Property Types in Kotlin: A Guide to Using typealias
When developing applications in Kotlin, a common challenge arises: how can we efficiently manage property types within our classes? Let's take the example of a simple data class representing a car. You might initially have something like this:
[[See Video to Reveal this Text or Code Snippet]]
You could then create a function like so:
[[See Video to Reveal this Text or Code Snippet]]
However, what happens if your client decides that the id should be an Int instead of a String? This is where the problem sets in, as you would have to change the property type in both the data class and the function declaration, leading to potential inconsistencies and increased workload. This situation is not uncommon and serves as a cue for us to explore a more efficient solution.
The Challenge
The Elegant Solution: Using typealias
Fortunately, Kotlin offers a neat feature called typealias, which allows you to create an alias for an existing type. This provides a clean workaround to the problem of having to change types in multiple places. Here’s how you can incorporate typealias into your code:
Step 1: Define a Type Alias
You can start by defining a type alias for your CarId:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Update Your Data Class
Next, you can modify your Car class to use this new alias instead of the standard String type:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Update Functions to Use the Alias
Finally, when defining your function, you can utilize the alias for its parameter type:
[[See Video to Reveal this Text or Code Snippet]]
Benefits of Using typealias
There are several key benefits to using typealias in this way:
Single Definition: The actual type (String in this case) is now only defined in one place. If you need to change the type to an Int, you only have to do it in your typealias declaration. This reduces the chance of errors and saves time.
Enhanced Readability: By using CarId, you provide semantic clarity. It signifies that this particular String is meant to represent a car ID, making your code easier to read and understand.
Flexibility: While a typealias does not enforce structure (the function will still accept Strings since it's just an alias), it greatly improves the readability of your code.
Conclusion
Using typealias in Kotlin is an effective way to manage property types dynamically without creating unnecessary complications in your code. It allows for better long-term maintainability and makes your development process smoother and more organized. So the next time you find yourself needing to change property types, consider using typealias to streamline the process.
In summary, this approach not only helps in reducing redundancy but also enhances the readability and maintainability of your code, which is essential for any good programming practice. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Set property type from specific class as parameter type
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Dynamically Link Property Types in Kotlin: A Guide to Using typealias
When developing applications in Kotlin, a common challenge arises: how can we efficiently manage property types within our classes? Let's take the example of a simple data class representing a car. You might initially have something like this:
[[See Video to Reveal this Text or Code Snippet]]
You could then create a function like so:
[[See Video to Reveal this Text or Code Snippet]]
However, what happens if your client decides that the id should be an Int instead of a String? This is where the problem sets in, as you would have to change the property type in both the data class and the function declaration, leading to potential inconsistencies and increased workload. This situation is not uncommon and serves as a cue for us to explore a more efficient solution.
The Challenge
The Elegant Solution: Using typealias
Fortunately, Kotlin offers a neat feature called typealias, which allows you to create an alias for an existing type. This provides a clean workaround to the problem of having to change types in multiple places. Here’s how you can incorporate typealias into your code:
Step 1: Define a Type Alias
You can start by defining a type alias for your CarId:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Update Your Data Class
Next, you can modify your Car class to use this new alias instead of the standard String type:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Update Functions to Use the Alias
Finally, when defining your function, you can utilize the alias for its parameter type:
[[See Video to Reveal this Text or Code Snippet]]
Benefits of Using typealias
There are several key benefits to using typealias in this way:
Single Definition: The actual type (String in this case) is now only defined in one place. If you need to change the type to an Int, you only have to do it in your typealias declaration. This reduces the chance of errors and saves time.
Enhanced Readability: By using CarId, you provide semantic clarity. It signifies that this particular String is meant to represent a car ID, making your code easier to read and understand.
Flexibility: While a typealias does not enforce structure (the function will still accept Strings since it's just an alias), it greatly improves the readability of your code.
Conclusion
Using typealias in Kotlin is an effective way to manage property types dynamically without creating unnecessary complications in your code. It allows for better long-term maintainability and makes your development process smoother and more organized. So the next time you find yourself needing to change property types, consider using typealias to streamline the process.
In summary, this approach not only helps in reducing redundancy but also enhances the readability and maintainability of your code, which is essential for any good programming practice. Happy coding!